4 Instrumenting and Registering Custom MBeans
This chapter includes the following sections:
- Overview of the MBean Development Process
This section describes the MBean development process. - Create and Implement a Management Interface
One of the main advantages to the standard MBeans design pattern is that you define and implement management properties (attributes) as you would any Java property (usinggetxxx
,setxxx
, andisxxx
methods); similarly, you define and implement management methods (operations) as you would any Java method. - Modify Business Methods to Push Data
If your management attributes contain data about the number of times a business method has been invoked, or if you want management attributes to contain the same value as a business property, modify your business methods to push (update) data into the management implementation class. - Register the MBean
If you want to instantiate your MBeans as part of application deployment, create anApplicationLifecycleListener
that registers your MBean when the application deploys. - Package Application and MBean Classes
Package your MBean classes in the application'sAPP-INF
directory or in a module's JAR, WAR or other type of archive file depending on the access that you want to enable for the MBean.
Overview of the MBean Development Process
This section describes the MBean development process.
Figure 4-1 illustrates the MBean development process. The steps in the process, and the results of each are described in Table 4-1. Subsequent sections detail each step in the process.
Figure 4-1 Standard MBean Development Overview
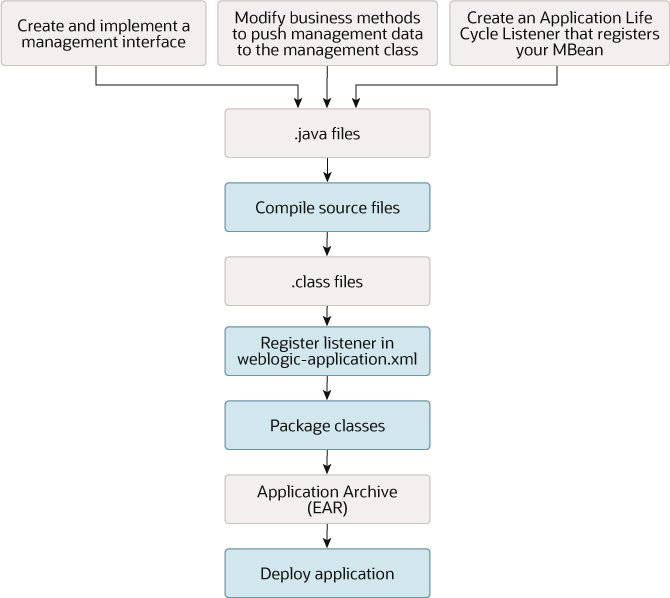
Description of "Figure 4-1 Standard MBean Development Overview"
Table 4-1 Model MBean Development Tasks and Results
Step | Description | Result |
---|---|---|
Create a standard Java interface that describes the properties (management attributes) and operations you want to expose to JMX clients. Create a Java class that implements the interface. Because management logic should be separate from business logic, the implementation should not be in the same class that contains your business methods. |
Source files that describe and implement your management interface. |
|
If your management attributes contain data about the number of times a business method has been invoked, or if you want management attributes to contain the same value as a business property, modify your business methods to push (update) data into the management implementation class. For example, if you want to keep track of how frequently your business class writes to the database, modify the business method that is responsible for writing to the database to also increment a counter property in your management implementation class. This design pattern enables you to insert a minimal amount of management code in your business code. |
A clean separation between business logic and management logic. |
|
If you want to instantiate your MBeans as part of application deployment, create a WebLogic Server |
A Java class and added entries in |
|
Package your compiled classes into a single archive. |
A JAR, WAR, EAR file or other deployable archive file. |
Parent topic: Instrumenting and Registering Custom MBeans
Create and Implement a Management Interface
One of the main advantages to the standard MBeans design pattern is that you define
and implement management properties (attributes) as you would any Java property (using
getxxx
, setxxx
, and isxxx
methods);
similarly, you define and implement management methods (operations) as you would any Java
method.
When you register the MBean, the MBean server examines the MBean interface and
determines how to represent the data to JMX clients. Then, JMX clients use the
MBeanServerConnection.getAttribute()
and
setAttribute()
methods to get and set the values of attributes in
your MBean and they use MBeanServerConnection.invoke()
to invoke its
operations. See MBeanServerConnection
in the Java SE 17 API
Specification at https://docs.oracle.com/en/java/javase/17/docs/api/java.management/javax/management/MBeanServerConnection.html
.
To create an interface for your standard MBean:
-
Declare the interface as public.
-
Oracle recommends that you name the interface as follows:
Business-objectMBean.java
where
Business-object
is the object that is being managed.Oracle's recommended design pattern for standard MBeans enables you to follow whatever naming convention you prefer. In other standard MBean design patterns (patterns in which the MBean's implementation file does not extend
javax.management.StandardMBean
), the file name must follow this pattern:Impl-fileMBean.java
whereImpl-file
is the name of the MBean's implementation file. -
For each read-write attribute that you want to make available in your MBean, define a getter and setter method that follows this naming pattern:
getAttribute-name
setAttribute-name
where
Attribute-name
is a case-sensitive name that you want to expose to JMX clients.If your coding conventions prefer that you use an
isAttribute-name
as the getter method for attributes of typeBoolean
, you may do so. However, JMX clients use theMBeanServerConnection.getAttribute()
method to retrieve an attribute's value regardless of the attribute's data type; there is noMBeanServerConnection.isAttribute()
method. -
For each read-only attribute that you want to make available, define only an
is
or agetter
method.For each write-only attribute, define only a setter method.
-
Define each management operation that you want to expose to JMX clients.
Example 4-1 is an MBean interface that defines a read-only attribute of type int
and an operation that JMX clients can use to set the value of the attribute to 0
.
Example 4-1 Management Interface
package com.bea.medrec.controller; public interface RecordSessionEJBMBean { public int getTotalRx(); public void resetTotalRx(); }
To implement the interface:
Example 4-2 MBean Implementation
package com.bea.medrec.controller; import javax.management.StandardMBean; import com.bea.medrec.controller.RecordSessionEJBMBean; public class RecordSessionEJBMBeanImpl extends StandardMBean implements RecordSessionEJBMBean { public RecordSessionEJBMBeanImpl() throws javax.management.NotCompliantMBeanException { super(RecordSessionEJBMBean.class); } public int TotalRx = 0; public int getTotalRx() { return TotalRx; } public void incrementTotalRx() { TotalRx++; } public void resetTotalRx() { TotalRx = 0; } }
Parent topic: Instrumenting and Registering Custom MBeans
Modify Business Methods to Push Data
If your management attributes contain data about the number of times a business method has been invoked, or if you want management attributes to contain the same value as a business property, modify your business methods to push (update) data into the management implementation class.
Example 4-3 shows a method in an EJB that increments the integer in the TotalRx
property each time the method is invoked.
Example 4-3 EJB Method That Increments the Management Attribute
private Collection addRxs(Collection rXs, RecordLocal recordLocal) throws CreateException, Exception { ... com.bea.medrec.controller.RecordSessionEJBMBeanImpl.incrementTotalRx(); ... }
Parent topic: Instrumenting and Registering Custom MBeans
Register the MBean
If you want to instantiate your MBeans as part of application deployment, create an
ApplicationLifecycleListener
that registers your MBean when the
application deploys.
For more information, see Use ApplicationLifecycleListener to Register Application MBeans.
-
Create a class that extends
weblogic.application.ApplicationLifecycleListener
. -
In this
ApplicationLifecycleListener
class, implement theApplicationLifecycleListener.postStart(ApplicationLifecycleEvent evt)
method.In your implementation of this method:
-
Construct an object name for your MBean.
Oracle recommends this naming convention:
your.company:Name=Parent-module,Type=MBean-interface-classname
To get the name of the parent module, use
ApplicationLifecycleEvent
to get anApplicationContext
object. Then useApplicationContext
to get the module's identification. -
If you are registering the MBean on the WebLogic Server Runtime MBean Server:
Access the WebLogic Server Runtime MBean Server through JNDI.
If the classes for the JMX client are part of a Jakarta EE module, such as an EJB or Web application, the JNDI name for the Runtime MBeanServer is:
weblogic/jmx/runtime
For example:
InitialContext ctx = new InitialContext(); MBeanServer server = (MBeanServer) ctx.lookup("weblogic/jmx/runtime");
If the classes for the JMX client are not part of a Jakarta EE module, the JNDI name for the Runtime MBean Server is:
java:comp/jmx/runtime
See Make Local Connections to the Runtime MBean Server in Developing Custom Management Utilities Using JMX for Oracle WebLogic Server.
-
If you are registering the MBean on the Domain Runtime MBean Server:
Access the Domain Runtime MBean Server through JNDI.
If the classes for the JMX client are part of a Jakarta EE module, such as an EJB or Web application, the JNDI name for the Domain Runtime MBean server is:
weblogic/jmx/domainRuntime
For example:
Initial context ctx = new InitialContext(); server = (MBeanServer)ctx.lookup("weblogic/jmx/domainRuntime");
If the classes for the JMX client are not part of a Jakarta EE module, the JNDI name for the Domain Runtime MBean Server is:
java:comp/jmx/domainRuntime
Note:
The Domain Runtime MBean Server is present only on the Administration Server. Therefore, since the
ctx.lookup()
call returns a reference to the local MBean Server, the lookup method can only be called when running on the Administration Server. If called when running on a managed server, aNameNotFound
exception is thrown.See Make Local Connections to the Domain Runtime MBean Server in Developing Custom Management Utilities Using JMX for Oracle WebLogic Server
-
Register your MBean using
MBeanServer.registerMBean(Object
object
,ObjectName
name
)
, where:object
represents an instance of your MBean implementation class.name
represents the JMX object name for your MBean.
When your application deploys, the WebLogic deployment service emits
ApplicationLifecycleEvent
notifications to all its registered listeners. When the listener receives apostStart
notification, it invokes itspostStart
method. See Programming Application Life Cycle Events in Developing Applications for Oracle WebLogic Server. -
-
In the same class, implement the
ApplicationLifecycleListener.preStop(ApplicationLifecycleEvent evt)
method.In your implementation of this method, invoke the
javax.management.MBeanServer.unregister(ObjectName
MBean-name
)
method to unregister your MBean. -
Register your class as an
ApplicationLifecycleListener
by adding the following element to theweblogic-application.xml
file of your application:<listener> <listener-class> fully-qualified-class-name </listener-class> </listener>
Parent topic: Instrumenting and Registering Custom MBeans
Package Application and MBean Classes
Package your MBean classes in the application's APP-INF
directory or
in a module's JAR, WAR or other type of archive file depending on the access that you want
to enable for the MBean.
For more information, see Additional Design Considerations.
Parent topic: Instrumenting and Registering Custom MBeans