Demand Plan Detail Sublist
The internal ID for this sublist is demandplandetail.
The Demand Plan Detail sublist appears on the Item Demand Plan record type.
The Demand Plan Detail sublist is a matrix that is similar to the Pricing sublist. This matrix stores projected quantities demanded by date. Each row in the matrix represents a specific month, week, or day, and each column in the matrix represents an expected quantity demand.
For help working with this record in the UI, see Demand Planning.
Functionally, this sublist shares many of the characteristics of List Sublists. However, scripting with the Demand Plan Detail sublist is not like scripting with most other sublists in NetSuite. You must use Matrix APIs for the Demand Plan Detail Sublist to access quantity values on a per-row, per-column basis, similar to the way that item pricing values are accessed. These APIs are a subset of the N/currentRecord Module and N/record Module APIs more commonly used for scripting with other sublists.
The format of the Demand Plan Detail sublist depends on the values set in body fields for the start date of the plan, the end date of the plan, and the time period to be used (monthly, weekly, or daily). Because of this dependence, you should work with the Item Demand Plan record and the Demand Plan Detail sublist in dynamic mode. See SuiteScript 2.x Standard and Dynamic Modes
Be aware of the following requirements:
-
To script with the Item Demand Plan record and the Demand Plan Detail sublist for inventory items, the Demand Planning feature must be enabled. For assembly/BOM items, the Work Orders feature also must be enabled.
-
Demand plans are supported only for item(s) that have the supplyreplenishmentmethod field set to Time Phased.
-
Required body field values must be defined before matrix field values can be edited. In dynamic mode, current values may be retrieved. Start date and end date body fields default to the first day and last day of the current year.
For more details and code samples for each type of demand plan, see the following help topics:
Monthly Demand Plan
A monthly demand plan includes a row for each month within the body field start date and end date, and one quantity column for each month.
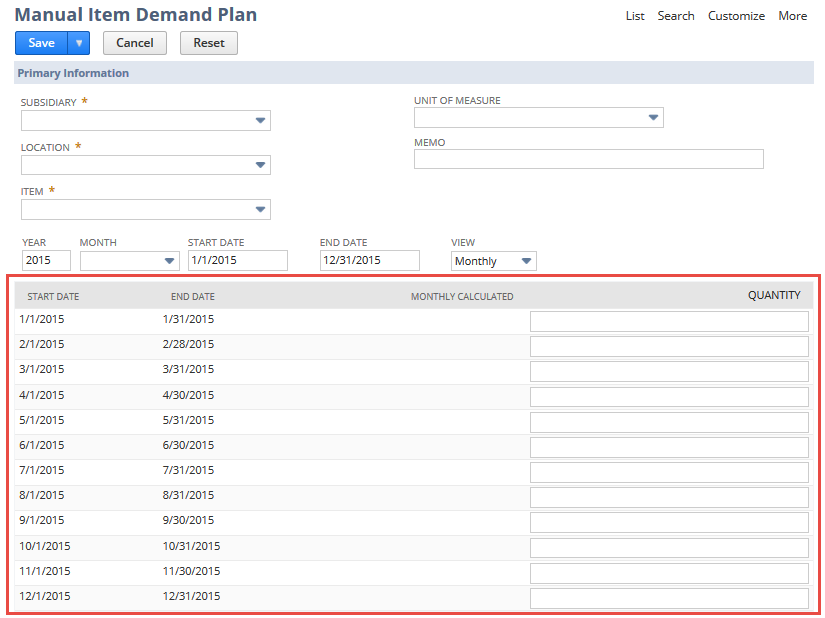
-
The sublist startdate and enddate fields are system-calculated and read-only.
-
The startdate is the date of the first day of the month represented by each row.
-
The enddate is the date of the last day of the month represented by each row.
-
The month for row 1 is the month set in the body field start date, the month for row 2 is the next month, and so on, until the month set in the body field end date is reached.
-
-
The values for the quantity field can be set in SuiteScript. For monthly demand plans, the column parameter for this field is always 1.
Monthly Demand Plan Code Sample
The following sample sets quantities for the months of January and February, 2011:
/**
* @NApiVersion 2.1
*/
require(['N/record', 'N/log'], (record, log) => {
const demandplan = record.create({
type: record.Type.ITEM_DEMAND_PLAN,
isDynamic: true
})
demandplan.setValue({
fieldId: 'demandplancalendartype',
value: 'MONTHLY'
})
demandplan.setValue({
fieldId: 'subsidiary',
value: 1
})
demandplan.setValue({
fieldId: 'location',
value: 1
})
demandplan.setValue({
fieldId: 'item',
value: 253
})
const id = demandplan.save({
enableSourcing: true
})
const loadDemandPlan = record.load({
type: record.Type.ITEM_DEMAND_PLAN,
id: id,
isDynamic: true
})
loadDemandPlan.setValue({
fieldId: 'startdate',
value: '1/1/2023'
})
loadDemandPlan.setValue({
fieldId: 'enddate',
value: '12/31/2023'
})
loadDemandPlan.selectLine({
sublistId: 'demandplandetail',
line: '0'
})
loadDemandPlan.setCurrentSublistValue({
sublistId: 'demandplandetail',
fieldId: 'quantity_1_',
value: 100
})
loadDemandPlan.selectLine({
sublistId: 'demandplandetail',
line: '1'
})
loadDemandPlan.setCurrentSublistValue({
sublistId: 'demandplandetail',
fieldId: 'quantity_1_',
value: 200
})
loadDemandPlan.commitLine({
sublistId: 'demandplandetail'
})
loadDemandPlan.save()
})
Weekly Demand Plan
A weekly demand plan includes a row for each week contained in the time period set by the body field start date and end date, and one quantity column for each week.
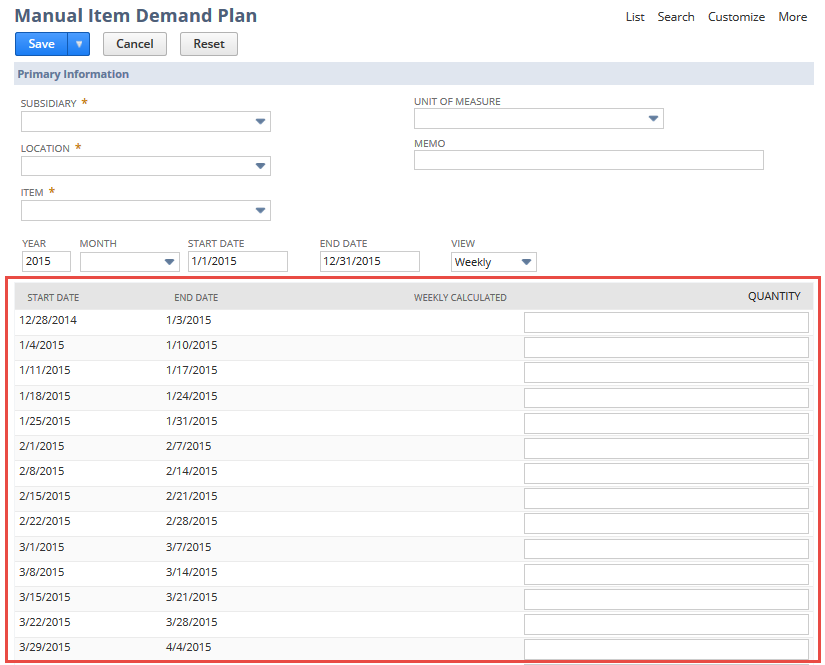
-
The sublist startdate and enddate fields are system-calculated and read-only.
-
The startdate is the date of the first day of the week represented by each row.
-
The enddate is the date of the last day of the week represented by each row.
Note:The first day of the week by default is Sunday, but may vary according to the company preference set for First Day of the Week at Setup > Company > General Preferences.
-
The week for row 1 is the week of the date set in the body field start date. Note that unless the body field start date happens to be the first day of the week, the startdate for this first row may precede the body field start date.
-
The week for the final sublist row is the week of the date set in the body field end date. Note that unless the body field end date happens to be the last day of the week, the enddate for this last row may be after the body field enddate.
-
-
The values for the quantity field can be set in SuiteScript. For weekly demand plans, the column parameter for this field is always 1.
Weekly Demand Plan Code Sample
The following sample sets quantities for the first two weeks of 2011:
/**
* @NApiVersion 2.1
*/
require(['N/record', 'N/log'], (record, log) => {
const demandplan = record.create({
type: record.Type.ITEM_DEMAND_PLAN,
isDynamic: true
})
demandplan.setValue({
fieldId: 'demandplancalendartype',
value: 'WEEKLY'
})
demandplan.setValue({
fieldId: 'subsidiary',
value: 1
})
demandplan.setValue({
fieldId: 'location',
value: 1
})
demandplan.setValue({
fieldId: 'item',
value: 252
})
const id = demandplan.save({
enableSourcing: true
})
const loadDemandPlan = record.load({
type: record.Type.ITEM_DEMAND_PLAN,
id: id,
isDynamic: true
})
loadDemandPlan.setValue({
fieldId: 'startdate',
value: '1/1/2023'
})
loadDemandPlan.setValue({
fieldId: 'enddate',
value: '12/31/2023'
})
loadDemandPlan.selectLine({
sublistId: 'demandplandetail',
line: '0'
})
loadDemandPlan.setCurrentSublistValue({
sublistId: 'demandplandetail',
fieldId: 'quantity_1_',
value: 100
})
loadDemandPlan.selectLine({
sublistId: 'demandplandetail',
line: '1'
})
loadDemandPlan.setCurrentSublistValue({
sublistId: 'demandplandetail',
fieldId: 'quantity_1_',
value: 200
})
loadDemandPlan.commitLine({
sublistId: 'demandplandetail'
})
loadDemandPlan.save()
})
Daily Demand Plan
A daily demand plan includes a row for each week contained in the time period set by the body field start date and end date, and seven quantity columns for each week, one for each day of the week.
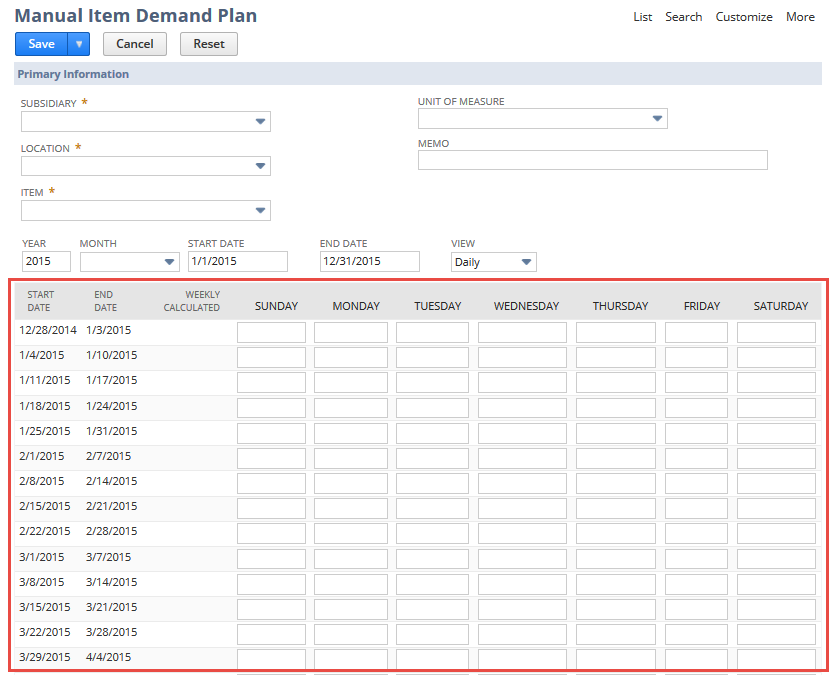
-
The sublist startdate and enddate fields are system-calculated and read-only.
-
The startdate is the date of the first day of the week represented by each row.
-
The enddate is the date of the last day of the week represented by each row.
Note:The first day of the week by default is Sunday, but may vary according to the company preference set for First Day of the Week at Setup > Company > General Preferences.
-
The week for row 1 is the week of the date set in the body field start date. Note that unless the body field start date happens to be the first day of the week, the startdate for this first row may precede the body field start date.
-
The week for the final sublist row is the week of the date set in the body field end date. Note that unless the body field end date happens to be the last day of the week, the enddate for this last row may be after the body field enddate.
-
-
The values for the quantity fields can be set in SuiteScript.
-
The column parameter for a quantity field is 1,2,3,4,5,6, or 7, depending on the day of the week.
-
In the screenshot above, the week starts with Sunday, which is the default first day of the week, and in this case, maps to a column parameter of 1. However, 1 does not always map to Sunday; it maps to the first day of the week as set in the company preferences.
-
Daily Demand Plan Code Sample
/**
* @NApiVersion 2.1
*/
require(['N/record', 'N/log'], (record, log) => {
const demandplan = record.create({
type: record.Type.ITEM_DEMAND_PLAN,
isDynamic: true
})
demandplan.setValue({
fieldId: 'demandplancalendartype',
value: 'DAILY'
})
demandplan.setValue({
fieldId: 'subsidiary',
value: 1
})
demandplan.setValue({
fieldId: 'location',
value: 1
})
demandplan.setValue({
fieldId: 'item',
value: 254
})
const id = demandplan.save({
enableSourcing: true
})
const loadDemandPlan = record.load({
type: record.Type.ITEM_DEMAND_PLAN,
id: id,
isDynamic: true
})
loadDemandPlan.setValue({
fieldId: 'startdate',
value: '1/1/2023'
})
loadDemandPlan.setValue({
fieldId: 'enddate',
value: '12/31/2023'
})
loadDemandPlan.selectLine({
sublistId: 'demandplandetail',
line: '0'
})
loadDemandPlan.setCurrentSublistValue({
sublistId: 'demandplandetail',
fieldId: 'quantity_1_',
value: 100
})
loadDemandPlan.selectLine({
sublistId: 'demandplandetail',
line: '1'
})
loadDemandPlan.setCurrentSublistValue({
sublistId: 'demandplandetail',
fieldId: 'quantity_1_',
value: 200
})
loadDemandPlan.commitLine({
sublistId: 'demandplandetail'
})
loadDemandPlan.save()
})
Matrix APIs for the Demand Plan Detail Sublist
Use the following matrix APIs with the Demand Plan Detail sublist:
With all APIs listed above, use the Record.selectLine(options) or CurrentRecord.selectLine(options) APIs first to select an existing line.
For more information about APIs, see N/currentRecord Module and N/record Module.