N/ui/dialog Module Script Samples
The following script samples demonstrate how to use the features of the N/ui/dialog module:
Create an Alert Dialog
The following sample shows how to create an alert dialog.
This script sample uses the define
function, which is required for an entry point script (a script you attach to a script record and deploy). You must use the require
function if you want to copy the script into the SuiteScript Debugger and test it. For more information, see SuiteScript 2.x Global Objects.
To debug client scripts like the following, you should use Chrome DevTools for Chrome, Firebug debugger for Firefox, or Microsoft Script Debugger for Internet Explorer. For information about these tools, see the documentation provided with each browser. For more information about debugging SuiteScript client scripts, see Debugging Client Scripts.
This sample uses SuiteScript 2.1. For more information, see SuiteScript 2.1.
/**
* @NApiVersion 2.1
* @NScriptType ClientScript
*/
define(['N/ui/dialog'], (dialog) => {
function pageInit() {
let options = {
title: 'I am an Alert',
message: 'Click OK to continue.'
};
function success(result) {
console.log('Success with value ' + result);
}
function failure(reason) {
console.log('Failure: ' + reason);
}
dialog.alert(options).then(success).catch(failure);
}
return {
pageInit: pageInit
};
});
The following screenshot shows the Alert dialog created in this example:
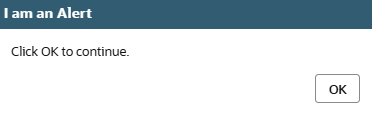
Create a Confirmation Dialog
The following sample shows how to create a confirmation dialog.
This script sample uses the define
function, which is required for an entry point script (a script you attach to a script record and deploy). You must use the require
function if you want to copy the script into the SuiteScript Debugger and test it. For more information, see SuiteScript 2.x Global Objects.
To debug client scripts like the following, you should use Chrome DevTools for Chrome, Firebug debugger for Firefox, or Microsoft Script Debugger for Internet Explorer. For information about these tools, see the documentation provided with each browser. For more information about debugging SuiteScript client scripts, see Debugging Client Scripts.
This sample uses SuiteScript 2.1. For more information, see SuiteScript 2.1.
/**
* @NApiVersion 2.1
* @NScriptType ClientScript
*/
define(['N/ui/dialog'], (dialog) => {
function pageInit() {
let options = {
title: 'I am a Confirmation',
message: 'Press OK or Cancel'
};
function success(result) {
console.log('Success with value ' + result);
}
function failure(reason) {
console.log('Failure: ' + reason);
}
dialog.confirm(options).then(success).catch(failure);
}
return {
pageInit: pageInit
};
});
The following screenshot shows the Confirmation dialog created in this example:
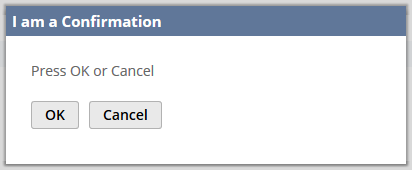
Create a Dialog with Buttons
The following sample shows how to create a dialog with three buttons.
This script sample uses the define
function, which is required for an entry point script (a script you attach to a script record and deploy). You must use the require
function if you want to copy the script into the SuiteScript Debugger and test it. For more information, see SuiteScript 2.x Global Objects.
To debug client scripts like the following, you should use Chrome DevTools for Chrome, Firebug debugger for Firefox, or Microsoft Script Debugger for Internet Explorer. For information about these tools, see the documentation provided with each browser. For more information about debugging SuiteScript client scripts, see Debugging Client Scripts.
This sample uses SuiteScript 2.1. For more information, see SuiteScript 2.1.
/**
* @NApiVersion 2.1
* @NScriptType ClientScript
*/
define(['N/ui/dialog'], (dialog) => {
function pageInit() {
let button1 = {
label: 'I am A',
value: 1
};
let button2 = {
label: 'I am B',
value: 2
};
let button3 = {
label: 'I am C',
value: 3
};
let options = {
title: 'Alphabet Test',
message: 'Which One?',
buttons: [button1, button2, button3]
};
function success(result) {
console.log('Success with value ' + result);
}
function failure(reason) {
console.log('Failure: ' + reason);
}
dialog.create(options).then(success).catch(failure);
}
return {
pageInit: pageInit
};
});
The following screenshot shows the dialog with buttons created in this example:
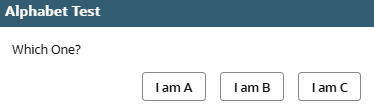
Create a Dialog that Includes a Default Button
The following sample shows how to create a dialog without specifying any buttons. The default behavior is to create a dialog that includes a single button with the label OK.
This script sample uses the define
function, which is required for an entry point script (a script you attach to a script record and deploy). You must use the require
function if you want to copy the script into the SuiteScript Debugger and test it. For more information, see SuiteScript 2.x Global Objects.
To debug client scripts like the following, you should use Chrome DevTools for Chrome, Firebug debugger for Firefox, or Microsoft Script Debugger for Internet Explorer. For information about these tools, see the documentation provided with each browser. For more information about debugging SuiteScript client scripts, see Debugging Client Scripts.
This sample uses SuiteScript 2.1. For more information, see SuiteScript 2.1.
/**
* @NApiVersion 2.1
* @NScriptType ClientScript
*/
define(['N/ui/dialog'], (dialog) => {
function pageInit() {
let options = {
title: 'I am a Dialog with the default button',
message: 'Click a button to continue.',
};
function success(result) {
console.log('Success with value ' + result);
}
function failure(reason) {
console.log('Failure: ' + reason);
}
dialog.create(options).then(success).catch(failure);
}
return {
pageInit: pageInit
};
});
The following screenshot shows the dialog with the default button created in this example:
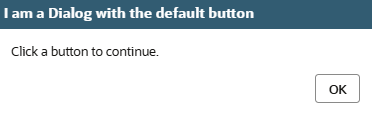