C C++ SRP API Template Design
This chapter is intended for C++ SRP API developers and designers who have no prior experience developing the C++ SRP API. The following sections are included:
API library structures
Figure C-1 illustrates the APIs that can be linked to a C++ SRP API.
The C++ SRP API provides the following capabilities:
-
Interface protocols with external systems.
-
Functionalities of the C++ SRP API library
-
Controls data transfer between upstream systems (submitting work order to ASAP for provisioning) and the SARM.
Figure C-2 Data Transfer Through the C++ SRP API
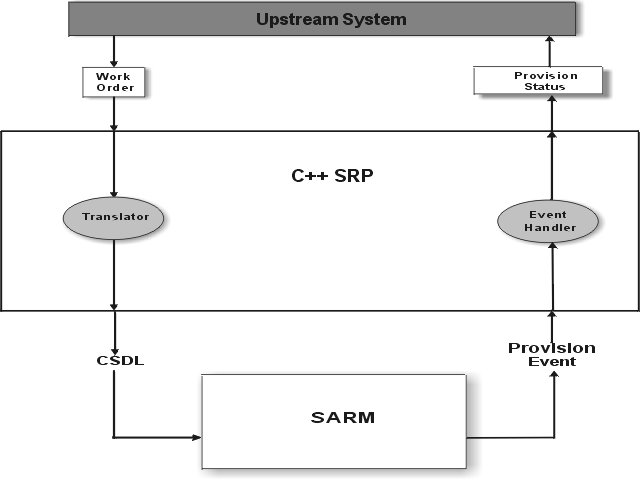
Description of "Figure C-2 Data Transfer Through the C++ SRP API"
Fundamental tasks performed by all C++ SRP API:
-
Converts work orders containing service requests in their native format to CSDL and sends work order to the SARM for provisioning.
-
Returns the work order provisioning status passed from the SARM to the upstream system.
C++ SRP API library
The C++ SRP API library is a library in ASAP to let the user applications interface with SARM. In addition, the library will help the users to develop SRP in ASAP with an object-oriented interface. It provides the POSIX thread interfaces.
The C++ SRP API library provides the following functionality:
-
OO interface to generate and submit work orders.
-
OO interface to manipulate work orders.
-
OO interface to process work orders.
-
OO interface to retrieve work order information in ASAP.
-
ASAP high availability.
-
Reliable communication between SRP and SARM.
Common object library (liboo_asc)
The liboo_asc library provides you with the following functionality:
-
Diagnostic message logging facility to the application programmers.
-
System event generation.
-
Application configuration parameter determination.
-
Remote Procedure Calls API.
-
Network connection management.
C++ SRP API components
Non-interfering tasks are separated into threads in the C++ SRP. Different threads (non-interfering task) can process their own task at the same time to improve performance (increase parallel processing). The threads in C++ server are:
Work order submission
-
Receiver – Establishes connection and receives service request data from upstream system.
-
Translator – Translates service request in native format to CSDL command, Work Order and Parameters.
-
SARM Driver – Submits ASAP work order to SARM for ASAP provisioning.
Communication between threads
Threads communicate with each other using the message queue.
-
Synchronous Message Transfer – Message sender waits for the message receiver to finish processing (Sequential).
-
Asynchronous Message Transfer – Message sender does not wait for message receiver to finish processing (Parallel).
The following message queues are used in the communication between threads:
-
Translator message queue – The receiver thread sends a pointer to a work order structure containing a service request in native format to a translator thread.
-
SARM Driver message queue – The translator thread sends a pointer to the ASAP work order structure containing the CSDL commands to the SARM Driver thread.
-
Work Order Manager message queue – The work order manager thread sends the provision status to the event handler thread.
-
Sender message queue – Event handler passing message structure returns to Upstream system to sender thread.
Multiple instances of threads
Multiple instances of the same type of thread can process multiple work orders in parallel. The number of thread instances can be configured in the configuration file to obtain optimal results. To improve performance, multiple work orders can be processed at the same time. The complexity of the C++ SRP increases due to the synchronization between threads and work order dependency.
Communications with ASAP internal systems
The C++ SRP maintains constant connection with the following internal systems:
-
SARM – C++ SRP API submits CSDL commands and obtains provisioning status.
-
SRP database – C++ SRP API obtains static information from the SRP database and may use the database to store work order information.
Communication between C++ SRP API and SARM
-
SARM Driver thread and the Work Order Manager thread in the CsolSrp Library, and the SRP Driver thread in SARM, handle the connections between the C++ SRP API and SARM.
-
Sybase Open Client RPC handles the data transfer between the C++ SRP API and SARM. The Socket RPC message goes from SARM to the C++ SRP API.
Communication between C++ SRP API and SRP database
-
C++ SRP API maintains a pool of connections with the SRP database using the Sybase Open Client connection routines.
-
Any thread in the C++ SRP API can allocate connection from the pool using ClntProcMgr Class.
-
RPCs are preferred over SQL statements to transfer data between the C++ SRP API and the SRP database.
Upstream system interface
This section describes upstream system interface.
Protocol
The Upstream System interface protocol consists of:
-
Communication between the C++ SRP API and Upstream systems
-
TCP/IP Sockets
-
Connection Verification
Communication between C++ SRP API and upstream systems
-
Sender thread and Receiver thread in each C++ SRP API handles the connections between C++ SRP API and upstream system.
-
Connection method is site specific.
-
Commonly used communication protocol, for example, TCP/IP (socket), Open Client/Server Language Communication, SNA LU 6.2).
-
Routines must be thread-saved with the Sybase multithread library.
-
Synchronous communication is used, for example, acknowledgement required for data transfer.
TCP/IP sockets
-
Implementation of TCP/IP requires a new thread (Connection Handler thread) in the C++ SRP API server.
-
Connection Handler Thread [server] constantly waits for connection from the upstream system [client].
-
IP address and port number belong to the upstream system, and the C++ SRP API is defined in command line or input file.
-
To create a socket, the Connection Handler Thread uses listen.
-
To wait for I/O from the upstream system and connection socket, Accept is used.
-
To handle the data transfer between C++ SRP API and upstream system, the Connection Handler thread spawns the Receiver thread.
-
Read and write are used for data transfer between receiver and upstream system.
Data format
The data format used must be the same for both the upstream system and customized SRP.
Input message from upstream system
The message from the upstream system received by receiver thread, for example, service request.
Label value pair:
-
Linear representation of the work order.
-
Simple format, hard to represent complex work order.
-
API function get_name_value( ) can extract the work order with the format label=value;\n.
Synchronous processing
-
The C++ SRP API returns the acknowledgment to the Upstream system after the work order submits to SARM.
-
Upstream system submits the next work order after it receives an acknowledgment from the C++ SRP API.
Asynchronous processing
-
The C++ SRP API returns acknowledgment to the Upstream system after the C++ SRP API successfully receives a message from the Upstream system.
-
Upstream system submits the next work order after it receives an acknowledgement from the C++ SRP API.
-
The C++ SRP API translates the work order and submits the work order to SARM. During this time, the current work order has no persistence (for example, if the C++ SRP API system goes down, the work order cannot be recovered).
-
The C++ SRP API returns to the Upstream system after the work order successfully submits to SARM.
-
Upstream system needs to maintain the work order submitted to the C++ SRP API until the C++ SRP API successfully submits the work order to SARM.
Figure C-7 Synchronous and Asynchronous C++ SRP API Processing
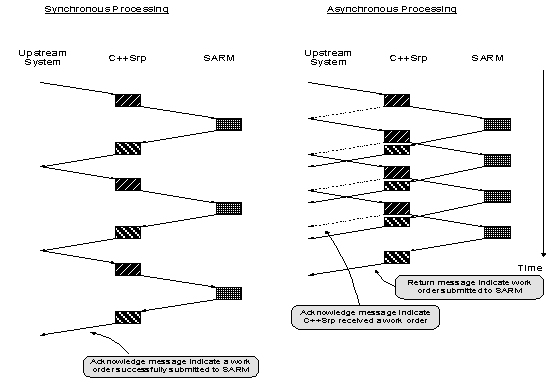
Description of "Figure C-7 Synchronous and Asynchronous C++ SRP API Processing"
If Upstream system can persist, the work order is submitted to the C++ SRP API before the work order is submitted to the SARM, therefore work order dependency is not important or is maintainable. The asynchronous processing is recommended because of its faster throughput.
Table C-1 Processing characteristics
Synchronous | Asynchronous |
---|---|
Receiver and Translator thread process in sequence. |
Receiver and Translator thread process in parallel. |
SRP return SARM submission status to upstream through receiver thread. |
C++ SRP API return SARM submission status through sender thread (other connect to upstream system). |
Slow throughput. |
Fast throughput for work order submission. |
Simpler Implementation |
Harder implementation |
Easy re-submission of work order during system failure. |
Hard implementation of re-submission of work order during system failure. |
Upstream system does not need to maintain work order once acknowledgement received from SRP. |
Upstream system needs to maintain work order after acknowledgement received, until the SRP returns stating work order successfully saved in SARM. |
Work Order dependency preserves because work order submits to SARM in the same sequence work order submitted from Upstream. |
Work Order dependency needs to manage by C++ SRP API because work order might not submit to SARM in the same sequence work order submits from upstream system. |
If throughput is not as important, synchronous processing should be used for simple implementation.
Single and multiple connections
If the volume of depending work order is very low and the upstream system can handle multiple connections, you should use multiple connections for increased parallel processing (better speed).
If the volume of depending work order is high, you should use a single connection for easier implementation.
Table C-2 Single and multiple connections
Single Connection | Multiple Connections |
---|---|
One receiver thread in C++ SRP system submits work order to translator thread. |
Multiple receiver threads submit work order message to translator thread. |
Work order dependency can be maintained if the submission sequence is the same as the dependency sequence. |
Method handling work order dependency is required if the depending work order can be sent through a different connection. |
Easier implementation. |
Complex implementation. |
Sequential processing. |
Parallel processing. |
Low throughput. |
High throughput. |
No backup connection if connection fails. |
Backup connection available when one connection fails. |
API libraries
The following API libraries are used to develop SRPs:
-
Common API (liboo_asc) – Network connection handling functions.
-
Thread API (libthreadfw) – Multithread Server routines.
-
C++ SRP API (libCsolSrp) – CsolSrp required routines
-
Interpreter API (libinterpret) – Interpreter routines
Main( )
The program starts up in single-thread mode. The initialization code in main( ) is executed while the process is still in single-thread mode. After initialization, the process turns multi-thread.
Application threads are spawned and wait for the initialization thread to release the initialization mutex. When the initialization thread has finished, it releases the initialization mutex. Application threads can start its process.
Figure C-8 Multithread Server Initialization
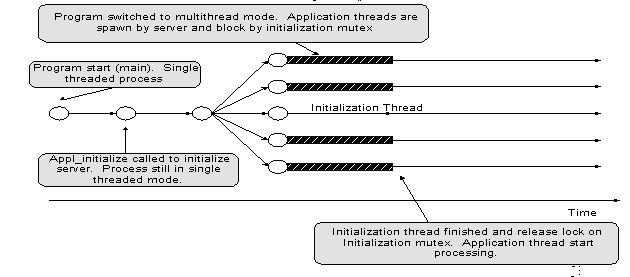
Description of "Figure C-8 Multithread Server Initialization"
SRP_initialize
Calls SRP_initialize() in appl_initialize() before other initialization routines. Add functionality that is generic to all SRP servers.
SRP_initialize() creates the following threads:
-
SARM Driver thread
-
Work Order Manager thread
-
part of event handler thread
SRP_initialize() creates the following message queues:
-
Work Order Manager
-
SARM Driver
Figure C-9 shows the components that are added to C++ SRP API server after call to SRP_initialize.
You must develop code that:
-
Interfaces with the Upstream system (receiver thread and sender thread).
-
Translates a service request in native format to CSDL (Translator thread).
-
Submits an ASAP work order containing CSDL to SARM Driver message queue.
-
Handles a provisioning event from SARM by defining the event handling function.
C++ SRP threads
The following section describes the threads in the C++ based SRP server:
-
Receiver
-
Translator
-
Event Handler
-
Sender
Receiver
The following tasks are performed by the receiver:
-
Connects to the Upstream system using the protocol agreed with upstream system.
-
Validates the connection for security purposes.
-
Waits for the work order containing the service request in the native format from upstream system.
-
Checks the dependency of work order.
-
Allocates the translator message structure, stores the work order in the native format and provides the field containing the translator return status (synchronous).
-
Submits the pointer to the translator message to the Translator message queue.
-
Waits for the return status from the translator.
-
Formats the acknowledgement message.
-
Returns the acknowledgement message to the upstream system.
-
Waits for the next request from the upstream system.
Connecting with the upstream system
This is site specific. The common protocols used are TCP/IP sockets.
Multiple concurrent connections can be accepted from the upstream system, that is, multiple instances of the receiver thread. However, the dependency between work orders submitted from different connections must be managed properly by the receiver before it is sent to the translator message queue for translation.
To benefit from multiple connections, multiple translator threads and the SARM driver must be present to obtain maximum performance.
Verifying incoming message
Verification can be performed after a formatted data string is successfully received from the upstream system. The purpose of the verification is to check if the message is in the appropriate format that is understandable by the SRP or any other restriction without delimiting the raw message.
Synchronous processing
-
The receiver thread submits the message to translator message queue with wait.
-
Translator thread wakes up the receiver thread after the work order successfully submits to SARM.
-
Translator message contains the field to store the SARM submission status. The receiver has the responsibility to deallocate the translator message structure after translator returns.
-
Receiver returns the acknowledgment to the upstream system and waits for the work order from the upstream system.
Asynchronous processing
-
Receiver thread submits the message to the translator message queue with no wait.
-
Translator thread process in parallel with the receiver thread.
-
Receiver thread returns the acknowledgement to the upstream system after it submits the message to the translator thread.
-
After the work order submits to SARM, the translator is responsible for freeing memory allocated for the translator message.
Thread examples
This section provides thread examples.
Single connection, asynchronous processing (work order dependency)
-
One Receiver thread and multiple Translator thread is used.
-
Dependent work orders are submitted to the C++ SRP API in sequence.
-
Work orders are submitted to the SARM in parallel when asynchronous processing. Child work orders might be submitted to SARM and processed by SARM before parent work orders.
-
Receiver thread maintains the work order list order by a key value that determines dependency.
-
Work order received from the upstream system will check the list if the key is found. The parent work order of the current work order is updated to the work order already in the list. Then the work order is submitted to translator.
-
When SARM returns successful provision status of the work order, the work order will be deleted from the list.
Figure C-10 Single Connection, Asynchronous Processing (Work Order Dependency)
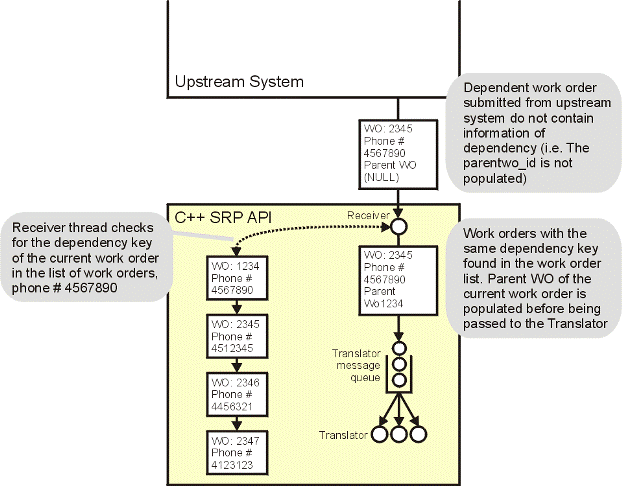
Description of "Figure C-10 Single Connection, Asynchronous Processing (Work Order Dependency)"
Single connection, synchronous processing (batch submission of work order)
-
One Receiver thread and multiple Translator thread are used.
-
Multiple work orders are submitted to the C++ SRP API in batches within one data transfer from the upstream system.
-
Receiver maintains a counter of the number of work orders and a message queue (Receiver message queue).
-
After the receiver thread submits all work orders of a batch to the Translator thread, it waits for the message from the Receiver message queue.
-
Translator thread sends a message to the Receiver message after finish submitting one work order to SARM.
-
Receiver thread decrements the work order counter when it receives one message from the translator. Once the counter reaches zero, the Receiver returns an acknowledgement message to the upstream system and waits for the next batch of work orders.
Figure C-11 Single Connection, Synchronous Processing (Batch Submission of Work Order)
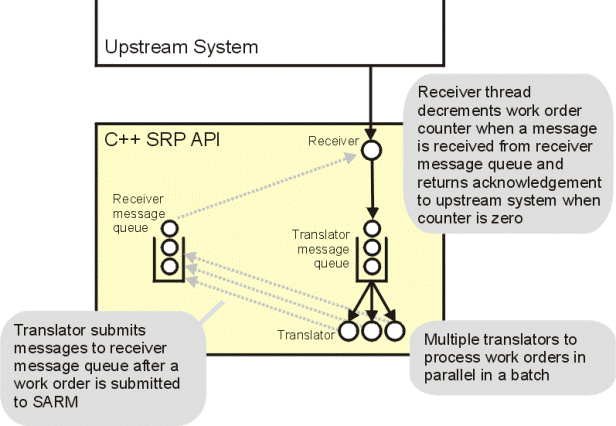
Description of "Figure C-11 Single Connection, Synchronous Processing (Batch Submission of Work Order)"
Translator
The following tasks are performed by the translator:
-
Receives the message from the translator message queue
-
Constructs the work order (intermediate structure) from the service request passed in from the upstream system.
-
Translates the work order into the ASAP work order structure and sends it to SARM.
-
Synchronous translation – Updates the return status inside the translator message, and wakes up receiver thread.
-
Asynchronous translation – Allocates to the sender message structure and sends the structure to the sender message queue asynchronously.
The following steps are required to return the translation status to the upstream system:
Table C-3 Synchronous vs asynchronous processing
Synchronous Processing | Asynchronous Processing |
---|---|
Update the return status in the Translator Message structure. |
Allocate and populate the Sender Message structure with the status of translation. |
Wake up the Receiver Thread. |
Send a message to Sender Message queue without waiting (asynchronously). |
Receiver thread will lookup the translation status in the Translator Message structure. |
Translator frees the memory allocated for the Translator Message structure. |
Receiver frees the memory allocated for the Translator Message structure. |
Translator status will send through the connection established with Sender Thread. |
Receiver will return the Translation status as ACK/NACK to the upstream system through the connection established with receiver |
- |
Event handling
This section describes event handling.
SARM events
At different stages of provisioning, SARM will notify the C++ SRP Work Order Manager thread. The Work Order Manager (handled by API), in turn, spawns threads to handle the event.
The SRP_EventManager manages a connection with SARM to receive work order events. When a SRP_EventManager thread starts up, it waits to receive a message, which is an event, from SARM. The message is constructed as a DU packet format, which is provided. The SRP_EventManager thread decodes the message and creates the SRP_Event object to the corresponding event type in the messsage. The thread calls the corresponding event handler for the event with the SRP_Event object. After the event handler is done, it returns to receive an event. If an event is the kick_start, the thread does not create a SRP_Event object. It just sends CS_TRUE back to the SARM.
The following are the possible events and the sequence of events for a work order:
-
SRP_WO_ESTIMATE_EVENT
-
WO_STARTUP_EVENT
-
WO_SOFT_ERROR_EVENT
-
WO_NE_UNKNOWN_EVENT
-
WO_ROLLBACK_EVENT
-
WO_TIMEOUT_EVENT
-
WO_FAILURE_EVENT
-
WO_COMPLETE_EVENT
The sequence of events is guaranteed for a work order. For example, a WO_FAILURE event will not occur before a WO_STARTUP event.
Actions performed by event handler
All action methods send a diagnostic message for performance testing to indicate in what is the status of the work order and then calls the appropriate RPC to update the SRP database to reflect the new conditions of the work order.
-
EventHandler::softErrorHandler().
-
EventHandler::woEstimateHandler()
-
EventHandler::woStartHandler()
-
EventHandler::woRollbackHandler()
-
EventHandler::neUnknownHandler()
-
EventHandler::woBlockHandler()
-
EventHandler::woTimeOutHandler()
-
EventHandler::neAvailHandler()
-
EventHandler::neUnavailHandler()
-
EventHandler::woAcceptHandler()
-
EventHandler::woCompleteHandler()
-
EventHandler::woFailureHandler()
Sender
Sender manages the connection with the upstream system to return provisioning information to the upstream system. The Sender thread can receive a message from an event handler or translator (asynchronous processing).
The following tasks are performed by the Sender thread:
-
Establishes the dedicated connection with the upstream system.
-
Waits for a message from sender message queue.
-
Formats the sender message.
-
Sends the message to the upstream system.
-
If the sender message comes from the event handler, it wakes up the event handler thread.
-
If the message comes from the translator, it deallocates the sender message.
Sender message
Wo_id must be part of the sender message to allow the upstream system to recognize which work order the message belongs to.
If the Translator sends a message to the Sender, all Sender Messages should include the type of message (for example, translator or event message) because the translation message is different from the event message.
C++ SRP API specification template example
The C++ SRP API Template example contains the following:
-
Support TCP/IP socket connection.
-
Choose either Asynchronous or Synchronous Translation with compile option –DASYNC or –DSYNC respectively.
-
If the static table or interpreter translation is used, the database table and function in the Translator Thread must be created and populated.
Figure C-12 Specification Template Example
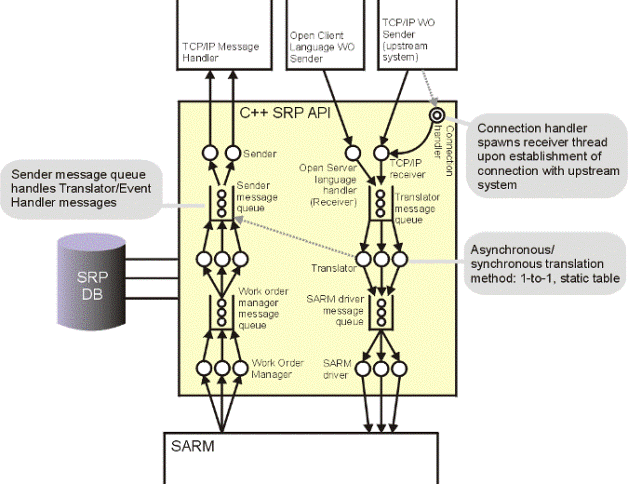
Description of "Figure C-12 Specification Template Example"
Communication interface
This section describes the communication interface.
TCP/IP socket interface
The TCP/IP socket interface contains the following:
-
Connection Handler
-
Receiver
Connection handler
-
One instance of this thread is spawned when the C++ SRP API server starts.
-
Connection Handler thread will listen for the connection from the port specified in the ASAP configuration file.
-
When the connection is detected, it can check the client connection information.
-
Spawns the Receiver thread and passes in the socket descriptor of the client connection to handle the incoming data.
-
Waits for the next connection.
Receiver
-
Spawned by the connection handler once the connection is detected.
-
Loops and does the following:
-
Reads the message header and message from the socket connected to the upstream system and populates the translator message structure.
-
If the connection drop is detected, it terminates the current receiver thread only.
-
If the connection drop is not detected, it verifies the message retrieved from the socket.
-
Table C-4 Compilation options
Compile with SYNC defined | Compile with ASYNC defined |
---|---|
Submits message to the translator synchronously. |
Submits message to the translator asynchronously. |
Waits for the translator to finish translation. |
Returns ACK/NACK to the upstream system through the socket connected to the upstream system. |
Retrieves the return status of translation from translator message. |
- |
Returns ACK/NACK to the upstream system through the socket connected to the upstream system. |
- |
Translator thread
-
Main program spawns the translator threads and creates the translator message queue when the server starts up.
-
Repeat the following:
-
Translator thread waits for the message from the receiver through translator message queue.
-
Translator parses out the service request in the native format and stores the tokens in the SRP work order structure.
-
Creates SRP_WO object base on SRP work order structure.
-
Translators submit SRP_WO to SARM using submitWO.
-
Table C-5 Compilation options
Compile with SYNC defined | Compile with ASYNC defined |
---|---|
Translator populates the translation message status passed in from the receiver thread. |
Translator constructs the sender message with translator type. |
Wakes up the receiver thread. |
Submits the sender message to the sender message queue with no waiting. |
- |
Free memory allocated for the translator message. |
Translation process
The C++ SRP API template supports translation methods. You must provide the mapping of the native service request to CSDL command.
1 to 1 mapping:
Direct mapping of the service request to the CSDL with no translation.
Static Table translation:
-
Load data in the database tables into SRP memory using the RPC (function in SRP database).
-
For each service request in SRP work order, look up the CSDL from the table in memory using bsearch.
-
For every parameter of the CSDL, search for the appropriate parameters in the SRP WO and add to the ASAP WO.
Event handling
-
Events – woStartupHandler, woCompleteHandler, woFailureHandler, etc.
-
Populates the sender message.
-
Retrieves the CSDL log from the SARM database.
-
Submits the message to the sender with wait (synchronous transfer).
-
Frees the sender message.
Sender thread
-
C++ SRP API server spawns sender threads when the server starts up.
-
Sender message can be an event message or a translation status message (if ASYNC is defined).
-
Sender will format the return message based on the message type and sends it back to the upstream system.
Event message handling
The Event sender message is submitted synchronously. Upon completion, the Sender will wakeup the event handler thread.
Upstream system
The following is the upstream system process.
WO submission
-
Program will connect to C++ SRP API server.
-
Read message files from a directory.
-
Send data in the file to C++ SRP API.
-
Print an acknowledgment message to standard output.
-
TCP/IP.