CustomLine
Type |
Object |
Description |
Contains all properties for a single custom line for the GL impact on a transaction. Use the methods available to the CustomLine object to set the values for the custom line and define plug-in implementation functionality based on the values. The CustomLines object contains a reference to each custom GL impact line. Create a new CustomLine object with addNewLine(). |
Methods |
Note:
The StandardLine and CustomLine objects share some common methods. For more information, see Common StandardLine and CustomLine Object Methods. |
Parent Object(s) |
|
Child Object(s) |
n/a |
isBookSpecific()
Function Declaration |
|
Type |
Object method |
Description |
Returns
Note:
This method only applies if you use the Multi-Book Accounting feature and the method is being called for the primary accounting book. For more information about how the plug-in implementation processes book-specific lines, see Custom GL Lines Plug-in Process Flow. |
Returns |
boolean |
Input Parameters |
None. |
Parent object |
Example
function customizeGlImpact(transactionRecord, standardLines, customLines, book)
{
...
if(book.isPrimary())
{
// set all custom lines to apply to all books
for (var i = 0; i < customLines.getCount(); i++)
{
var currLine = customLines.getLine(i);
if (currline.isBookSpecific())
{
currLine.setBookSpecific(false);
...
}
}
}
...
}
setBookSpecific(bookSpecific)
Function Declaration |
|
Type |
Object method |
Description |
Sets a custom GL impact line to affect only the primary book in a Custom GL plug-in implementation. If you use this method and set the value to If you do not use this method or set the value to |
Returns |
void |
Input Parameters |
bookSpecific {boolean} — Use |
Parent object |
Example
function customizeGlImpact(transactionRecord, standardLines, customLines, book)
{
...
if (book.isPrimary())
{
// set all custom lines to be specific to the primary book
for (var i = 0; i < customLines.getCount(); i++)
{
var currLine = customLines.getLine(i);
if (!currline.isBookSpecific())
{
currLine.setBookSpecific(true);
...
}
}
}
...
}
setAccountId(accountId)
Function Declaration |
|
Type |
Object method |
Description |
Sets the account ID property for a CustomLine object in a primary or secondary book. This value is the internal NetSuite ID for a general ledger account. You must use this method to set the account to be debited or credited for a custom line. You can view the internal NetSuite ID for all accounts on the Chart of Accounts page at Setup > Accounting > Chart of Accounts. You can use helper functions in a utility file to customize the internal NetSuite account IDs in the plug-in implementation logic. See Utility Files for a Custom GL Lines Plug-In Implementation. |
Returns |
void |
Input Parameters |
accountId {int} — Internal NetSuite ID for an account. |
Parent object |
Example
function customizeGlImpact(transactionRecord, standardLines, customLines, book)
{
...
var newLine = customLines.addNewLine();
newLine.setCreditAmount(standardLines.getLine(0).getCreditAmount());
newLine.setAccountId(standardLines.getLine(0).getAccountId());
newLine.setMemo("Payment catches both revenue and cash.");
...
var newLine = customLines.addNewLine();
newLine.setDebitAmount(standardLines.getLine(1).getDebitAmount());
newLine.setAccountId(standardLines.getLine(1).getAccountId());
newLine.setMemo("Payment catches both revenue and cash.");
...
}
setClassId(classId)
Function Declaration |
|
Type |
Object method |
Description |
Sets the class ID property for a CustomLine object in a primary or secondary book. This value is the internal NetSuite ID for a class. Classes are categories that you can create to track records such as financials, transactions, and employees. You can view all classes defined in NetSuite at Setup > Company > Classes. See also getClassId(). You can use helper functions in a utility file to avoid adding the internal NetSuite IDs into the plug-in implementation logic. See Utility Files for a Custom GL Lines Plug-In Implementation. |
Returns |
void |
Input Parameters |
classId {int} — Internal NetSuite ID for a class. |
Parent object |
Example
function customizeGlImpact(transactionRecord, standardLines, customLines, book)
{
var context = nlapiGetContext();
var makeClassesMandatory = context.getPreference('classmandatory');
var allowPerLineClasses = context.getPreference('classesperline');
...
var newLine = customLines.addNewLine();
...
if (makeClassesMandatory || allowPerLineClasses)
{
var classId = getClassForItem(itemId);
newLine.setClassId(classId);
}
...
}
// Utility methods
function getClassForItem(itemId)
{
...
return classId;
}
setCreditAmount(credit)
Function Declaration |
|
Type |
Object method |
Description |
Sets the credit amount of a CustomLine object in a primary or secondary book. The value is rounded to currency precision. To set a debit amount, use setDebitAmount(debit).
Note:
The currency for the amount of the custom line depends on the subsidiary and accounting book to which the GL impact posts. For example, if the accounting book being processed by the plug-in implementation uses USD, the currency for the amount set by this method is USD. For more information, see Multiple Currencies Examples. |
Returns |
void |
Input Parameters |
credit {string} — String value of a credit on a general ledger account. Requires a positive value. |
Parent object |
Example
function customizeGlImpact(transactionRecord, standardLines, customLines, book)
{
...
var newLine = customLines.addNewLine();
newLine.setCreditAmount(standardLines.getLine(0).getCreditAmount());
newLine.setAccountId(standardLines.getLine(0).getAccountId());
newLine.setMemo("Payment catches both revenue and cash.");
...
var newLine = customLines.addNewLine();
newLine.setDebitAmount(standardLines.getLine(1).getDebitAmount());
newLine.setAccountId(standardLines.getLine(1).getAccountId());
newLine.setMemo("Payment catches both revenue and cash.");
...
}
setDebitAmount(debit)
Function Declaration |
|
Type |
Object method |
Description |
Sets the debit amount of a CustomLine object in a primary or secondary book. The value is rounded to currency precision. To set a credit amount, use setCreditAmount(credit).
Note:
The currency for the amount on the custom line depends on the accounting book to which the GL impact posts. For example, if the accounting book being processed by the plug-in implementation uses USD, the currency for the amount set by this method is USD. For more information, see Multiple Currencies Examples. |
Returns |
void |
Input Parameters |
debit {string} — String value of a debit on a general ledger account. Requires a positive value. |
Parent object |
Example
function customizeGlImpact(transactionRecord, standardLines, customLines, book)
{
...
var newLine = customLines.addNewLine();
newLine.setCreditAmount(standardLines.getLine(0).getCreditAmount());
newLine.setAccountId(standardLines.getLine(0).getAccountId());
newLine.setMemo("Payment catches both revenue and cash.");
...
var newLine = customLines.addNewLine();
newLine.setDebitAmount(standardLines.getLine(1).getDebitAmount());
newLine.setAccountId(standardLines.getLine(1).getAccountId());
newLine.setMemo("Payment catches both revenue and cash.");
...
}
setDepartmentId(departmentId)
Function Declaration |
|
Type |
Object method |
Description |
Sets the department ID for a CustomLine object in a primary or secondary book. This value is the internal NetSuite ID for a department. Departments are listed first on transactions, and are useful when designating transactions and employees as part of an internal team. You can view all departments defined in NetSuite at Setup > Company > Departments. See also getDepartmentId(). You can use helper functions in a utility file to avoid adding the internal NetSuite IDs into the plug-in implementation logic. You can also access the |
Returns |
void |
Input Parameters |
departmentId {int} — Internal NetSuite ID for a department. |
Parent object |
Example
function customizeGlImpact(transactionRecord, standardLines, customLines, book)
{
var context = nlapiGetContext();
var makeDepartmentsMandatory = context.getPreference('deptmandatory');
var allowPerLineDepartments = context.getPreference('deptsperline');
...
var newLine = customLines.addNewLine();
...
if (makeDepartmentsMandatory || allowPerLineDepartments)
{
var departmentId = getDepartmentForItem(itemId);
newLine.setDepartmentId(departmentId);
}
...
}
// Utility methods
function getDepartmentForItem(itemId)
{
...
return departmentId;
}
setEntityId(entityId)
Function Declaration |
|
Type |
Object method |
Description |
Sets the entity ID property for a CustomLine object in a primary or secondary book to the internal NetSuite ID Supported entity types include:
The following restrictions apply to setting of entities on custom GL lines:
Note:
This method does not set the sales representative for the line, and it does not change the date of the first sale for the customer. |
Returns |
void |
Input Parameters |
entityId {int} — internal NetSuite ID for an entity |
Parent object |
Example
function customizeGlImpact(record, standardLines, customLines, book)
{
var line = customLines.addNewLine()
line.setAccountId(6)
line.setDebitAmount(100)
line.setEntityId(99)
line = customLines.addNewLine()
line.setAccountId(7)
line.setCreditAmount(100)
}
Reports that include GL information and are grouped by entity include custom GL information, if the entity is supported by the new method.
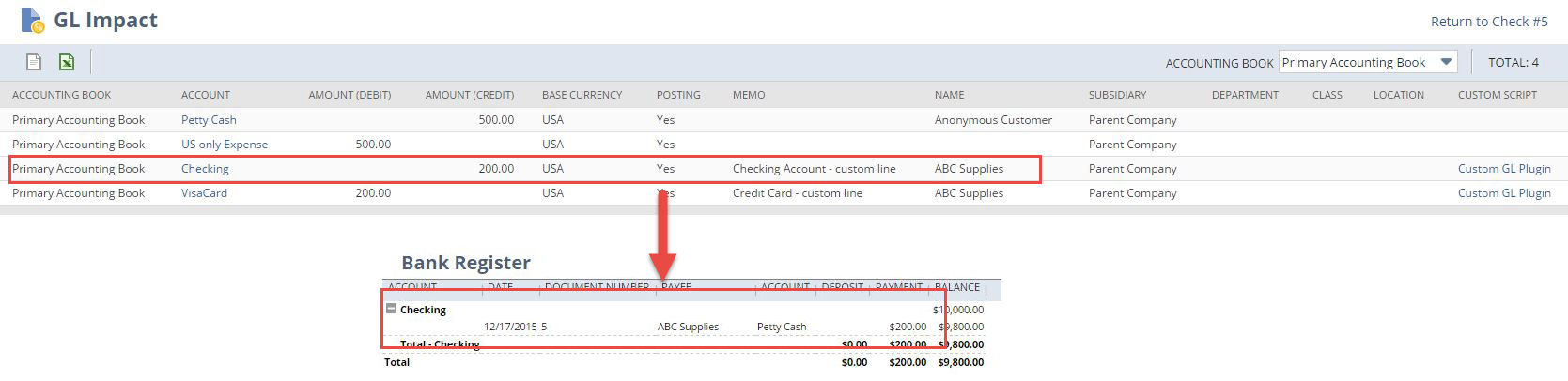
setLocationId(locationId)
Function Declaration |
|
Type |
Object method |
Description |
Sets the location ID for a CustomLine object in a primary or secondary book. This value is the internal NetSuite ID for a location. Use locations to track information about employees and transactions for multiple offices or warehouses. You can view all locations defined in NetSuite at Setup > Company > Locations. See also getLocationId(). You can use helper functions in a utility file to avoid adding the internal NetSuite IDs into the plug-in implementation logic. You can also access the |
Returns |
void |
Input Parameters |
locationId {int} — Internal NetSuite ID for a location. |
Parent object |
Example
function customizeGlImpact(transactionRecord, standardLines, customLines, book)
{
var context = nlapiGetContext();
var makeLocationsMandatory = context.getPreference('locmandatory');
var allowPerLineLocations = context.getPreference('locsperline');
...
var newLine = customLines.addNewLine();
...
if (makeLocationsMandatory || allowPerLineLocations)
{
var locationId = getLocationForItem(itemId);
newLine.setLocationId(locationId);
}
...
}
// Utility methods
function getLocationForItem(itemId)
{
...
return locationId;
}
setMemo(memo)
Function Declaration |
|
Type |
Object method |
Description |
Sets the Memo field on a CustomLine object. See also getMemo(). |
Returns |
void |
Input Parameters |
memo {string} — String text for the Memo field. |
Parent object |
Example
function customizeGlImpact(transactionRecord, standardLines, customLines, book)
{
...
var newLine = customLines.addNewLine();
newLine.setCreditAmount(standardLines.getLine(0).getCreditAmount());
newLine.setAccountId(standardLines.getLine(0).getAccountId());
newLine.setMemo("Payment catches both revenue and cash.");
...
var newLine = customLines.addNewLine();
newLine.setDebitAmount(standardLines.getLine(1).getDebitAmount());
newLine.setAccountId(standardLines.getLine(1).getAccountId());
newLine.setMemo("Payment catches both revenue and cash.");
...
}
setSegmentValueId(segmentId, segmentValueId)
Function Declaration |
|
Type |
Object method |
Description |
Sets custom segment values on a CustomLine object NetSuite assigns custom segment values as follows:
|
Returns |
void |
Input Parameters |
segmentId {string} — String value of the custom segment ID segmentValue {integer} — Integer value that the custom line should be set to |
Parent object |
Example
function customizeGlImpact(transactionRecord, standardLines, customLines, book)
{
...
var newLine = customLines.addNewLine();
...
// custom function for getting value for cost center segment
newLine.setSegmentValueId('csegcostcenter', getCostCenterValueForItem(itemId));
// using constant for region segment
newLine.setSegmentValueId('csegregion', 3);
// copying segment value from line 0 for work center segment
var workcenter = 'csegworkcenter'
newLine.setSegmentValueId(workcenter, standardLines.getLine(0).getSegmentValueId(workcenter));
...
}
// Utility methods
function getCostCenterValueForItem(itemId)
{
...
return valueId;
}