Managing Prompts and Text Enhance Actions Using the N/llm Module
The content in this help topic pertains to SuiteScript 2.1.
You can use the N/llm
module in your scripts to send prompts to a large language model (LLM) and receive a response. The module supports two approaches:
-
Use llm.generateText(options) or llm.generateText.promise(options) to send text to the LLM and receive a response. When you call these methods, you can specify the model family and model parameters that the LLM should use to provide the response.
-
Use llm.evaluatePrompt(options) or llm.evaluatePrompt.promise(options) to send a prompt that already exists in NetSuite to the LLM and receive a response. When you call these methods, you can specify the ID of an existing prompt and values for any variables that prompt uses.
You can use Prompt Studio to manage prompts and Text Enhance actions in the NetSuite UI, including creating, updating, and deleting them. For more information about Prompt Studio, see Prompt Studio.
The N/llm
module works alongside Prompt Studio and lets you work with prompts and Text Enhance actions in your scripts. You can do the following:
Create, Update, and Delete Prompts and Text Enhance Actions
You can use the N/llm
module and the N/record
module together to manage prompts and Text Enhance actions. For a complete code sample, see Create a Prompt and Evaluate It.
To create a prompt or Text Enhance action, use record.create(options) and specify prompt
as the record type to create a prompt record, or specify textenhanceaction
as the record type to create a Text Enhance action record.
const newPrompt = record.create({
type: "prompt"
});
const newTEAction = record.create({
type: "textenhanceaction"
});
You do not need to specify a script ID for new prompts or Text Enhance actions before saving them. If you do not specify a script ID, a script ID is generated automatically for the saved prompt or Text Enhance action when it is saved, and you can view this script ID in Prompt Studio.
You can load an existing prompt or Text Enhance action record using record.load(options). This method accepts the type of record to load (prompt
or textenhanceaction
, as appropriate) and the internal ID (as a number) of the record. You can find the ID of a prompt or Text Enhance action that you want to load in Prompt Studio.
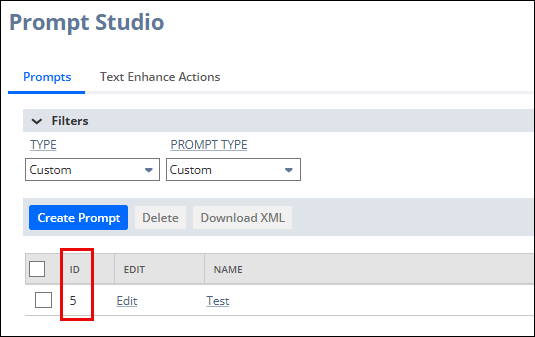
const loadedPrompt = record.load({
type: "prompt",
id: 5
});
const loadedTEAction = record.load({
type: "textenhanceaction",
id: 6
});
After you create or load a prompt or Text Enhance action record, you can use record.Record object methods to work with the record. For example, you can use Record.setValue(options) to set the values of fields on the record. You must populate all required fields on a record before you can save it. For prompt records, the following fields are required:
-
name
-
prompttype
-
modelfamily
-
template
For Text Enhance action records, the following fields are required:
-
name
-
language
You can confirm which fields are required for prompt and Text Enhance action records in Prompt Studio when creating or viewing a prompt or Text Enhance action. Required fields are marked with an asterisk (*), and you can click the field name to see the field ID to use in your scripts.
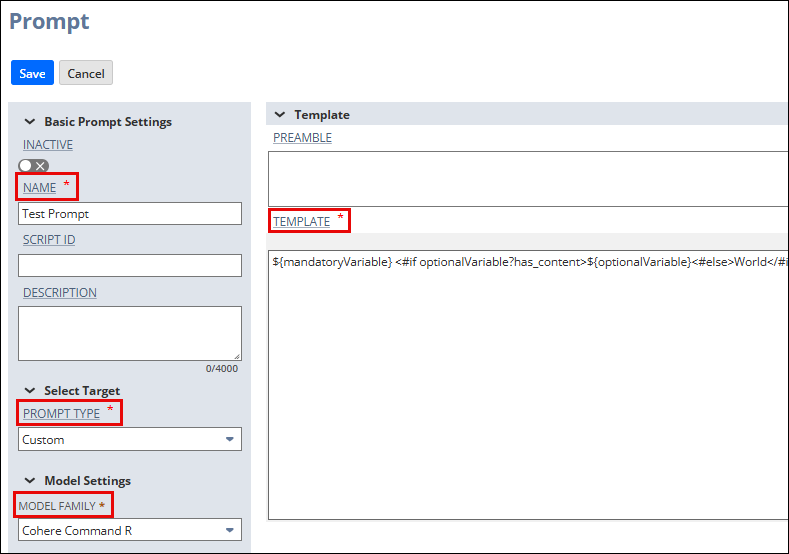
// The newPrompt record object was created in a preceding sample
newPrompt.setValue({
fieldId: "name",
value: "Test Prompt"
});
newPrompt.setValue({
fieldId: "prompttype",
value: "CUSTOM"
});
newPrompt.setValue({
fieldId: "modelfamily",
value: "COHERE_COMMAND_R"
});
newPrompt.setValue({
fieldId: "template",
value: "${mandatoryVariable} <#if optionalVariable?has_content>${optionalVariable}<#else>World</#if>"
});
// The newTEAction record object was created in a preceding sample
newTEAction.setValue({
fieldId: "name",
value: "Test Text Enhance Action"
});
newTEAction.setValue({
fieldId: "language",
value: "en-us"
});
To save the prompt or Text Enhance action record, use Record.save(options). To delete the prompt or Text Enhance action record, use record.delete(options).
// The newPrompt record object was created in a preceding sample
newPrompt.save();
// The newTEAction record object was created in a preceding sample
newTEAction.save();
record.delete({
type: "prompt",
id: 5
});
record.delete({
type: "textenhanceaction",
id: 6
});
Generate SuiteScript Code from an Existing Prompt
When viewing a prompt in Prompt Studio, you can generate a SuiteScript code sample that demonstrates how to work with the prompt. You can use the generated code sample directly in the SuiteScript 2.1 debugger. For more information, see Debugging SuiteScript 2.1 Scripts. You can also modify the sample and use it in your scripts.
To generate a code sample from a prompt, when viewing the prompt in Prompt Studio, click Show SuiteScript Example.

The generated code sample calls llm.evaluatePrompt(options) and specifies the prompt information as parameters. The generated code sample includes any required or optional variables that are used in the prompt's template. If you specified values for the template variables in Prompt Studio, these values are populated in the generated code sample. You can also provide your own values when you run the sample, which can be useful for testing purposes.
