Converged Application Server Profile API
The IMS specification defines the Sh interface as the method of communication between the Application Server (AS) function and the Home Subscriber Server (HSS), or between multiple IMS Application Servers. The AS uses the Sh interface in two basic ways:
- To query or update a user's data stored on the HSS
- To subscribe to and receive notifications when a user's data changes on the HSS
The user data available to an AS may be defined by a service running on the AS (repository data), or it may be a subset of the user's IMS profile data hosted on the HSS. The Sh interface specification, 3GPP TS 29.328 V5.11.0, defines the IMS profile data that can be queried and updated via Sh. All user data accessible via the Sh interface is presented as an XML document with the schema defined in 3GPP TS 29.328.
The IMS Sh interface is implemented as a provider to the base Diameter protocol support in Converged Application Server. The provider transparently generates and responds to the Diameter command codes defined in the Sh application specification. A higher-level Profile Service API enables SIP Servlets to manage user profile data as an XML document using XML Document Object Model (DOM). Subscriptions and notifications for changed profile data are managed by implementing a profile listener interface in a SIP Servlet.
Figure 2-4 Profile Service API and Sh Provider Implementation
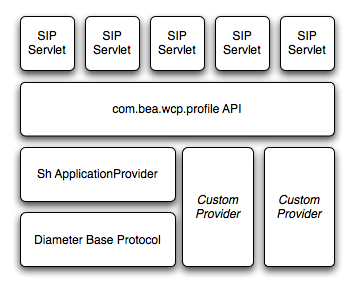
Converged Application Server includes only a single provider for the Sh interface. Future versions of Converged Application Server may include new providers to support additional interfaces defined in the IMS specification. Applications using the profile service API will be able to use additional providers as they are made available.
Using Document Keys for Application-Managed Profile Data
Servlets that manage profile data can explicitly obtain an Sh XML document from a factory using a key, and then work with the document using DOM.
The document selector key identifies the XML document to be retrieved by a
Diameter interface, and uses the format protocol://
uri/
reference_type[/
access_key]
.
The example below summarizes the required document selector elements for each type of Sh data reference request.
Table 2-1 Summary of Document Selector Elements for Sh Data Reference Requests
Data Reference Type | Required Document Selector Elements | Example Document Selector |
---|---|---|
RepositoryData |
sh://uri/reference_type/Service-Indication |
sh://sip:user@oracle.com/RepositoryData/Call Screening/ |
IMSPublicIdentity |
sh://uri/reference_type/[Identity-Set] where Identity-Set is one of:
|
sh://sip:user@oracle.com/IMSPublicIdentity/Registered-Identities |
IMSUserState |
sh://uri/reference_type |
sh://sip:user@oracle.com/IMSUserState/ |
S-CSCFName |
sh://uri/reference_type |
sh://sip:user@oracle.com/S-CSCFName/ |
InitialFilterCriteria |
sh://uri/reference_type/Server-Name |
sh://sip:user@oracle.com/InitialFilterCriteria/www.oracle.com/ |
LocationInformation |
sh://uri/reference_type/(CS-Domain | PS-Domain) |
sh://sip:user@oracle.com/LocationInformation/CS-Domain/ |
UserState |
sh://uri/reference_type/(CS-Domain | PS-Domain) |
sh://sip:user@oracle.com/UserState/PS-Domain/ |
Charging information |
sh://uri/reference_type |
sh://sip:user@oracle.com/Charging information/ |
MSISDN |
sh://uri/reference_type |
sh://sip:user@oracle.com/MSISDN/ |
Converged Application Server provides a helper class,
com.bea.wcp.profile.ProfileService
, to help you easily retrieve a
profile data document. The getDocument()
method takes a constructed
document key, and returns a read-only org.w3c.dom.Document
object. To
modify the document, you make and edit a copy, then send the modified document and key
as arguments to the putDocument()
method.
See "Using the Profile Service API (Diameter Sh Interface)" in Converged Application Server Diameter Application Development Guide for more information.
Monitoring Profile Data
The IMS Sh interface enables applications to receive automatic notifications when a subscriber's profile data changes. Converged Application Server provides an easy-to-use API for managing profile data subscriptions. A SIP Servlet registers to receive notifications by implementing the com.bea.wcp.profile.ProfileListener
interface, which consists of a single update method that is automatically invoked when a change occurs to profile to which the Servlet is subscribed. Notifications are not sent if that same Servlet modifies the profile information (for example, if a user modifies their own profile data).
Actual subscriptions are managed using the subscribe method of the com.bea.wcp.profile.ProfileService
helper class. The subscribe method requires that you supply the current SipApplicationSession
and the key for the profile data document you want to monitor. See "Using Document Keys for Application-Managed Profile Data" for more information.
Applications can cancel subscriptions by calling ProfileSubscription.cancel()
. Also, pending subscriptions for an application are automatically cancelled if the application session is terminated.
Example 2-7 shows sample code for a Servlet that implements the ProfileListener
interface.
Example 2-7 Sample Servlet Implementing ProfileListener Interface
package demo; import com.bea.wcp.profile.*; import javax.servlet.sip.SipServletRequest; import javax.servlet.sip.SipServlet; import org.w3c.dom.Document; import java.io.IOException; public class MyServlet extends SipServlet implements ProfileListener { private ProfileService psvc; public void init() { psvc = (ProfileService) getServletContext().getAttribute(ProfileService.PROFILE_SERVICE); } protected void doInvite(SipServletRequest req) throws IOException { String docSel = "sh://" + req.getTo() + "/IMSUserState/"; // Subscribe to profile data. psvc.subscribe(req.getApplicationSession(), docSel, null); } public void update(ProfileSubscription ps, Document document) { System.out.println("IMSUserState updated: " + ps.getDocumentSelector()); } }
The ProfileListener
interface is handled similar to the TimerService
provided by JSR 116 for application timers. Multiple Servlets in an application may implement the ProfileListener
interface, but only one Servlet may act as a listener. The SIP deployment descriptor for the application must designate the profile listener class in the set of listeners as shown in Example 2-8.
Example 2-8 Declaring a ProfileListener
<listener> <listener-class>com.foo.MyProfileListener</listener-class> Declaring a ProfileListener </listener> <listener> <listener-class>com.foo.MyProfileListener</listener-class> </listener>
Example 2-7 can be rewritten using an annotated POJO as shown in Example 2-9.
Example 2-9 Sample Servlet Implementing ProfileListener Interface Using an Annotated POJO
package demo; // Includes excluded for brevity... @SipServlet(loadOnStartup = 1); public class MyServlet implements ProfileListener { private ProfileService psvc; @Inject SipFactory sipFactory; public void init() { psvc = (ProfileService) getServletContext().getAttribute(ProfileService.PROFILE_SERVICE); } @Invite protected void handleInvite(SipServletRequest req) throws IOException { String docSel = "sh://" + req.getTo() + "/IMSUserState/"; // Subscribe to profile data. psvc.subscribe(req.getApplicationSession(), docSel, null); } public void update(ProfileSubscription ps, Document document) { System.out.println("IMSUserState updated: " + ps.getDocumentSelector()); } }