2 Migrating XML APIs to Web Services
This chapter provides information about migrating existing Oracle Communications MetaSolv Solution (MSS) XML APIs to web services.
About MSS XML APIs
Previous releases of MSS including 6.2.1 supported XML APIs, which ran on WebLogic Server 10.3.1. The requirements for providing XML APIs are the following from WebLogic:
-
WebLogic Integration - WLI
-
WebLogic Workshop
The current release of MSS runs on a WebLogic Server release that does not support these requirements for providing XML APIs. Therefore, MSS provides a new set of web services to replace the XML API functionality. This chapter includes instructions on migrating to the web services, and a mapping of the XML API operations to the web service operations.
Migrating XML APIs to Web Services
Migrating XML APIs to web services can be done in several ways. The following sections describe a sampling of the possible migration paths:
Migrating XML APIs Developed Using Workshop Using Java
Since the XML APIs were developed using Workshop, it is not possible to migrate them using a tool. Therefore, the best way to migrate these XML APIs to web services is to write them in Java.
Figure 2-1 describes an overview of the migration steps.
Figure 2-1 Overview of Migration Steps Using Java
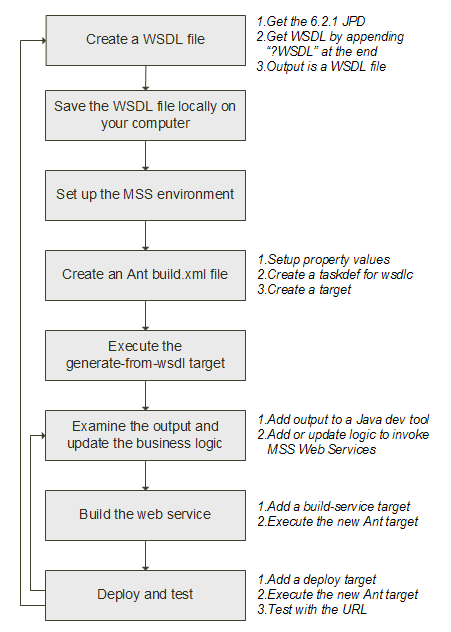
Description of "Figure 2-1 Overview of Migration Steps Using Java"
The following steps show an example of how to write Java functionality for a sample XML API:
-
Create a WSDL File. This step assumes you are using Java Process Definition (JPD) for your integration.
-
For your XML API, get the WSDL file for the API which must be migrated.
-
Find your JPD file (these have a .jpd or .java extension) for the XML API you are converting. You can get the WSDL file by adding “?WSDL" after the JPD file information.
http://hostname:port/custom/testAPI.jpd?WSDL
This will give you the complete WSDL for your JPD file.
Note:
In WebLogic Integration, Process Definition for Java (JPD) defines a business process.
-
-
Save the file to a new name, for example: testAPI.wsdl.
-
Set up your WebLogic Server and MSS environment.
Open a command window and execute the setDomainEnv.cmd (Windows) or setDomainEnv.sh (UNIX) script, located in the bin subdirectory of your domain directory.
-
Create a working directory, for example:
c:\opt\customWebService
where customWebService is the name of the XML API that you want to migrate.
-
Put your WSDL file into an accessible directory on your computer.
In this example, it is assumed that your WSDL file is called testAPI.wsdl and place the WSDL file in the '\customWebService \wsdl_file' directory.
-
Create a standard Ant build.xml file in the project directory. Set up the values of the property names for items such as the URL, server name, and password.
<project name="GenerateWS" default="all"> <property name="build" value="output" /> <property name="adminurl" value="t3://192.0.2.251:7001"/> //Server IP & Port <property name="serverName" value="m63server" /> // Server Name <property name="userName" value="weblogic" /> // User Name <property name="password" value="password" /> // Password <property name="ear.name" value="Custom_WebService" /> // Ear Name
In this example, the build.xml file will be located under the c:\opt\customWebService directory.
See "Sample Build XML File" for an example of a complete build.xml file.
-
Add a taskdef item to specify the full Java classname of the wsdlc task.
<taskdef name="jwsc" classname="weblogic.wsee.tools.anttasks.JwscTask" /> <taskdef name="wsdlc" classname="weblogic.wsee.tools.anttasks.WsdlcTask"/>
-
Create a generate-from-wsdl target and add the following to the wsdlc Ant task in the build.xml file:
<target name="generate-from-wsdl"> <wsdlc srcWsdl="wsdl_file/testAPI.wsdl" destJwsDir="jarJws" destImplDir="src" type="JAXWS" packageName="custom.mss.test"/> </target>
Before generating the web service from WSDL file, the following changes need to be made on the MSS WSDL file for a successful compilation:
-
Remove one <wsdl:port> which contains the name with JpdSoap under <wsdl:services>.
-
Remove the corresponding <wsdl:binding> for JpdSoap.
-
Remove the corresponding <wsdl:port type> for JpdSoap.
-
Remove the corresponding <wsdl:message> for both Input and Output messages.
-
-
Execute the generate-from-wsdl target to run the wsdlc Ant task by running this command line:
ant generate-from-wsdl
See the output directory to examine the artifacts and files generated by the wsdlc Ant task.
The wsdlc task in the examples generates the JAR file that contains the JWS Service Endpoint Interface (SEI) and JAXB data binding artifacts into the jarJws directory under the current directory.
It also generates a partial implementation file (testAPISoapImpl.java) of the JWS SEI into the src\custom\mss\test\ directory (which is a combination of the output directory specified by destImplDir and the directory hierarchy specified by the package name). All generated JWS files will be packaged in the custom.mss.test package.
-
Update the implementation file.
-
Run the wsimport Java command to generate the web service client files. The wsimport command creates JAX-WS portable artifacts that can be packaged in a web application archive or WAR file.
Refer to the following website for more information on the wsimport command:
https://docs.oracle.com/javase/8/docs/technotes/tools/unix/wsimport.html
-
Package the generated files with the web service project or package it as a JAR file and include it as a library.
-
Create a transaction to use for the API calls and do a lookup for the UserTransaction.
ctx = new InitialContext(env); utx = (UserTransaction) ctx.lookup("javax.transaction.UserTransaction");
where the env variable contains the server information.
-
Add code to convert the API input object to an MSS input object. (This is the same concept as xquery converter logic in Workshop.) This is an example passing an input document number of type String to the Start Order input object.
StartOrderByKeyRequest startOrderByKeyReq = new StartOrderByKeyRequest(); MetaSolvOrderKeyChoice metaSolvOrderKeyChoi = new MetaSolvOrderKeyChoice(); OrderKey ordKey = new OrderKey(); ordKey.setPrimaryKey("11111"); ordKey.setType("String");
-
Initialize the web service port.
OrderSoap ord = getWebService();
The getWebService() method is a separate method and contains the Atomic Transaction along with the web service reference which points to the server WSDL file. The @Transactional and @WebServiceRef are annotations used by the getWebService() method.
@Transactional(value = Transactional.TransactionFlowType.SUPPORTS, version = Transactional.Version.DEFAULT); @WebServiceRef(wsdlLocation = "http://ipaddress:port/MssWS/order/Order?WSDL", value = client.Order.class)Order service; private OrderSoap getWebService() { return service.getOrderSoap(); }
The client.Order.class is the Order class generated by wsimport command.
-
Start the transaction.
utx.begin();
-
Secure the web service port using the credentials.
List<CredentialProvider> credProviders = new ArrayList<CredentialProvider>(); String username = "username"; String password = "password"; CredentialProvider cp = new ClientUNTCredentialProvider(username.getBytes(), password.getBytes()); credProviders.add(cp); Map<String, Object> rc = ((BindingProvider)ord).getRequestContext(); rc.put(WSSecurityContext.CREDENTIAL_PROVIDER_LIST, credProviders);
-
Invoke the web service operation. For instance, call the start order by key web service.
StartOrderByKeyResponse StartOrderByKeyRes = ord.startOrderRequest(startOrderByKeyReq);
-
Map the MSS response object to the custom response object using converter logic.
-
Close the transaction.
utx.commit();
Note:
In addition to these steps, ensure that all exceptions are handled properly using a catch block, and throw the error encountered.
For multiple operations, you must repeat these steps and the transaction has to be handled.
-
-
Build the web service.
-
Add a build-service target to the build.xml file that executes the jwsc Ant task against the updated JWS implementation class. Use the compiledWsdl attribute of jwsc task to specify the name of the JAR file generated by the wsdlc Ant task:
<target name="build-service"> <mkdir dir="${build}/${ear.name}" /> <jwsc srcdir="src" destdir="${build}/${ear.name}"> <jws file="src/custom/mss/test/testAPISoapImpl.java" com-piledWsdl="jarJws/testAPI_wsdl.jar" type="JAXWS"/> </jwsc> </target>
-
Ensure you have the wsimport client class files or the JAR file in the classpath. This is required to successfully compile the implementation files.
-
After adding the build-service target, go to command prompt and run the following command
ant build-service
This will compile the web service class that contains the business logic.
-
-
Generate the custom web service EAR file using build.deliverable target.
<target name="build.deliverable" depends="build-service" description="Generates the WebService EAR"> <delete file="Custom_WebService.ear"/> <ear destfile="Custom_WebService.ear" appxml="descriptors/META-INF/application.xml"> <fileset dir="output/${ear.name}"> <include name="**"/> </fileset> </ear> </target>
This target creates an EAR file using the contents in output/earFileName directory.
-
Deploy the EAR file on the server:
-
Deploy the web service, packaged in an Enterprise Application, to WebLogic Server, using the wldeploy Ant task.
-
To use the wldeploy Ant task, add the following target to the build.xml file:
<taskdef name="wldeploy" classname="weblogic.ant.taskdefs.management.WLDeploy"/> <target name="deploy"> <wldeploy action="deploy" name="wsdlcEar" source="output/wsdlcEar" user="${username}" password="${password}" verbose="true" adminurl="t3://${hostname}:${port}" targets="${server.name}" /> </target>
Substitute the values for username, password, hostname, port, and server.name that correspond to your WebLogic Server instance.
-
Deploy the file by executing the deploy target:
ant deploy
-
-
Test that the web service is deployed correctly by invoking its WSDL in your browser:
http://hostname:port/custom/mss/test/testAPI?WSDL
The context path and service URI section of the preceding URL are specified by the original WSDL. Use the hostname and port relevant to your WebLogic Server instance. The deployed and original WSDL files are the same, except for the host and port of the endpoint address.
For more information on “Developing JAX-WS Web Services for Oracle WebLogic Server", refer to the following Oracle WebLogic Server website:
For 12.2.1.4: https://docs.oracle.com/en/middleware/fusion-middleware/weblogic-server/12.2.1.4/wsget/jax-ws-setenv.html
Migrating XML APIs Using Oracle SOA Suite
Oracle SOA Suite enables system developers to set up and manage services and to orchestrate them into composite applications and business processes. Oracle SOA Suite runs on the same platform as WebLogic Integration (WebLogic Server).
To migrate a Workshop project to an Oracle SOA Suite project, you must design and build it. In Oracle SOA Suite, workflows are defined as Business Process Execution Language (BPEL) files. You create these files in the same way as you create JPD files in Workshop. Oracle SOA Suite has features which are similar to Workshop features that assist in the design process of workflows.
See the following website for information on the community of joint WLI/SOA users and the “SOA Suite Essentials for WLI users" series:
https://www.oracle.com/technical-resources/articles/middleware/soa-wli-bpel-transactions.html
Migrating XML APIs Using a Java Class
Often you can have a call to an XML API as part of integration code that may call into several different systems. This section addresses how a simple Java class can invoke MSS Web Services.
The following steps show an example of how to write a Java class to invoke MSS Web Services:
-
Create a Java class with a main method that invokes another method, for example getOrderDetails(). The getOrderDetails() method calls the web service to retrieve order details and also contains the invocation logic.
public static void main(String[] args) { getOrderDetails(); }
-
Create the method getOrderDetails() that invokes the MSS Web Service.
-
The first step of this method is to determine the WSDL URL and add a definition for that string variable. For instance, the following contains a line of code with a sample URL format for the Order Web Service:
String url = "http://username:password@ipAddress:port/MssWS/order/Order?WSDL";
where:
-
username is the username to log in to the web service
-
password is the password to log in to the web service
-
ipAddress is the IP Address for the WSDL location, such as 192.0.2.12
-
port is the port for the WSDL location, such as 1521
-
-
Define the soap message which is passed in as input XML.
Note:
The input XML can be read in from a file. These details are included to illustrate how the input message is built.
MessageFactory mf = MessageFactory.newInstance(); SOAPMessage message = mf.createMessage(); SOAPPart soapPart = message.getSOAPPart();
-
Define the message header with the XML namespaces and add these to the envelope.
/* XML NameSpaces */ String soapenv = "http://schemas.xmlsoap.org/soap/envelope/"; String open="http://www.openuri.org/"; String ord="http://xmlns.oracle.com/communications/mss/OrderManagementAPI"; String ord1="http://xmlns.oracle.com/communications/mss/OrderManagementEntities"; String com="http://java.sun.com/products/oss/xml/Common"; String wsse = "http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-secext-1.0.xsd"; String wsu = "http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-utility-1.0.xsd"; /* Adding Name Spaces to the Envelope */ SOAPEnvelope envelope = soapPart.getEnvelope(); envelope.addNamespaceDeclaration("soapenv", soapenv); envelope.addNamespaceDeclaration("open", open); envelope.addNamespaceDeclaration("ord", ord); envelope.addNamespaceDeclaration("ord1", ord1); envelope.addNamespaceDeclaration("com", com); envelope.addNamespaceDeclaration("wsse", wsse); envelope.addNamespaceDeclaration("wsu", wsu); SOAPHeader soapheader = envelope.getHeader();SOAPBody soapbody = envelope.getBody();
-
Because MSS Web Services are secured, you must pass proper credentials which update the security header.
SOAPElement soapsecurity = soapheader.addChildElement("Security","wsse"); QName soapsecurityattribute = new QName("soapenv:mustUnderstand"); soapsecurity.addAttribute(soapsecurityattribute, "1"); SOAPElement soapusernametoken = soapsecurity.addChildElement("UsernameToken","wsse"); QName usernameattribute = new QName("wsu:Id"); soapusernametoken.addAttribute(usernameattribute, "UsernameToken-983A273E3EDA90F960148681422777119"); SOAPElement soapusername = soapusernametoken.addChildElement("Username","wsse"); soapusername.addTextNode("yourUsername"); SOAPElement soappassword = soapusernametoken.addChildElement("Password","wsse"); QName passwordattribute = new QName("Type"); soappassword.addAttribute(passwordattribute, "http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-username-token-profile-1.0#PasswordText"); /* password is your password value set in the addTextNode method */ soappassword.addTextNode("password");
-
Add the contents to the message body.
/* Add the SOAP body */ SOAPElement soapgetOrder = soapbody.addChildElement("getOrderByKey", "open"); SOAPElement soapmomGetOrderByKeyRequest = soapgetOrder.addChildElement("momGetOrderByKeyRequest", "ord"); SOAPElement soapmommekey = soapmomGetOrderByKeyRequest.addChildElement("mommekey","ord"); SOAPElement soapmetaSolvOrderKey = soapmommekey.addChildElement("metaSolvOrderKey","ord1"); SOAPElement soapapplicationDN = soapmetaSolvOrderKey.addChildElement("applicationDN","com"); SOAPElement soaptype = soapmetaSolvOrderKey.addChildElement("type","com"); SOAPElement soapprimaryKey = soapmetaSolvOrderKey.addChildElement("primaryKey","ord1"); /* This DOCUMENT_NUMBER is for the order number */ soapprimaryKey.addTextNode(DOCUMENT_NUMBER);
-
Add the code to invoke the web service. The soapResponse variable contains the output details.
/* Call with the SOAP message being returned */ SOAPMessage soapResponse = con.call(message, url);
Mapping Existing XML APIs to Web Services
The goal of the web services release is that the web service functionality matches the functionality of the XML APIs. However, there are some changes to the method names and to some schema file names. Table 2-1 shows the changes from the XML APIs to the web service operation names.
Note:
Table 2-1 through Table 2-10 refer to changes in moving from the XML APIs to the web services for MSS Release 6.3. Table 2-11 refers to changes made in MSS Release 6.3.0.1.
Table 2-1 Mapping XML APIs to Web Services
XML API Method Name | Web Service Operation Name |
---|---|
GetServiceActivationData |
getActivationData |
DeleteCustomerAccount |
deleteCustomerRequest |
GetCustomerAccountSync |
getCustomerAccountByKey |
ImportCustomerAccountSync |
importCustomerAccount |
GetIntegrationEvent |
getIntegrationEventData |
InboundEventStatusUpdate |
updateInboundEventStatus |
UpdateIntegrationEvent |
updateIntegrationEventStatus |
OutboundEventStatusUpdate |
updateOutboundEventStatus |
AuditTrail |
auditTrailRecording |
CreateEntitySync |
createEntityByValueRequest |
CreateLocationSync |
createLocationRequest |
CreateNewInventoryItem |
createNewInventoryItemRequest |
DeleteLocationSync |
deleteLocationRequest |
GetAvailablePhysicalPorts |
getAvailablePhysicalPortsRequest |
GetDlrByKeySync |
getDlrByKeyRequest |
GetDlrByOrderKeySync |
getDlrByOrderKey |
GetEntityByKeySync |
getEntityByKeyRequest |
GetIPAddresses |
getIpAddressesRequest |
GetLocationSync |
getLocationRequest |
GetNetworkAreasByGeoArea |
getNetworkAreasByGeoAreaRequest |
GetNetworkComponents |
getNetworkComponentsRequest |
GetServiceLocationSync |
getServiceLocationRequest |
CreateInventoryAssociation |
inventoryAssociationRequest |
TNRecall |
processTNRecallRequestDocument |
QueryEndUserLocation |
queryEndUserLocation |
QueryInventoryManagementSync |
queryInventoryManagementRequest |
QueryNetworkLocation |
queryNetworkLocation |
TNValidation |
tnValidationRequest |
UpdateEntitySync |
updateEntityByValueRequest |
UpdateLocationSync |
updateLocationRequest |
UpdateTelephoneNumber |
updateTNRequest |
AddTaskJeopardy |
addTaskJeopardyRequest |
AssignProvPlanSync |
assignProvPlanRequest |
CompletOrderTask |
completeTaskRequest |
CreateAttachment |
createAttachment |
CreateISROrderSync |
createISROrderRequest |
CreateOrderMainSync |
createOrderRequest |
CreateOrderRelationship |
createOrderRelationship |
CreatePSROrderSync |
createPSROrderRequest |
GetCnamData |
getCnamData |
GetE911Data |
getE911Data |
GetLidbData |
getLidbData |
GetPSROrderByTN |
getPSROrderByTN |
GetPSROrderSync |
getOrderByKey |
GetTaskJeopardy |
TaskJeopardyRequest |
GetTaskViewDetail |
TaskRequest |
ProcessBillingTelephoneNumber |
billingTelephoneNumberRequest |
ProcessSuppOrder |
processSuppOrderRequest |
QueryOrderManagementSync |
queryOrderManagementRequest |
ReopenTask |
reopenTaskRequest |
StartOrderSync |
startOrderRequest |
TransferTaskSync |
transferTaskRequest |
UpdateCnamData |
updateCnamData |
UpdateE911Data |
updateE911DataRequest |
UpdateEstimatedCompletionDate |
updateEstimationCompletedDateRequest |
UpdateGatewayEventSync |
updateGWEventRequest |
UpdateLidbData |
updateLidbData |
UpdateOrderMainSync |
updateOrderRequest |
UpdatePSROrderSync |
updatePSROrderRequest |
CreateSoaMessage |
createSoaMessageRequest |
GetSoaDefaults |
getSoaDefaultsRequest |
GetSoaInformation |
getSoaInformationRequest |
GetSoaMessagesToSend |
getSoaMessageToSendRequest |
GetSoaTnsForOrder |
getSoaTnsForOrderRequest |
SetTnSoaComplete |
setTnSoaCompleteRequest |
Table 2-2 through Table 2-10 show the details of the following:
-
An element or complex type name change.
-
An XSD definition change.
Table 2-2 OrderManagementData.xsd
Before | After |
---|---|
PsrOrderItemPriceType |
MomPsrOrderItemPriceType |
LocationTypeEnumType |
MomLocationTypeEnumType |
Table 2-3 OrderManagementEntities.xsd
Before | After |
---|---|
name="primaryKey" type="string" nillable="true" minOccurs="0" |
name="primaryKey" type="string" minOccurs="1" |
name="taskPrimaryKey" type="string" |
name="taskPrimaryKey" type="string" minOccurs="1" |
Table 2-4 ServiceData.xsd
Before | After |
---|---|
HuntGroupType |
MsdHuntGroupType |
TrunkGroupType |
MsdTrunkGroupType |
ISDNTrunkGroupInformationType |
MsdISDNTrunkGroupInformationType |
Table 2-5 ServiceEntities.xsd
Before | After |
---|---|
name="serviceSpecificationPrimaryKey" type="string" nillable="true" minOccurs="0" |
name="serviceSpecificationPrimaryKey" type="string" minOccurs="1" |
name="servicePrimaryKey" type="string" default="0" nillable="true" minOccurs="0" |
name="servicePrimaryKey" type="string" default="0" minOccurs="1" |
Table 2-6 InventoryManagementEntities.xsd
Before | After |
---|---|
LocationValue |
MimLocationValue |
name="networkLocationPrimaryKey" type="string" nillable="true" minOccurs="0" |
name="networkLocationPrimaryKey" type="string" minOccurs="1" |
name="endUserLocationPrimaryKey" type="string" nillable="true" minOccurs="0" |
name="endUserLocationPrimaryKey" type="string" minOccurs="1" |
Table 2-7 CustomerManagementAPI.xsd
Before | After |
---|---|
key |
mcmmekey |
value |
mcmmevalue |
Table 2-8 OrderManagementAPI.xsd
Before | After |
---|---|
PsrOrderItemPriceType |
PsrOrderItemPriceType |
LocationTypeEnumType |
MomLocationTypeEnumType |
QueryValue |
MomQueryValue |
key |
mommekey |
value |
mommevalue |
addTaskJeopardyRequest |
momAddTaskJeopardyRequest |
createAttachmentResponse |
momCreateAttachmentResponse |
createOrderRelationshipResponse |
momCreateOrderRelationshipResponse |
getE911DataResponse |
momGetE911DataResponse |
getCNAMDataResponse |
momGetCnamDataResponse |
getPSROrderByTNResponse |
momGetPSROrderByTNResponse |
getLIDBDataResponse |
momGetLIDBDataResponse |
getOrderByKeyRequest |
momGetOrderByKeyRequest |
getOrderByKeyResponse |
momGetOrderByKeyResponse |
queryOrderManagementRequest |
momQueryOrderManagementRequest |
processSuppOrderRequest |
momProcessSuppOrderRequest |
reopenTaskRequest |
momReopenTaskRequest |
transferTaskRequest |
momTransferTaskRequest |
updateE911DataRequest |
momUpdateE911DataRequest |
Table 2-9 ServiceOrderActivationAPI.xsd
Before | After |
---|---|
createSOAMessageRequest |
msoaCreateSOAMessageRequest |
getSOADefaultsRequest |
msoaGetSOADefaultsRequest |
getSOAInformationRequest |
msoaGetSOAInformationRequest |
getSOATNsForOrderRequest |
msoaGetSOATNsForOrderRequest |
setTNSOACompleteRequest |
msoaSetTNSOACompleteRequest |
Table 2-10 InventoryManagementAPI.xsd
Before | After |
---|---|
queryValue |
MimQueryValue |
queryResponse |
MimQueryResponse |
getAvailablePhysicalPortsRequest |
mimGetAvailablePhysicalPortsRequest |
CreateLocationRequest |
mimCreateLocationRequest |
GetLocationRequest |
mimGetLocationRequest |
UpdateLocationRequest |
mimUpdateLocationRequest |
createEntityByValueRequest |
mimCreateEntityByValueRequest |
updateEntityByValueRequest |
mimUpdateEntityByValueRequest |
getEntityByKeyRequest |
mimGetEntityByKeyRequest |
queryInventoryManagementRequest |
mimQueryInventoryManagementRequest |
updateTNRequest |
mimUpdateTNRequest |
getNetworkComponentsRequest |
mimGetNetworkComponentsRequest |
getNetworkAreasByGeoAreaRequest |
mimGetNetworkAreasByGeoAreaRequest |
getIpAddressesRequest |
mimGetIpAddressesRequest |
createNewInventoryItemRequest |
mimCreateNewInventoryItemRequest |
queryNetworkLocationResponse |
mimQueryNetworkLocationResponse |
queryEndUserLocationResponse |
mimQueryEndUserLocationResponse |
Note:
Table 2-11 describes the schema changes in MSS Release 6.3.0.1.
Table 2-11 ServiceOrderActivationData.xsd
Before | After |
---|---|
NpaNxxNumericStringType length value="3" NpaNxxNumericStringType pattern value="[0123456789]" |
NpaNxxNumericStringType pattern value="[0-9]{3}" |
LineRangeNumericStringType length value="4" LineRangeNumericStringType pattern value="[0123456789]" |
LineRangeNumericStringType pattern value="[0-9]{4}" |
Functional Differences from the XML APIs
The following sections highlight areas that have changed from the XML APIs to the new MSS Web Services.
Empty Structures on Responses
In using the XML APIs a subordinate or child structure may be returned with empty data. In using the MSS Web Services, subordinate structures are only returned with data for a response. For instance, if you retrieve a PSR order with a web service operation and the user data does not exist, the user data structure is not returned as part of the response.
Get DLR Response Field Changes
In retrieving the Design Layout Report (DLR) details using the getServiceRequestDLRs operation, the field values for the circuit type are modified. Table 2-12 shows the before and after values for the 'type' field in the DLRResult complexType.
Table 2-12 Changes to the 'type' Field in DLRResult
Field Name | Description | Before | After |
---|---|---|---|
type |
Circuit type in DLRResult |
C F S T P V B |
Connection Facility Special Trunk Product Virtual Bandwidth |
Sample Build XML File
In the section "Migrating XML APIs Developed Using Workshop Using Java" sections of an Ant build.xml file are described. For reference, Example 2-1 shows a complete sample build.xml file. This XML file is used as an input to Ant to run the targets defined in the file.
Example 2-1 Full build.xml File
<project name="GenerateWS" default="all"> <property name="build" value="output"/> <property name="adminurl" value="t3://192.0.2.251:7001"/> // Server IP and Port <property name="serverName" value="m63server"/> // Server Name <property name="userName" value="weblogic"/> // User Name <property name="password" value="password"/> <property name="ear.name" value="Custom_WebService"/> // Ear Name <property name="main_dist.dir" value="../../../dist/lib"/> <taskdef name="jwsc" classname="weblogic.wsee.tools.anttasks.JwscTask"/> <taskdef name="clientgen" classname="weblogic.wsee.tools.anttasks.ClientGenTask"/> <taskdef name="wsdlc" classname="weblogic.wsee.tools.anttasks.WsdlcTask"/> <taskdef name="wldeploy" classname="weblogic.ant.taskdefs.management.WLDeploy"/> <target name="all" depends="generate-from-wsdl,wait"/> <target name="generate-from-wsdl"> <wsdlc srcWsdl="wsdl_file/testAPI.wsdl" destJwsDir="jarJws" destImplDir="src" type="JAXWS" packageName="custom.mss.test"/> </target> <target name="wait"> <echo message=" Now you need to provide your own Implementation for sayHello() of [[[ ws/testAPISoapImpl.java ]]]"/> <echo message=" then you need to run 'ant deploy' to rebuild your edited Service and to Deploy it Mon the Server..."/> </target> <target name="build-service"> <mkdir dir="${build}/${ear.name}"/> <jwsc srcdir="src" destdir="${build}/${ear.name}"> <jws file="src/custom/mss/test/testAPISoapImpl.java" com-piledWsdl="jarJws/testAPI_wsdl.jar" type="JAXWS"/> </jwsc> </target> <target name="build.deliverable" depends="build-service" description="Generates the WebService EAR"> <delete file="Custom_WebService.ear"/> <ear destfile="Custom_WebService.ear" appxml="descriptors/META-INF/application.xml"> <fileset dir="output"> <include name="**"/> </fileset> </ear> </target> <target name="deploy"> <wldeploy action="deploy" name="wsdlcEar" source="output/wsdlcEar" user="${username}" password="${password}" verbose="true" adminurl="t3://${hostname}:${port}" targets="${server.name}"/> </target> </project>