12 Creating Action Processors and Programs for Processing Requests and Responses
This chapter describes how to create action processors and Java programs for atomic actions that implement man-machine language (MML) commands for Oracle Communications ASAP.
About Action Processors and Programs
The Network Element Processor (NEP) is the ASAP server component that manages interactions with network elements (NEs) and element management systems (EMSs). The NEP receives atomic actions from the service activation request manager (SARM) and uses programs to interact with the NE. Based on the programs, the NEP sends commands to the NE and returns responses from the NE to the SARM.
The NEP must choose the correct program to fulfill the atomic action. To determine which program to use, the NEP uses action processors. Action processors map atomic actions to programs. When you create ASAP cartridges, you can write Java programs from scratch, or configure the action processor to auto-generate Java programs.
This chapter describes the following:
-
How to create and configure action processors.
-
How to auto-generate Java command line interface (CLI) code and the situations where you need to write custom business logic. Auto-generating CLI code is available for cartridges that use CLI commands, such as TL1 over TCP/IP and Telnet over TCP/IP.
-
How to auto-generate Java code stubs and the places where you need to write custom business logic. Auto-generating a Java stub is available for any cartridge type.
-
Recommendations for writing Java programs from scratch.
-
How to auto-generate unit test cases and the places where you need to write custom business logic.
Note:
While you can write a Java implementation, Oracle recommends that you auto-generate Java stubs. This method provides code that you would normally have to write yourself.
When the NEP receives an atomic action and its parameters from the SARM, the NEP determines what program to run based on the SARM tbl_nep_asdl_prog table. This table contains the mappings between atomic actions and programs that is defined in the action processor. The table defines the following columns:
-
asdl_cmd: The atomic action passed to the NEP interpreter or jinterpreter.
-
tech: The technology type of the NE that the NEP interpreter or jintepreter interacts with. With Java programs, the values in this column are a combination of vendor and technology separated by a dash (for example, ALU-FTTU).
-
sftwr_load: The software version of the software currently running on the NE.
-
program: The Java program that the jinterpreter must run to fulfill the atomic action.
-
interpreter_type: A value of J indicates a Java program.
Table 12-1 shows how the same atomic action can map to various vendors, technologies, and software versions.
Table 12-1 Atomic-Action-to-Program Mappings
asdl_cmd | tech | sftwr_load | program | interpreter_type |
---|---|---|---|---|
CLEAR_INTERCEPT |
ALU-DMS |
BCS35 |
com.alu.dms.bcs35.ClearInterceptProxy.execute |
J |
CREATE_LINE |
ALU-DMS |
BCS35 |
com.alu.dms.bcs35.CreateLineProxy.execute |
J |
SET_OPTION_ON |
ALU-DMS |
BCS35 |
com.alu.dms.bcs35.SetOptionOnProxy.execute |
J |
CLEAR_INTERCEPT |
CSCO-INV |
1.0 |
com.csco.inv.1_0.ClearInterceptProxy.execute |
J |
CREATE_LINE |
CSCO-INV |
1.0 |
com.csco.inv.1_0.CreateLineProxy.execute |
J |
SET_OPTION_ON |
CSCO-INV |
1.0 |
com.csco.inv.1_0.SetOptionOnProxy.execute |
J |
For more information about the NEP and the JInterpreter, see ASAP Server Configuration Guide. For more information about tbl_nep_asdl_prog, see ASAP Developer's Guide.
About the Ratio of Provisioning Commands to Atomic Actions
Whenever possible, map each atomic action to a program containing only one provisioning command. As a general rule, the fewer commands associated with the atomic action, the easier it is to use the atomic action as a building block in the implementation of higher level services. However, in some less common scenarios, several actions must be run on the NE in sequence. In such cases, you map a single atomic action to more than one action.
For example, NEs that require certain modes to be set before a provisioning command can be sent to the NE may need to encapsulate the commands to set the modes along with the provisioning command.
Review the following considerations before deciding whether to encapsulate several commands:
-
Determine whether encapsulating the mode commands substantially increases ASAP and router performance.
-
Determine whether the service model becomes less complicated when commands are encapsulated. Reducing service model complexity allows the service modeler to focus on implementing service offerings rather than on understanding and modeling mode setting dependencies for every service.
-
Determine whether encapsulating mode commands removes the need to have complex mutex logic within the cartridge. For example, multiple ASAP work orders destined to the same NE may result in interleaved atomic actions. In some devices, without implementing mutex logic, atomic actions fail because the router is in an indeterminate mode for any given atomic action.
-
Determine whether encapsulating mode commands removes the for implement additional logic for connection handlers. For example, if each atomic action sets its own mode, when a connection to a router is lost, at any point the connection handler would not have to determine whether any mode setting commands must be re-run.
For additional considerations at the service action level, see "About Limiting Independent Network Element Commands to Optimizing the Network Element Interface."
About Creating and Configuring Action Processors
An action processor maps an atomic action to a Java program. For every action processor, you need to define a program as the implementation that performs the work.
The naming convention for action processors is the same as the naming convention for atomic actions, with the exception of the prefix: Action processors use the prefix I whereas atomic actions use the prefix A. See "About Creating and Configuring Atomic Actions" for information about the naming convention.
Design Studio for ASAP automatically enforces this naming convention when you create an action processor with the Action Processor Wizard.
Creating an Action Processor
To create an action processor:
-
From an Activation project, select Studio, then select New, then Activation, and then Action Processor.
-
From the Action Processor Wizard, enter the following:
-
From the Project list, select a cartridge project in which to create the action processor.
-
In the Action field, the name of the action that the action processor performs (see "Selecting the Action Tokens").
-
In the Entity field, enter an entity that is the object of the action (see "Selecting Entity Tokens").
-
-
Click Finish.
The Action Processor editor appears. From the action processor, you can either auto-generate code or associate the action processor with code that you have written yourself. For more information about these options, see:
Understanding the Auto-Generated Java CLI Code
After you create the action processor, you can auto-generate the CLI Java code. You auto-generate the code when you want ASAP to interact with NEs that use CLI-based commands such as TL1 over TCP/IP or Telnet over TCP/IP. The code that is generated sends requests. You can include additional post processing logic if required. You must add business logic to the generated code for receiving responses.
Note:
The CLI code generation option is available for cartridges created or upgraded to ASAP 7.3.2 or later releases. If you are designing a cartridge that is not CLI-based, see "Understanding the Auto-Generated Java Code Stubs."
Before you can auto-generate CLI Java code, you must have created and configured the atomic action associated to the action processor. See "Creating and Configuring Atomic Actions" for instructions.
To auto-generate CLI Java code, you perform the following tasks:
-
Configure the default command structure of the CLI commands that ASAP sends to the NE. For more information, see "About the CLI Command Structure Elements."
-
The action processor editor Request tab. This tab defines the CLI commands that ASAP builds and sends to the NE. You can parse sample CLI commands to generate the elements names that are part of the outgoing command or manually add the command parameters. You can map these command parameters to atomic action parameters or define them as static parameters. For more information, see "Configuring CLI Command Requests."
-
The action processor editor Response tab. This tab defines a response pattern and the position of the value within the response pattern that matches with an ASAP user exit type. You must also add response handling logic to the generated Java code. For more information, see "About Configuring CLI Command Responses."
Consider the following restrictions when choosing to auto-generate CLI Java code:
-
The auto-generated code applies to network cartridges only; not to service cartridges.
-
The auto-generated code supports only a one-to-one mapping ratio between the action processor and CLI command.
-
If you want to use the CLI code generation function in cartridges developed before Design Studio 7.3, you must delete the old action processor and generate a new action processor. You must manually copy over any descriptive information contained in the old action processor.
About Configuring the CLI Command Structure
After you create an activation project, you can define the Java CLI command structure. The command structure defines the delimiters to use in CLI commands. You define a general command structure for request commands. When you configure the request commands, you can overwrite the general structure if a command you're configuring requires a different structure.
You must enable auto-parsing of CLI commands if you want Design Studio to automatically parse and map CLI command parameters to atomic action parameters when you configure your Java CLI command requests.
When automatically parsed, the command parameters are mapped to the atomic action parameters that have the same parameter names. If a command parameter name does not match any atomic action parameter name, you must manually map that command parameter to an action parameter.
About the CLI Command Structure Elements
After you create an activation project, you can define the CLI command structure. The command structure can have one or more of the following elements:
-
Header Body Separator: Defines the separator between the header and the rest of the CLI command.
-
Parameter Separator: Defines the separator between parameters within the CLI command.
-
Parameter Name-Value Separator: Defines the separator between a parameter and its value.
-
Compound Parameter Encloser: Defines the separator that encloses compound parameters.
-
Compound Parameter Index Separator: Defines the separator between members of a compound parameter.
-
Command Tail: Defines the character at the end of the CLI command.
-
End of Command Control Character: Defines the command control character or string at the end of the CLI command. For example, a carriage return or a line-feed character.
Each command structure elements can take one of the following values:
-
NONE: Select this value if the CLI command does not use the element.
-
: COLON: Select this value if the element should be a colon.
-
, COMMA: Select this value if the element should be a comma.
-
. DOT: Select this value if the element should be a period.
-
= EQUAL: Select this value if the element should be the equals sign.
-
; SEMI_COLON: Select this value if the element should be a semicolon.
-
SPACE: Select this value if the element should be a space.
-
CARRIAGE: Select this value if the element should be a carriage return.
-
NEW LINE: Select this value if the element should be a new line.
-
Ctrl+C: Select this value if the element should be the Ctrl+C key combination.
-
OTHER: Select this value if the element requires one or more characters not specified in this list. When you select OTHER, a field appears next to the list in which you enter one or more special characters. For example, if the End of Command Control Character is the word COMMIT, you could specify this using the OTHER option.
Configuring the CLI Command Structure
To configure the Java CLI command structure:
-
Open the activation project editor.
-
Click the Command Structure tab.
-
For each command structure element, do the following:
-
From the Header Body Separator list, select the command structure element.
-
From the Parameter Separator list, select the command structure element.
-
From the Parameter Name-Value Separator list, select the command structure element.
-
From the Compound Parameter Encloser list, select the command structure element.
-
From the Compound Parameter Index Separator list, select the command structure element.
-
From the Command Tail list, select the command structure element.
-
From the End of Command Control Character list, select the command structure element.
-
Click OK.
-
-
Enable the Command Auto-Parsing check box if you want to enable the Command Auto-Parse Override check box for all new action processors in the action processor editor Request tab.
-
Save the changes.
About Parsing and Configuring CLI Command Requests
Parsing Java CLI command parameters extracts the parameters from a sample CLI command and maps the parameters to corresponding atomic action parameters. You parse command parameters when you configure your CLI command requests. When you parse and configure CLI command requests, you can do the following:
-
Manually parse the parameters or specify to automatically parse the parameters.
-
Add logic that calls a helper method that manipulates atomic action parameters for name-value parameters or value-only parameters. Helper methods are not used for static string parameters because those parameters are not mapped to atomic action parameters.
You can use the helper methods defined in these files:
-
Utils.java: This file contains predefined methods. See "Provided Methods for Manipulating Parameters" for more information.
-
ReusableMethods.java: You can define your own custom helper methods in this file. See "Defining Custom Methods for Manipulating Parameters" for more information.
-
Provided Methods for Manipulating Parameters
The Utils.java file contains helper methods that you can use to manipulate atomic action parameters and values. The methods include tasks such as appending characters to a parameter, concatenating two or more parameters, and so on. Design Studio generates the Ultils.java file when you configure your request CLI commands. You can access this file from the Design Studio Package Explorer view in the src directory.
All methods defined in Utils.java throw exceptions defined in the ProvCartridgeException.java file, which is auto-generated when you add a sample command.
Table 12-2 describes the methods contained in Utils.java and provides examples. For more information about using the methods, open the Utils.java file in the Package Explorer view. You can access this file after entering a CLI command to parse when configuring your CLI command requests.
Table 12-2 Utils.java Methods
Method | Description | Example |
---|---|---|
append |
This method appends a value to a parameter. |
The following appends the hash character to the MSISDN parameter: append(MSISDN,"#") |
concat |
This method concatenates multiple parameters together with a specified delimiter. The first value specifies the delimiter. You can specify multiple parameters after the delimiter using the Java variable arguments feature. The method returns a string after concatenating all the parameters passed. For example, you can use this method when you have to join two atomic action parameters into one parameter in the CLI command. |
The following concatenates the MSISDN and LCC_CODE parameters using a dash delimiter: concat("-","MSISDN","LCC_CODE") |
encloseWith |
This method encloses a parameter between two strings. |
The following encloses the MSISDN parameter between two hash characters: encloseWith(MSISDN,"#","#") |
fixedLength |
This method ensures that a parameter value is of a fixed number of characters. The method takes the required length of the parameter, the character to be used as padding, and the mapped parameter. If appender is true, the padding characters are prefixed otherwise it is suffixed. The method returns a string with a length equal to the length passed by prefixing or suffixing the padded character to the value passed. |
The following appends the * padding character to the MSISDN parameter value if its value length is less than 5. Because the boolean flag is set to true, the padding character is added to the beginning of the value: fixedLength("MSISDN",MSISDN,5, '*', true) |
prepend |
This method adds a value to the beginning of a parameter. |
The following adds the hash character to the beginning of the MSISDN parameter: prepend(MSISDN,"#") |
replaceWith |
This method replaces all occurrences of a string in the parameter value with a new string. |
The following replaces dashes with hash characters within the MSISDN parameter value: replaceWith(MSISDN,"--", "##") |
substring |
This method extracts a substring from a parameter value. The substring is identified by its start and end character positions in the value. For example, if the value of MSDN were ABCDE and the start position is 3 and end position is 5, then the return value is CDE. |
The following extracts the 3rd, 4th, and 5th characters from the value of the MSISDN parameter: substring(MSISDN,3,5) |
translate |
This method translates a parameter value to a specified value. You specify the translation using value pairs separated by an equals sign (=). Delimit each pair with a comma (,). For example if an upstream system sends "Y" or "N", and the NE expects "Yes" or "No", you can translate the incoming values using "Y=Yes, N=No". This method returns the new value. If the method syntax is incorrect, the method returns null. |
The following takes the ACTIVATE parameter value from the atomic action and translates the value from Y to Yes or from N to No.: translate(ACTIVATE,"Y=Yes,N=No") |
Defining Custom Methods for Manipulating Parameters
When you configure you Java CLI command requests, you can add logic that calls a helper method to manipulate atomic action name-value parameters or value-only parameters. If you need helper methods other than those defined in the Utils.java file, you can define your own in the ReusableMethods.java file. (For information about the methods in the Utils.java file, see "Provided Methods for Manipulating Parameters.") Design Studio generates the ReusableMethods.java file when you configure your request CLI commands. You can access this file from the Design Studio Package Explorer view in the src directory.
The methods you define in ReusableMethods.java must throw exceptions defined in the ProvCartridgeException.java file.
Configuring CLI Command Requests
To configure a CLI command request:
-
Open the action processor editor and click the Editor tab.
-
From the Type list, select CLI Code Generation.
The Request and Response tabs appears.
-
Click the Request tab.
-
If the CLI command you want to configure does not conform to the command structure you previously configured (in "About the CLI Command Structure Elements"), select Overwrite in Separators area and configure the command structure you need. Do the following:
-
From the Header Body Separator list, select the command structure element.
-
From the Parameter Separator list, select the command structure element.
-
From the Parameter Name-Value Separator list, select the command structure element.
-
From the Compound Parameter Encloser list, select the command structure element.
-
From the Compound Parameter Index Separator list, select the command structure element.
-
From the Command Tail list, select the command structure element.
-
From the End of Command Control Character list, select the command structure element.
See "About Configuring the CLI Command Structure" for information about the structure elements and their values.
-
-
In the Input Command field, enter a sample CLI command to use as a template for defining the action processor parameters. The sample command should be a typical CLI command that you want ASAP to send to NEs to fulfill the atomic action that the action processor is associated with.
-
Parse the command and map the command parameters to action parameters by doing one of the following:
Tip:
Preview the structure of the command in the Preview area as you map CLI command elements to atomic action parameters.
For example, the following command shows a header called HSDPA, a value-only parameter, two value-pair parameters, two static parameters, and ends with the COMMIT control character:
HSDPA:<mcliVal>,LCC_CODE=<user_routingVal>,LINE=<lineVal>,static, program,;COMMIT
To automatically parse the CLI command:
-
Select the Command Auto-Parsing check box.
The Parse Input Command button becomes selectable.
-
Click the Parse Input Command button.
The command parameters are parsed based on the parameter structure separators you configured. The parameters appear in the Parameters area in the Element Name list.
If the parameter name does not match any atomic action parameter, then the parameter is still added to the Element Name list, but does not map to any atomic action parameter in the Maps To field.
-
If all parameter names are mapped to atomic action parameters, go to step 7.
-
If some parameters are not mapped to atomic action parameters, go to the instructions for manually parsing the CLI command below.
To manually parse the sample CLI command:
-
Using your mouse, highlight and right-click on a parameter in the command.
-
If you want to designate the parameter as the command header, select Command Header.
The parameter appears in the Command Header field.
-
If you want to designate the parameter as a command parameter, select Command Parameter, then select the value type to use in the command:
-
Name Value Pair: When you select this option, the parameter name and value are used. You must select a parameter from the atomic action from which the value is populated.
-
ValueOnly: When you select this option, only the parameter value is used. You must select a parameter from the atomic action from which the value is populated.
-
StaticString: When you select this option, only the parameter name is used. Static strings do not contain values and cannot be associated with atomic action parameters.
After you make your selection, the highlighted parameter appears in the Element Name list. When you select one of the auto-generated parameters from the Element Name list, the associated atomic action parameters appear in the Maps To field (except for StaticString parameters).
-
-
-
(Optional) For Name Value Pair and ValueOnly parameters, add a line of logic in the Parameter Logic field that calls a helper method that manipulates the atomic action parameter and click OK.
You can use the helper methods defined in either the Utils.java file (see "Provided Methods for Manipulating Parameters" or the ReusableMethods.java file (see "Defining Custom Methods for Manipulating Parameters."
You can nest two or more commands in the Parameter Logic field. For example, the following line nests the encloseWith method within the concat method:
concat("*",encloseWith(MCLI,"#","#"),MY_TEST)
-
If you need to add more complex logic for a particular parameter that can be enabled by a one line method as in the Parameter Logic field, click Edit Parameter Logic. The first time you click this button Design Studio generates additional java files where you can make these modifications. If you make changes to the command parameter to atomic action parameter mappings after you have clicked the Edit Parameter Logic button for the first time, new files are not generated if you click the Edit Parameter Logic button again. For more information about the files generated when you click the Edit Parameter Logic button, see "About Auto-Generated and Synchronized CLI Java Files."
About Configuring CLI Command Responses
You must write additional code for handling CLI command responses. Design Studio generates the ResponseHandlerImplementation.java file when you configure your CLI commands. You can access this file from the Design Studio Package Explorer view in the src directory.
You can also configure the action processor to search for a specific response snippet in response messages before sending the response to the user defined exit type code you have written.
Configuring CLI Command Responses
To configure a CLI command response:
-
Open the action processor editor and click the Editor tab.
-
From the Type list, select CLI Code Generation.
The Request and Response tabs appears.
-
Click the Response tab.
-
In the Response Section, Response area, Response field, enter a description of the response.
-
If you want the action processor to search for responses with specific headers or exit type patterns before sending the response to the user exit type code, do the following:
-
In the Response Section, Exit Type, Response Header field, enter a response header.
-
In the Response Section, Exit Type, Exit Type Pattern field, enter a response pattern.
-
Click Mark Positions button.
The Mark Positions Specifier dialog appears.
-
In the Start Position field, enter a starting position from 1 to 10. The start position must be less than the end position.
-
In the End Position field, enter an end position from 1 to 10. The end position must be greater than the start position.
-
-
Click Edit Response Logic. The ResponseHanderlImplementation.java file opens in the Package Explorer view.
-
Add response handling code to the file. For more information about the ResponseHanderlImplementation.java file, see "Auto-Generating the Java CLI Files."
Auto-Generating the Java CLI Files
To auto-generate the Java CLI code files:
-
Open the action processor editor and select the Editor tab.
-
From the Type list, select CLI Code Generation.
-
Click New.
Design Studio automatically generates Java code.
The Class field points to the auto-generated proxy java file (for example, alu.fttu.x74.ont.add.generated.AddOntProxy). This file contains a proxy class that is situated between the NEP and action processor and manages the interaction between them.
The Method field points to the execute method within the processor java file (for example alu.fttu.x74.action.ont.add.AddOntProcessor.java).
For more information about the auto-generated Java files and code and the areas where you must include additional business logic, see "About Auto-Generated and Synchronized CLI Java Files."
-
Click Finish.
About Auto-Generated and Synchronized CLI Java Files
After the Java files are generated, Design Studio automatically updates those files that are synchronized whenever you build the cartridge. Never make changes to synchronized files because Design Studio overwrites these files when you build the cartridge. You can, however, modify files that are not synchronized.
Oracle recommends that you backup the cli_project/src directory (where cli_project is the activation project that contains the CLI code) to a source control system. This directory contains all the modifiable CLI Java files. For more information about backing up this folder, see Developer's Guide.
Table 12-3 describes the Java files that are created when you auto-generated them, and whether the generated files are synchronized and modifiable.
Note:
In the files specified in Table 12-3, action and entity represent the action and entity values you selected when you created the action processor (see "Creating an Action Processor").
Table 12-3 CLI Code Generation Java Files
Java File | Description | Sync | Mod |
---|---|---|---|
ActionEntityProcessor.java |
This file contains the ActionEntityProcessor class that implements the generated processor interface. The ActionEntityProcessor class includes the execute class and the following methods:
The logic in the execute class is fully functional when you auto-generate the CLI Java files. The code is generated based on the command element to atomic action mappings that you configured using the action processor editor Request tab. The code is also based on the Exit Type Pattern field in the action processor editor, Response tab. You specify a response snippet in this field that the code searches for in response messages. You use the Mark Positions button to specify what part of the response snippet to verify before sending the response to the user defined exit type code you have written. For more information about exit types, see "Creating Java User Exit Types." The ActionEntityProcessor.java file is auto-generated when you click the New button as described in "Auto-Generating the Java CLI Files." However, if the file already exists when you click the New button, then the file will not be auto-generated and will not reflect any changes that you made to the action processor since you first auto-generated the file. If you want to Design Studio to auto-generate the file, you must delete the old file first. |
No |
Yes |
ModifyGeneratedMML.java |
This file contains methods you can use to augment the generated MML command if you require any additional post processing. For example, you may need to encrypt the CLI command before sending it. |
No |
Yes |
ResponseHandlerImplementation.java |
This file contains the ResponseHandlerImplementation class that implements the ResponseHandlerInterface. You must do the following in this file:
|
No |
Yes |
ActionEntityInput.java |
This file contains the InputBean. The InputBean has set and get methods for all parameters of the atomic action and provides setters and getters for manipulating parameters. If the parameter is a scaler (simple type), it is received as a string and can be used immediately. For information about compound parameters, see "Understanding Generated Code for Compound Parameters" If you need to add logic to the input parameters, for example, if you are mapping a CLI command to more than one compound parameter, you can click the Edit Parameter Logic button in the action processor editor Request tab to generate files where you can add this logic. For more information about these generated files, see Table 12-4. |
Yes |
No |
ActionEntityOutput.java |
This file contains the Output class that enables you to populate output parameters. There are convenience methods for populating parameters to varying scope within a work order. Examples of parameters are as follows:
The output parameters are not explicitly defined in the model, so there are no convenience methods. To set a parameter, you need to know its string name and include it. |
Yes |
No |
ActionEntityProcessorInterface.java |
This file contains the processor interface that is implemented by the ActionEntityProcessor class. This interface is synchronized whenever the cartridge model changes so the ActionEntityProcessor class always has the correct cartridge data available. |
Yes |
No |
ActionEntityProxy.java |
This file contains the Proxy that is situated between the NEP and Processor class and manages the interaction between them. Proxy sets up all classes used by the processor and initiates and calls the processor. Most importantly, the proxy simplifies the work required by the Processor by:
When creating a Java processor from the action processor editor, the resulting class name is "Proxy" because the proxy gets initiated by the NEP (the Proxy is registered to be called in the activation). |
Yes |
No |
ConnectionHandler.java |
This file contains the ConnectionHandler class that extends a protocol class and implements the IConnectionHandler. IConnectionHandler is an instance of the connection handler that is associated with the vendor, technology, and software version of the action processor. For the Telnet connection handler, the basic methods on the interface can be used to send requests (because it is string-based). For technologies (for example, SOAP or XML) that provide multiple convenience methods, the processor may test the type of connection handler and pass the request to a more specific connection handler to obtain access to the convenience methods. If you want to expose more explicit methods when writing a connection handler, you can define an interface that extends IConnectionHandler and ensure that those methods are available through that interface. The processor should always use an interface when interacting with the ConnectionHandler, to achieve the implementation in more than one way and allow for unit testing. For more information about unit testing, see "Understanding Unit Testing." |
No |
Yes |
BaseActionEntityTestCase.java |
This file contains the unit test case for the action processor. For more information about this generated file, see "Understanding Unit Testing." |
Yes |
No |
MMLConstructor.java |
This file contains the code that builds the CLI command based on the command structure. For information about the command structure, see "About the CLI Command Structure Elements." The code does the following:
|
Yes |
No |
MMLConstructor.Interface.java |
This file provides the methods that the MML Constructor class implements. |
Yes |
No |
ResponseHandlerInterface.java |
This file contains the interface code that ResponseHandlerImplementation.java implements. |
Yes |
Yes |
Separators.java |
This file contains the command separators you specified when configuring the command structure. For more information about configuring the command structure, see "About the CLI Command Structure Elements." |
Yes |
No |
ISystemParameters.java |
This file contains the interface that extends the IBaseSystemParameters interface. The methods in IBaseSystemParameters class are implemented by the SystemParameter class. |
Yes |
No |
SystemParameters.java |
This file contains the SystemParameter class that implements the methods in the IBaseSystemParameters interface, such as getWorkOrderId(), getActionName(), and so on. The SystemParameter class extends the BaseSystemParameters class. |
Yes |
No |
Table 12-4 describes the Java files that Design Studio creates when perform the tasks required to auto-generate Java CLI code.
Note:
The files generated when using the Parameter Logic field or the Edit Parameter Logic field specified in Table 12-4 use the ElementName variable. ElementName is the parameter name in the Element Name list when the parameter is a Name Value Pair (for example ID_ROUTING.java). ElementName is a combination of the atomic action parameter in the Maps To field followed by an underscore and the parameter name in the Element Name list when the parameter is ValueOnly (for example, MCLIValue_LINE.java).
Note:
The files generated when using the Parameter Logic field or the Edit Parameter Logic field specified in Table 12-4 that are not synchronized will not reflect any changes made to the mappings between the command parameters and the atomic action parameters after you generate these files for the first time. You must delete these files manually if you want to auto-generate them again.
Table 12-4 Parameter Logic, Edit Parameter Logic, and Input Command Java Files
Java Files | Description | Sync | Mod |
---|---|---|---|
ProvCartridgeException.java |
This file contains the exception class used by the methods defined in the Utils.java file. |
Yes |
No |
ReusableMethods.Java |
This file contains the ReusableMethods class. You can use this file to define one-line methods that you can use when configuring your CLI command requests (see "Configuring CLI Command Requests"). This class should use the exception logic defined in ProvCartridgeException.java. |
No |
Yes |
Utils.java |
This file contains predefined one-line methods that you can use in the action processor editor Request tab Parameter Logic field. For more information about these methods, see "Provided Methods for Manipulating Parameters." |
Yes |
No |
ElementName.java |
This file is generated when you add a one-line method to the parameter logic when configuring your CLI command requests (see "Configuring CLI Command Requests"). |
Yes |
No |
ElementName_Implementation.java |
This file is generated when you modify the parameter logic that you specified when configuring your CLI command requests. You can add custom logic to this file if you need to include more complicated processing instructions than those in Utils.java or in ResuableMethods.java. |
No |
Yes |
ElementName_Interface.java |
This file defines an interface for the ElementName_Implementation class in the ElementName_Implementation.java file. This file is generated when you modify the parameter logic that you specified when configuring your CLI command requests. |
Yes |
No |
Understanding the Auto-Generated Java Code Stubs
You can use the action processor to auto-generate Java files with classes and methods configured for the protocol and attributes you selected. The auto-generated code are code stubs that provides a logical framework where you must include your business logic for sending and receiving responses.
The Java with code generation implementation for an action processor creates a Java processor that composes messages to be sent to a device, evaluates the response for errors, extracts output information from the response, and populates the information into output parameters.
Figure 12-1 shows some of the auto-generated files, whether they are synchronized, and how they relate to each other.
Figure 12-1 Generated and Synchronized Java Files
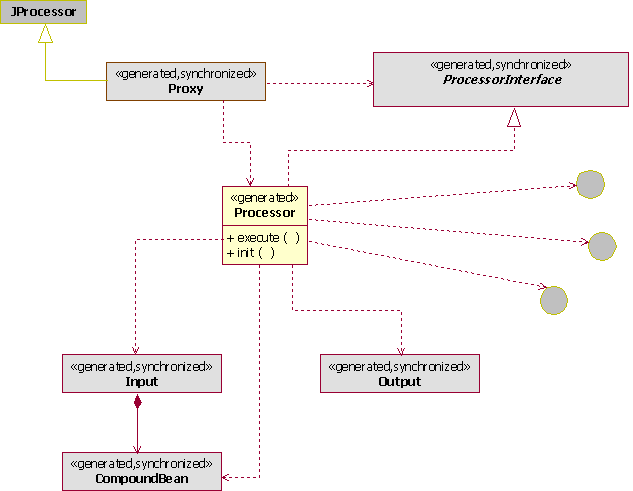
Description of "Figure 12-1 Generated and Synchronized Java Files"
When you configure the action processor to auto-generate Java code stubs, the central class is the Processor. You must add business logic to this class. The Processor is created only once. The processor includes sample code based on the associated atomic action parameters at creation time. You should delay creating the processor until the action processor is associated with an atomic action that has fully defined parameters. If parameters are not yet defined or the action processor is not yet associated with the atomic action, then the generated sample code will be incomplete and will require additional coding.
Note:
Synchronized classes or interfaces are rebuilt every time you save changes to atomic action parameters (for example, classes and interfaces are synchronized with the model and reflect the model). Therefore, you should never make code changes to any synchronized class or interface. Design Studio overwrites the code when you run the next build (with changes in the model). You should write code only for the Processor class.
There are 2 methods in the Processor:
-
execute
-
init
The main method is execute
. When called, it is provided with the following:
-
A number of classes to perform operations.
-
An input class that contains all input parameters.
-
An output class to populate the output parameters.
-
Access to a logger.
-
An implementation of the exit type to match responses against user-defined exit types and to set the exit type for the processor.
-
Access to the Connection Handler to send requests and get responses from a connected device.
Auto-Generating the Java Stubs
To auto-generate Java stubs:
-
Open the action processor editor and select the Editor tab.
-
From the Type list, select Java Action Processor (with Code Generation).
-
If you have not already done so, create and fully configure an atomic action that includes the required parameters. You must associate the atomic action to this action processor. For more information about creating and configuring an atomic action, see "Creating and Configuring an Atomic Action."
Note:
If you do not create and configure the atomic action the auto-generated code will not function properly.
-
Click New.
The Studio Activation Java Implementation Wizard appears.
-
In the Package field, enter a valid package name. You can use the default package name or enter a name of your choice. The default Package name uses the vendor, technology, software version, entity, and action that you selected when creating the action processor (see step 2).
For example:
alu.fttu.x74.ont.add
-
In the Name field, enter a name that appears in many of the auto-generated Java files and the classes they contain. You can use the default name or enter a name of your choice. The default name is a combination of the action and the entity.
For example:
AddOnt
-
Click Finish.
Design Studio automatically generates Java code.
The Method field points to the auto-generated proxy java file (for example, alu.fttu.x74.ont.add.generated.AddOntProxy). This file contains a proxy class that is situated between the NEP and Processor class and manages the interaction between them.
The Class field points to the execute method within the processor java file (for example alu.fttu.x74.action.ont.add.AddOntProcessor.java). You must add business logic to this class.
For more information about the auto-generated Java code and where you must include business logic, see "About Auto-Generated Java Files."
About Auto-Generated Java Files
Table 12-5 shows the Java files containing the classes and interfaces used by the Processor.
Table 12-5 Auto-Generated Java Files
Java Files | Description | Sync | Mod |
---|---|---|---|
ActionEntityProcessor.java |
This file contains the ActionEntityProcessor class that implements the generated and synchronized processor interface. The ActionEntityProcessor class includes the execute class with following methods:
You need to write the logic in the When implementing the action processor, Design Studio provides you with support such as auto-generation of code and sample data. In Design Studio, this is currently set up specifically for the Telnet protocol (Soap, CORBA, and other protocols require more coding; for example, you must write your own logic for send methods, requests, to extend the connection class, and so on.). Code for the processor is auto-generated by the proxy (getter and setter methods for each parameter) which provides you with an API to manipulate the data. For example, for an incoming object, methods such as To obtain the required method to get a value for a parameter, type the name of the parameter followed by a dot. This displays all available methods for the parameter. |
No |
No |
ActionEntityInput.java |
This file contains the InputBean. The InputBean is tied to the parameters of the atomic action (has set and get methods for all parameters of the atomic action), and provides setters and getters for manipulating parameters. If the parameter is a scaler (simple type), it is received as a string and can be used immediately If the parameter is a compound, see "Understanding Generated Code for Compound Parameters." |
Yes |
No |
ActionEntityOutput.java |
The Output class enables you to populate output parameters. There are convenience methods for populating parameters to varying scope within a work order. Examples of parameters are as follows:
The output parameters are not explicitly defined in the model, so there are no convenience methods. To set a parameter you need to know its string name and include it. |
Yes |
No |
ActionEntityProcessorInterface.java |
The processor interface is implemented by the ActionEntityProcessor class. This interface is synchronized whenever the cartridge model changes. |
Yes |
No |
ActionEntityProxy.java |
When creating a Java processor from the action processor editor, the resulting class name is "Proxy" because the proxy gets initiated by the NEP (Proxy is registered to be called in the activation). When you open that implementation it opens to the |
Yes |
Yes |
ConnectionHandler.java |
This file contains the ConnectionHandler class that extends a protocol class and implements the IConnectionHandler. IConnectionHandler is an instance of the Connection Handler that is associated with the vendor, technology, and software load of the action processor. For the Telnet Connection Handler, the basic methods on the interface can be used to send requests (because it is string-based). For technologies (for example, SOAP or XML) that provide multiple convenience methods, the Processor may want to test the type of Connection Handler and pass it to a more specific Connection Handler to obtain access to the convenience methods. If you want to expose more explicit methods when writing a Connection Handler, you can define an interface that extends the |
No |
Yes |
BaseActionEntityTestCase.java |
This file contains the unit test case for the action processor. For more information about this generated file, see "Understanding Unit Testing." |
Yes |
No |
ISystemParameters.java |
This file contains the interface which extends the IBaseSystemParameters interface. Those functions within IBaseSystemParameters class are implemented by SystemParameter class. |
Yes |
No |
SystemParameters.java |
This file contains the the SystemParameter class that implements the respective functions of IBaseSystemParameters interface such as getWorkOrderId(), getActionName(), and so on, by extending BaseSystemParameters class. |
Yes |
No |
Understanding Generated Code for Compound Parameters
The InputBean returns another bean that represents the compound if the parameter is a compound parameter with named members,. The returned bean has convenience methods to get the members within the compound. A compound bean for every defined type of compound parameter is created. You also get a set of instances of these beans based on the work order (you get a list of these). If the compound parameter does not have named members, it provides a vector of the members.
Note:
Specify the compound members whenever possible. Indicating the members will simplify the coding required and eliminate possible code to mode synchronization issues.
Multi-instance Compound parameters start at index one (e.g. CMPD[1]).
Bracket type (Index Parameter Identification Tokens) and delimiter (Indexed Parameter Delimiter) settings are configured on the Project editor Cartridge Locations tab in the Code Generation area. Design Studio applies these settings to all generated code within the cartridge. The following examples assume the defaults (square brackets with a period delimiter).
The following example shows a scalar parameter.
Service Action Parameter Name: SCALAR Atomic Action Parameter Name: SCALAR Order Format: SCALAR Usage: String myscalar = parms.getMyScalar();
The following example shows a compound parameter with no members specified.
Service Action Parameter Name: CMPD Atomic Action Parameter Name: CMPD Order Format: Entries will have the compound name as a prefix. There may be multiple entries with that prefix. For example, a compound named "CMPD" may have the following entries on an order. CMPD CMPD.X CMPD.Y CMPD.Z Usage: String mycmpd = parms.getMyCmpd String x = parms.getMyCmpd ("X"); String y = parms.getMyCmpd ("Y"); String z = parms.getMyCmpd ("Z");
The following example shows a compound parameter with members.
Service Action Parameter Name: CMPDMBR Atomic Action Parameter Name: CMPDMBR Order Format: CMPDMBR.A CMPDMBR.B CMPDMBR.C Usage: MyCmdMbrBean mycmpdmbr = parms.getMyCmpdMbr(); mycmpdmbr.getA(); mycmpdmbr.getB(); mycmpdmbr.getC();
The following example shows a multi-instance compound parameter with no members specified.
Service Action Parameter Name: CMPDMULTI Atomic Action Parameter Name: CMPDMULTI Order Format: Entries will have the compound name as a prefix. There may be multiple entries with that prefix. For example, a compound named "CMPDMULTI" may have the following entries on an order. CMPDMULTI[1] CMPDMULTI[1].X CMPDMULTI[1].Y CMPDMULTI[1].Z CMPDMULTI[2].X CMPDMULTI[2].Y CMPDMULTI[2].Z Usage: String mycmpdmulti = parms.getMyCmpdMulti (); String x1 = parms.getMyCmpdMulti (1, "X"); String y1 = parms.getMyCmpdMulti (1, "Y"); String z1 = parms.getMyCmpdMulti (1, "Z"); String x2 = parms.getMyCmpdMulti (2, "X"); String y2 parms.getMyCmpdMulti (2, "Y"); String z2 = parms.getMyCmpdMulti (2,"Z");
The following example shows a multi-instance compound parameter with members.
Service Action Parameter Name: CMPDMULTIMBR Atomic Action Parameter Name: CMPDMULTIMBR Order Format: CMPDMULTIMBR[1].A CMPDMULTIMBR[1].B CMPDMULTIMBR[1].C CMPDMULTIMBR[2].A CMPDMULTIMBR[2].B CMPDMULTIMBR[2].C Usage: MyCmpdMultiMbrBean[] mycmpdmultimbr = parms.getMyCmpdMultiMbr(); for (int i = 0; i <mycmpdmultimbr.length; i++) { MyCmpdMultiMbrBean bean = mycmpdmultimbr[i]; bean.getA(); bean.getB(); bean.getC(); }
The following example shows an indexed compound parameter with no members.
Service Action Parameter Name: CMPDIDX[++] Atomic Action Parameter Name: CMPDIDX Order Format: Entries will have the compound name as a prefix. There may be multiple entries with that prefix. For example, a compound named "CMPDIDX" may have the following entries on an order. CMPDIDX[0] CMPDIDX[0].X CMPDIDX[0].Y CMPDIDX[0].Z CMPDIDX[1].X CMPDIDX[1].Y CMPDIDX[1].Z Usage: String mycmpdidx = parms.getMyCmpdIdx(); String x = parms.getMyCmpdIdx ("X"); String y = parms.getMyCmpdIdx ("Y"); String z = parms.getMyCmpdIdx ("Z");
Note:
The implementation will be called multiple times, providing one instance of the compound during each call.
The following example shows a compound parameter with members.
Service Action Parameter Name: CMPDIDXMBR[++] Atomic Action Parameter Name: CMPDIDXMBR Order Format: CMPDIDXMBR[0].A CMPDIDXMBR[0].B CMPDIDXMBR[0].C CMPDIDXMBR[1].A CMPDIDXMBR[1].B CMPDIDXMBR[1].C Usage: MyCmpdIdxMbrBean mycmpdidxmbr = parms.getMyCmpdIdxMbr(); mycmpdidxmbr.getA(); mycmpdidxmbr.getB(); mycmpdidxmbr.getC();
Note:
-
The implementation will be called multiple times providing one instance of the compound during each call.
-
For multi-instance compounds, member parameters cannot be set as required because the system cannot determine whether a member is present or if there are additional entries.
Example: Typical Processor Call Sequence
The proxy:
-
The proxy creates the input, the output, and the exit type classes.
-
The proxy populates the exit type classes and initializes them.
-
The proxy creates the processor that will be called and initializes it.
-
If the logger needs to be used by the processor, the proxy provides this during the
init
method call. -
The proxy invokes the processor by calling the
execute
method with the input, output, connection, and exit type. -
The processor obtains parameters from the InputBean to compose a message or command to be sent to a device.
-
The processor calls the
send request
to send that message to the device. -
The processor sets the exit type based on the response.
-
The processor sets output parameters based on the response.
The processor may parse the response to obtain additional values for populating the output parameters.
-
The proxy cleans up the processor.
-
The proxy looks at the exit type that was set and populates it for return to the NEP, and cleans up the exit type.
-
The proxy extracts all output parameters for return to the NEP and to populate the work order.
The proxy then cleans up this class and (16) the remaining classes.
Writing Java Processor Execute Method Logic
The basic development steps to write the logic for the execute method of a Java Processor class are as follows:
To write Java Processor execute method logic:
-
Extract parameters from InputBean (retrieve information).
-
Use these parameters to build a command.
-
Send a message or command to the switch using the send request in Telnet.
-
Handle the response by setting the user-defined exit type.
See "Configuring Base Exit and User Exit Types" for more information about setting the user-defined exit type.
-
Using the OutputBean, you have the option to return some parameters upstream to log, infoparm, and so on.
Occasionally, for Telnet, you may need to build some helper classes, perform data derivation, and create parsers.
Example: Telnet Provisioning Class Flow
The following list describes the flow for Telnet provisioning classes.
-
Initialize generic data
-
Get the connection reference
-
Get the NE ID
-
Enable the response log
-
Get the work order parameters and build the AsapParameter objects passing the parameter label and value
-
Build the provisioning command with a specific action type and append parameter objects to the command
-
Convert the command to a string
-
send the command to the switch
-
Obtain the NE response
-
Exit with the appropriate user-defined -> base exit type.
//***** initialize generic data: get connection reference, get ne ID, enable response log initialize(); //****** get work order parameters and build AsapParameter objects passing the param label and value String imsi = getParam(IMSI); String bserv = getParam(BSERV); String msisdn = getParam(MSISDN); String nbr = getParam(NBR); AsapParameter imsiParm = new AsapParameter(IMSI, imsi); AsapParameter bservParm = new AsapParameter(BSERV, bserv); AsapParameter msisdnParm = new AsapParameter(MSISDN, imsi); AsapParameter nbrParm = new AsapParameter(NBR, bserv); //***** Build the provisioning command with a specific action type and append parameter objects to the command ProvisioningCommand cmd = new ProvisioningCommand(SAConstants.CREATE_BASIC_SRV); //***create command for adding a basic service cmd.append(imsiParm).append(bservParm).append(msisdnParm).append(nbrParm); //***** command ready! convert it to string mml and send it to the switch String strCmd = cmd.toString(); String reply = sendNeRequest(strCmd); //*****handle response, set user exit type etc handler.checkResponse(reply); //optional UserExitType uet = handler.getUserExitType(reply); setASDLExitType(uet.getUserExitType(), uet.getUserErrorText());
About Writing Java Programs from Scratch and Naming Conventions
You must extend the com.mslv.activation.jinterpreter.JProcessor class when writing Java code from scratch. Oracle recommends using the auto-generated code options. For more information about JProcessor, see ASAP Java Online Reference.
This section provides naming convention recommendations when writing Java programs from scratch.
Associating an Action Processors to the Java Code
To associate and action processor to the Java code you created:
-
Open the action processor editor and select the Editor tab.
-
From the Type list, select Java Action Processor.
-
In the Class field, enter a class name.
-
In the Method field, enter a method name.
-
Manually create the Java classes and methods to implement your network connection.
Java Package Naming Convention
The Java package naming convention consists of the constant prefix com.oracle.cartridge.oss in lowercase, with each of the tokens separated by a period (.) character. Each of the tokens must be separated by an underscore (_) character. The format of a Java package is as follows:
com.oracle.cartridge.oss.vendor_technology_softwareload_entity_action_
Where:
-
vendor: specifies the vendor name (see "Selecting the Vendor Token").
-
technology: specifies the technology (see "Selecting the Technology Token").
-
softwareload: specifies the software load (see "Selecting the Software Load Token"). Java class naming conventions exclude all period (.) characters from the software load token. For example, software load version 5.1 must appear as 51.
-
entity: specifies the entity (see "Selecting Entity Tokens").
-
action: specifies the action (see "Selecting the Action Tokens").
The following example illustrates the structure of a Java package used for the Alcatel-Lucent fiber to the user (FTTU) node running software load 7, providing the pay-per-view (PPV) service, with a buy action:
com.mslv.activation.cartridge.alu.fttu.7.ppv.buy
The convention used in most cartridges is based on the Metasolv name. For example com.mslv.cartridge.activation.cartridge.
Java Class Naming Convention
A Java class is a single entity that is contained within a Java package. The following list contains each of the types of cartridge Java classes and their corresponding naming conventions:
Connection class—Connection.java (for example HLRConnection.java) Provisioning class—<*>Provisioning.java (for example HLRProvisioning.java) Library class—<*>Lib.java
Class names should be nouns, in mixed case, with the first letter capitalized and with the first letter of each internal word capitalized. Try to keep your class names simple and descriptive. The wildcard token (*) used in the naming convention for provisioning and library classes is an optional string that can be used to either divide a provisioning or library class that is too large in size or identify a group of related features that are contained in the provisioning or library classes.
Java Helper and Utility Class Naming Convention
Java helper and utility class file names consists of a series of tokens that are separated by the underscore (_) character. Each token must begin with a lowercase letter. The ".jar" constant always appears at the end of the Java library file name to identify the file as a Java library file. The maximum allowable length for a Java library file name is dictated by the operating system.
The format of Java helper and utility classes is as follows:
vendor_technology_softwareload_entity.jar
Where:
-
vendor: specifies the vendor name (see "Selecting the Vendor Token").
-
technology: specifies the technology (see "Selecting the Technology Token").
-
softwareload: specifies the software load (see "Selecting the Software Load Token"). Java class naming conventions exclude all period (.) characters from the software load token. For example, software load version 5.1 must appear as 51.
-
entity: specifies the entity (see "Selecting Entity Tokens").
The following example illustrates the structure of a Java library file that contains the byte code to support VDSL service activation on a Alcatel-Lucent FTTU NE running software load 7.2:
alu_fttu_72_vdsl.jar
Java Method Naming Convention
Methods should be verbs. Tokens contained in the Java methods names are concatenated or separated using a combination of the period (.) and underscore (_) characters. The Java method naming convention consists of two tokens that are concatenated. The format of a Java method is as follows:
actionentity
Where:
-
action: specifies the action (see "Selecting the Action Tokens").
-
entity: specifies the entity (see "Selecting Entity Tokens").
The first letter of the action must appear in lowercase and the first letter of all subsequent tokens must appear in uppercase. The following example illustrates the structure of a Java method used for the Alcatel-Lucent FTTU NE supporting a pay per view (PPV) service:
addPpv
Java Variables Naming Convention
Variable names should be short yet meaningful. The choice of a variable name should be a mnemonic, and designed to indicate the intent of its use. One-character variable names should be avoided except for temporary "throwaway" variables. Common names for temporary variables are i, j, k, m, and n for integers; c, d, and e for characters.
Understanding Unit Testing
Unit testing in Design Studio does not need to be implemented to complete a cartridge, although it is highly recommended for these reasons:
-
Unit testing contributes to building quality code.
-
Unit testing provides repeatable tests for regression.
You can test the processor outside of the ASAP system because the interfaces and generative classes of the Java processor are all independent of the ASAP system and its classes (the generated InputBeans and output are not tied to ASAP). To run the processor, a TestCase
is generated once (with a sample test based on information at the time of creation), after which the developer owns it and can extend it.
The unit test framework initiates all tests in test subfolder. Unit testing is implemented as a JUnit test. JUnit tests can optionally be run with the JDT Debugger.
Figure 12-2 shows the generated test case and how it relates to the processor, input, and output files.
The TestCase
simulates the proxy for each individual test, and:
-
Creates an implementation of the interfaces, either the real implementation or a stubbed test implementation.
-
Generates input and output beans.
-
Invokes the processor.
The TestCase
is a JUnitTestCase
. Each TestCase
can contain many tests, and each test is defined by a no-parameter method beginning with "test".
The generated TestCase
has a framework that provides a test. The test runs based on input files, which find the data and test criteria for a particular test. This framework enables developers to create simple files to define new tests. This works for any standard type of test where you pass in data and check the request to ensure it was sent as expected, and that the returned exit type is the one you expected. Also, this allows for a simple, standard response to be used inside the test.
Sample test classes are provided for simulating IExit
and ILogger
. A base output class provides the methods required for output classes.
Running Unit Test Cases
Run the TestCase
class as a JUnit test, or as a Java application. Running as a JUnit test provides a richer user experience by providing results in the JUnit view. Running as a Java application allows the TestCase
to be run as part of an automated test framework. Java application test case results appear in the Eclipse IDE in the Console view.
To run unit test cases:
-
Right click the TestCase class and select Run As.
-
Select JUnit Test or Java Application.
Design Studio displays the results in the JUnit view or Console view, depending on your selection in step 2. Logging information is sent to the Console View.
Running Unit Tests with the JDT Debugger
To run unit test cases with the JDT debugger:
-
Set breakpoints in your Processor class as desired.
-
Right click the TestCase class and select Run As.
-
Select JUnit Test or Java Application.
The unit test is run and the debugger will break as appropriate, allowing for full debugger functionality, including variable inspection and code stepping.
Understanding Unit Test Property Files
You use a set of property files to set up a unit test (both are property file and follow the Java property file format):
-
testdata file (for example, TestExample.testdata)
-
testinfo file (for example, TestExample.testinfo).
Note:
The testinfo file is optional. Design Studio uses defaults if it is not present.
Testdata file
The testdata format for naming the input parameters is similar to that within a work order. However, you must populate the test data with atomic action labels (and not service action labels). Run the unit test as if the parameters have been previously defaulted.
Apply the defaults that are normally set by the SARM (based on what is configured in the atomic action) as if they had been applied in the test data (the processor runs after those defaults have been set). The unit test data should be based on data that has already been defaulted and based on names relating to the atomic action label (and not the service action label).
When you fill in the test data for compounds or incoming repeating elements, use square brackets to indicate the index for a compound as in the following example.
# Example Action Processor input property file NETID=ERIC-SDP_3-6-2-HOST MSISDN=0701234567 FAF_LIST[1].FAF_N=0701237777 FAF_LIST[1].TSC=O FAF_LIST[1].RCO=1 FAF_LIST[1].K=400 FAF_LIST[2].FAF_N=07052 FAF_LIST[2].TSC=4 FAF_LIST[2].K=100 FAF_LIST[3].FAF_N=071 FAF_LIST[3].K=500
Testinfo file
You can use this optional file to define the properties for which you are testing. You can also define what expected request the processor should create, the expected canned response returned to the processor, the expected exit type and whether it should be tested.
Note:
If you do not define a testinfo file, then by default the test case only tests whether the exit type is succeed (that is, to confirm that the test data has gone through).
# Example Action Processor test info property file request.check=true request.value=Test Message response.value=Test Response # Exit Type values: # SUCCEED # FAIL # RETRY # MAINTENANCE # SOFT_FAIL # DELAYED_FAIL # STOP exittype.check=true exittype.value=SUCCEED
If you wish to have multiple request and response values in your test, you can specify multiple values in the testinfo file. Add a dot separated numeric suffix to the value (starting at 1).
If your request or response has multiple lines or special character, follow the standard Java property guidelines.
# Example Action Processor test info property file request.check=true request.value.1=Test Message 1 request.value.2=Test Message 2 response.value.1=Test Response 1 response.value.2=Test Response 2 # Exit Type values: # SUCCEED # FAIL # RETRY # MAINTENANCE # SOFT_FAIL # DELAYED_FAIL # STOP exittype.check=true exittype.value=SUCCEED
Configuring a Unit Test
To configure a unit test:
-
Select File, select New, then select File.
-
Create a file name.testdata.
For example, you might create a file called TestExample.testdata.
Note:
Place this file in a subfolder of the action processor implementation package named test.
-
Enter the text for the file.
The file format is a Java property file, so each entry specifies the parameter and its value.
-
Repeat steps 1 and 2 as necessary to create a second file name.testinfo.
For example, you might create a file called TestExample.testinfo.
Understanding Java Libraries in Design Studio
There are several types of Java libraries available in Design Studio.
Referenced Libraries
Activation libraries are utilized by many cartridges and include the following:
-
studio_2_6_0.jar: This library contains the base implementation files extending JProcessor for auto-generated Java stubs and auto-generated CLI code. For more information about JProcessor, see the ASAP Java Online Reference.
-
asaplibcommon.jar: This library contains the core ASAP packages. For more information, see the ASAP Java Online Reference.
-
JInterp.jar: This library contains the jinterpreter packages, classes, and methods that you can use to develop Java programs that the JNEP uses to communicate with NEs. For more information about the jinterpreter packages, see the ASAP Java Online Reference.
Activation libraries are automatically added to the project when you create an action processor. They are added to the project classpath to enable the Java development toolkit access.
Note:
-
The studio_2_6_0.jar file is not installed by the ASAP installation. The studio_2_6_0.jar must be added to the ASAP installation prior to deployment of a Design Studio-created cartridge. Configure the studio_2_6_0.jar, asaplibcommon.jar, and JInterp.jar files on the server. See the discussion of installing a cartridge using Design Studio in ASAP Installation Guide.
-
When you are packaging a cartridge, exclude the studio_2_6_0.jar, asaplibcommon.jar, and JInterp.jar files. These JAR files are installed on the Activation server and are shared by all cartridges If you include these JAR files, Design Studio generates an error.
Other Libraries
Add other libraries to the lib folder under the project. Update the Java project properties to set the Java buildpath to make use of those libraries. See Eclipse help for adding folder or packages to the Java buildpath.
In the Project editor Packaging tab, select Libraries to display any jar files contained in the lib folder.
Programming Best Practices
The following sections include programming best practices applicable for writing Java implementations.
Using Default Values
Avoid hard coding default values in the Java methods. If there is a need to set a default value for one or more parameter the atomic action default configuration should be used (see tbl_asdl_parm).
Even if a default value has been configured in the cartridge (tbl_asdl_parm) for a particular parameter there is no guarantee that a default will be assigned in the customer specific service model (for example common service model), therefore the Java code cannot assume that the parameter will have a value and should therefore verify that it is not NULL before attempting to use it.
Enabling Value and Range Checking
The Java code must verify that parameters have a non-null value and log an error to the diagnostic file if such a parameter is missing (even if it is expected that it will be configured as a "required" parameter within the SARM) that are needed by an NE to ensure successful execution of the provisioning command. This checking is often used in common service modeling scenarios where it is not possible to perform error checking at the atomic action level. For example, a parameter may be required by one vendor, technology and software load and not another.
Perform value and range checking of atomic action parameters where possible within the Java code when an NE does not respond with a meaningful error message indicating which parameter has an invalid range/value.
If an NE expects a variable to be padded in some way the cartridge should perform the padding.
Logging Diagnostic Messages
Ensure that the ASAP core code (as well as cartridge code) does not write to stdout and stderr unless absolutely necessary. Instead, diagnostic messages should be written to the ASAP diagnostic files when required. For more information, see the Java diag method in the Diagnosis class in the ASAP Java Online Reference.
When ASAP is started, stderr and stdout messages are explicitly redirected to a file called ASAP.Console. For more information about the start_control_sys script that is called by the start_asap_sys script, see ASAP System Administrator's Guide. Writing to stdout and stderr can result in the ASAP.Console file dramatically increasing in size.
When logging optional parameters to the diagnostic files be sure to check if they have actually been defined first (including the MCLI parameter which is optional if ID_ROUTING is being used).
Do not log passwords of any kind (NE login passwords, database connection passwords etc.) to the ASAP diagnostic files.
Remove all internal debugging related diagnostic messages from the cartridge code when unit testing by the cartridge developer is complete.
Three diagnostic logging levels can be used within the cartridges. The developer can use KERN that should provide diagnostic messages more technical and debugging related. Use LOW for diagnostic message that are more cartridge related to show important information during development phase and test phase. Use SANE for diagnostic messages that are more informational. For more information about diagnostic levels, see ASAP Administrator's Guide.
Log messages which are stored in SARM database table tbl_srq_log to provide cartridge related information about work orders. For the telnet base cartridge, ASAP has already implemented that functionality, but for the CORBA, SOAP and another protocols you need to implement log messages, providing information about which method was run, and provide all atomic action parameters implemented in the method, log NE response, and error messages.
TCP/IP Message Parsing Options
When using the TCP/IP protocol you can take the following two approaches when parsing responses from the NE:
-
parsing the raw response
-
using the virtual screen in conjunction with ASAP core method calls.
Parsing the raw response means that more cartridge code is required, however it results in improved performance. In domains such as wireless where high volumes of work orders are expected, consider parsing the raw response from the NE.
The virtual screen mechanism extracts only the meaningful text strings from the responses and places them in the correct position on a two dimensional virtual screen where responses may be extracted using Cartesian coordinates. This approach results in less cartridge code however it decreases the performance of the cartridge. Use the virtual screen approach in low volume scenarios where ease of implementation is preferred.
Use of Journal Functionality
Some switches provide a journal ID as a response when a command is processed. If a subsequent error occurs on a later provisioning activity (either to the same switch or a different one) and rollback is therefore initiated, the journal ID can be used to undo commands that have previously been processed. This way, the cartridge does not have to keep track of exactly what commands were performed or query the switch in anticipation of rollback being performed (for example, to get the features on a line before a delete is performed so that they could be reapplied to the line at a later time). The journal IDs do however need to be remembered as each command is processed until the work order is completed.
Cartridges must support journaling capability where provided by the NE and should support use of this approach for rollback purposes.