Portlet Developer's Guide
-
Contents
Overview
What is a Portlet?
Creating a Portlet Application
Defining the Portlet JSP
Working Within the Portal Framework
Extending the PortalJspBase Class
Accessing Portal Session Information
Sending Requests Through the Portal Service
Manager
Using URL Links in Your Portlet
HTML Form Processing
Retrieving the Home Page
Retrieving the Current Page
Setting the Request Destination
Tracking User Login Status
Loading Content from an External URL
Using Example Portlets
Portlet JSP Example
Overview
The BEA WebLogic Portal enables you to create your own Business-to-Business
or Business-to-Consumer Internet portal solution. An integral part of
any portal solution is the portlet application. This guide explains
what you need to know to create a portlet application including:
-
The definition of a portlet and a portlet application
-
The steps necessary to develop a portlet application
-
Portlet examples
-
The code necessary to create a portlet application
To create a portlet application, you should be a J2EE developer with
a background in JavaServer Pages (JSP), JavaScript and HTML, and
have a knowledge of Enterprise Java Beans. Also, as a portlet developer,
you need to read this document to learn about the BEA WebLogic Portal
framework and have experience with configuring and running the WebLogic
Server.
What
is a Portlet?
From the end-user point-of-view, a portlet is a specialized content area
that occupies a small 'window' in the portal page. For example, a portlet
can contain travel itineraries, business news, local weather, or sports
scores. The user can personalize the content, appearance, and position
of the portlet according to the profile preferences set by the administrator
and group to which the user belongs. The user can also edit, maximize,
minimize, or float the portlet window.
The following figure shows how portlets appear in a portal home page:
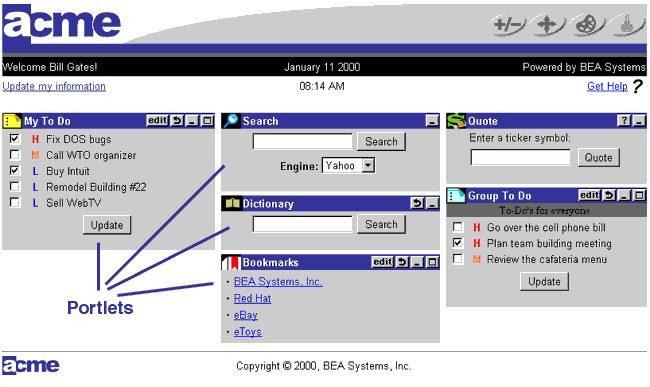
From a server application point-of-view, a portlet is a content component
implemented as a JSP that defines the static and dynamic content for
a specific content subject (weather, business news, etc.) in the portal
page. The portlet JSP generates dynamic HTML content from the server
by accessing data entities or content adapters implemented using the
J2EE platform. The Portlet JSP then displays the content in the portal.
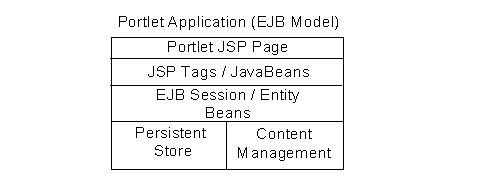
The diagram shown above defines the portal application programming
model. This programming model includes JSP, JSP tags, JavaBeans, EJBs,
data stores, and content management stores. The portlet JSP contains
static HTML and JSP code. This JSP code uses application or content
specific JSP tags and/or JavaBeans to access dynamic application data
through EJBs, content adapters, and legacy system interfaces. Once this
data is retrieved, the portlet JSP applies HTML styling to it and the
generated HTML is returned in the HTTP request to the client HTTP client.
Creating
a Portlet Application
The portlet application is a JSP that contains code responsible for retrieving
personalized content and rendering it as HTML.
Once you have created your portlets, you can associate them with one
or more portals. Therefore, you must create your portlet applications
before using the Portal Administration Tool to create and define your
portal.
Defining the Portlet JSP
The portal treats portlets as components or HTML fragments, not as entire
HTML documents. The portal relies on the portlet application to create
an HTML fragment for its portlet content. The portal renders the portlet's
content in the portal page according to the personalization rules (the
row and column position, colors, etc.) for the portal, group, and user
levels.
When creating a portlet application, keep the following items in mind
to ensure that your portlets run efficiently:
-
Avoid using forms in a portlet that update the data within the portlet.
This causes the entire portal to refresh its data which can be very
time consuming. For more information on using an HTML form in a portlet,
see HTML Form Processing.
-
Place items that require heavy processing in an edit page or a maximized
URL. Otherwise, the portal must wait for the portlet to process which
considerably slows down the painting of the portal.
To define your portlet JSP:
-
Create a JSP for your portlet content. This page is referred to
as the 'content URL' in this document and in the Portal
Administrator's Guide.
-
Create JSPs for the portlet banner, header, footer, alternate header,
alternate footer, help page, and edit URL as needed. For more information
on creating JSPs, see the Portal Administrator's
Guide.
Note: You do not need to create a JSP for the portlet title
bar because it is included in the BEA WebLogic Portal (example/titlebar.jsp).
The portlet title bar displays the appropriate portlet titlebar icons
and the name of the portlet you defined in the Portal Administration
Tool. See, Appendix A in the BEA
Portal Administrator's Guide for a complete listing of the BEA WebLogic
Portal framework JSP resources.
Note: Avoid using the following HTML tags in your portlet
content page. The HTML generated by the portlet content page is an
HTML fragment contained in a larger portal HTML page, not a separate
HTML document.
-
<html></html>
-
<header></header>
-
<body></body>
-
<meta></meta>
-
<title></title>
-
Use the following portlet layout guidelines.
Layout Attribute
|
Recommendation
|
Content Height
|
There are no restrictions on height as long as the content fits
in your portal page.
|
Column Width
|
Take into account that the width of your portlet is controlled
by the portal(s) it is associated with. A portal lays out your portlet
content in a column based on portal, group, and user personalization
rules. As a result the width of your portlet should be well behaved.
|
Content Wrapping
|
Allow wrapping for all portlet content. Do not use the
NOWRAP attribute in table cells.
|
Titlebar Icon Height
|
The image height attribute in titlebar.jsp is set to 20.
|
Titlebar Icon Width
|
The image width in titlebar.jsp is set to 27.
|
Working
Within the Portal Framework
The portal framework consists of JavaServer Pages, JSP tag libraries,
EJBs, Java servlets, and other supporting Java objects. The main Java
servlet is the Portal Service Manager, referred to as the 'traffic uri'.
The Portal Service Manager receives all incoming HTTP requests and dispatches
each request to the appropriate destination URL. As a result, all access
to your portlet pages is controlled by the Portal Service Manager. The
following diagram shows where the Portal Service Manager fits in the portal
framework.
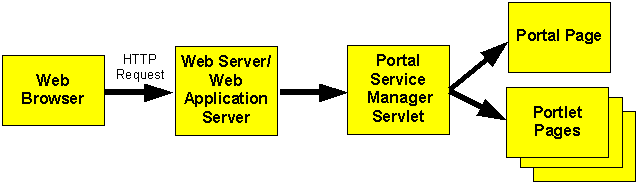
Extending the PortalJspBase
Class
It is recommended that your portlet JSP extend the framework's PortalJspBase
Java class. This class contains many convenience methods which perform
general tasks for your portlet JSP page, such as accessing session information,
the 'traffic uri', and user login information.
To extend the PortalJspBase class, include the following code at the
top of your portlet JSP:
<%@ page
extends="com.beasys.portal.admin.PortalJspBase"%>
Accessing Portal Session
Information
The session information you can access from the PortalJspBase class
are listed in the following table which lists the name, type, and description
for each session value. For more information, see the Portal
API Documentation.
Session Value Name
|
Type
|
Description
|
PortalAdminConstants.PORTAL_NAME
|
String
|
The name of the portal associated with the current request.
|
PortalAdminConstants.PORTAL_USER
|
String
|
The name of the user associated with the current request.
|
PortalAdminConstants.PORTAL_GROUP
|
String
|
The name of the user group associated with the current request.
|
PortalAdminConstants.PROFILE_USER
|
String
|
The name of the user profile associated with the current request.
|
PortalAdminConstants.PROFILE_GROUP
|
String
|
The name of the group profile associated with the current request.
|
You can retrieve the session information described above through the
following PortalJspBase methods:
-
public Object getSessionValue(String
aName, HttpServletRequest aRequest)
-
public void setSessionValue(String
aName, Object aValue, HttpServletRequest aRequest)
-
public void removeSessionValue(String
aName, HttpServletRequest aRequest)
Sending Requests Through
the Portal Service Manager
Remember that all HTTP requests and responses are sent to the Portal
Service Manager servlet. Therefore, your portlet HTML must refer to the
Portal Service Manager's URL for URL links and HTML form processing.
Using URL Links in Your Portlet
If your portlet contains links to other portal pages, use the following
PortalJspBase method to create your URL and to guarantee that the HTTP
request is sent to the service manager URL:
public String createURL(HttpServletRequest
aRequest, String destination, String parameters)
The destination should be a relative or qualified file location in the
form such as example/mytodo.jsp,
or /yourportal/example/mytodo.jsp.
Parameters should be a string such as column=4&row=5.
Note: Parameter values should already be encoded - as you would
for any HTTP request. For example, String
parms = "column=" + java.net.URLEncoder.encode("4");
Because of the way the JSP engine handles jsp:forward
and jsp:include,
you must 'fixup' the relative URLs in your portlet, especially relative
links to images. The web browser thinks the root for relative links is
the directory in which the Portal Service Manager resides and not your
portlet's directory.
To fixup relative URLs use the following PortalJspBase method:
public static String
fixupRelativeURL(String aURL, HttpServletRequest aRequest)
where aURL is
the destination URL to fix up and aRequest
is the current HTTP request.
For example, in your JSP page, use the following method to code a 'fixup':
<img src="<%=fixupRelativeURL("images/quote.gif",
request)%>"
width="50" height="35" border="0">
HTML Form Processing
If your portlet contains an HTML form, send all requests to the Portal
Service Manager and set the destination request parameter.
To process HTML forms:
-
Set the form action to action=getTrafficURI(request).
This sends the form action request to the Portal Service Manager.
This calls the PortalJspBase method:
public String getTrafficURI(HttpServletRequest
aRequest)
The following example shows the use of the HTML form action to send
a form request to the Portal Service Manager:
<form method="post"
action="<%=getTrafficURI(request)%>">
-
Set the destination request parameter in the HTTP post request. This
tells the Portal Service Manager where to dispatch the request. For
more information, see Setting the Request Destination.
Note: Do not go through the Portal Service Manager for HTTP requests
to other servers.
Retrieving the Home Page
The Portal Service Manager sets the home page for each portal in the
Portal Framework session information. Use the following PortalJspBase
method call to retrieve the home page:
public String getHomePage(HttpServletRequest
aRequest)
Retrieving the Current
Page
You can also retrieve the current page from the Portal Framework session
information by using the following PortalJspBase method:
public String getCurrentPage(HttpServletRequest
aRequest)
Note: When you maximize a portlet, the current page changes
to fullscreenportlet.jsp.
Setting the Request Destination
When routing a request through the Portal Service Manager, you must
specify the destination that should receive the request. The destination
can be relative to the current page or a fully qualified path from the
document root.
For HTML forms, to set the request destination, enter the following code
within your form in your JSP page:
<input
type="hidden" name="<%=DESTINATION_TAG%>" value="example/mytodo.jsp">
Note:
The
DESTINATION_TAG constant is available in
PortalJspBase.
If your portlet contains links to other portal pages, use the following
PortalJspBase method to create your URL and to guarantee that the HTTP
request is sent to the service manager URL:
public String createURL(HttpServletRequest
aRequest, String destination, String parameters)
The destination should be a relative or qualified file location in
the form such as example/mytodo.jsp,
or /yourportal/example/mytodo.jsp.
In some cases you may need to override the request parameter used by
the Portal Service Manager. For example, use an override destination if
your page contains a form that needs to be validated and forwarded elsewhere
after validation. Use the following method in your JSP page:
Tracking User Login Status
You can log the user in or out and track whether a user is currently
logged in.
Use the following method to track the user login status of a portal session:
public void setLoggedIn(HttpServletRequest
aRequest, HttpServletResponse aResponse, boolean aBool)
public Boolean getLoggedIn(HttpServletRequest
aRequest)
Loading Content from an
External URL
According to the JSP specification, a JSP processed by a JSP engine must
be relative to the server in which the JSP engine is running, requiring
that all of your portlets reside in your portal server and not on an external
web site. However, you can use the 'uricontent' tag to download the contents
of an external URL into your portlet. If you download the contents of
a URL into your portlet, you need to fully qualify the images located
on the remote server because the relative links contained within the remote
URL will not be found unless fully qualified.
Use the following method to load content from an external URL:
<es:uricontent
id="uriContent" uri="http://www.beasys.com/index.html">
<%
out.print(uriContent);
%>
</es:uricontent>
Note: The sample 'uricontent' tag is available in example/portlets/_uri_example.jsp.
Using Example
Portlets
The /portlets/example/portlets
directory of the BEA WebLogic Portal contains example portlets. The following
table lists the name of each example portlet, its description, and its
associated files.
Caution: The example portlets are intended for illustration purposes
only and should not be used for production code.
Example Portlet
|
Description
|
_uri_example.jsp
|
Demonstrates how to implement the 'uricontent' tag to import contents
from another URL on the Internet.
|
bookmarks.jsp
|
Displays the bookmarks associated to the current user.
|
definedportals.jsp
|
Displays the portals defined in the system. Uses the <es:foreachinarray>,
<es:simplereport>, and
<wb:sqlquery tags>.
|
definedportlets.jsp
|
Displays the portlets defined in the system. Uses the <es:foreachinarray>,
<es:simplereport>, and
<wb:sqlquery tags>.
|
dictionary.jsp
|
Demonstrates how to redirect a portlet to an external site.
|
generic_todo.jsp
|
For a complete generic_todo.jsp
example, see Using the Default Implementation.
|
grouptodo.jsp
|
Displays a 'Group To Do List'.
-
todo.jsp
– Statically included file that does not run by itself. It requires
user information from grouptodo.jsp.
-
grouptodo_edit.jsp
– Edit URL for grouptodo.jsp.
-
grouptodobanner.jsp
– Banner for the grouptodo.jsp.
-
images/pt_group_list.gif
– 'Group To Do List' icon for the portlet titlebar.
|
mytodo.jsp
|
Displays a 'My To Do List".
-
todo.jsp
– Statically included file that does not run by itself. It requires
user information from mytodo.jsp.
-
mytodo_edit.jsp
– 'Edit URL' for mytodo.jsp.
-
images/pt_my_list.gif
– 'My To Do List' icon for the portlet titlebar.
|
quote.jsp
|
Demonstrates how to redirect a portlet to an external site.
|
search.jsp
|
Demonstrates how to redirect a portlet to an external site.
|
Portlet
JSP Example
The following example shows many of the defined method calls and tags
mentioned in this document. Each tag is a file in the default implementation
directory portals/example/portlets,
named generic_todo.jsp.
The bean associated with this file is example.portlet.bean.TodoBean.
Using these files, you can recreate each example in your JSP file.
<%--
Set up the tag libraries for tag references.
Also have the page extends PortalJspBase, so that you can have access
to helper methods.
--%>
<%@ taglib uri="lib/esjsp.jar" prefix="es" %>
<%@ page extends="com.beasys.portal.admin.PortalJspBase"%>
<jsp:useBean id="todoBean" class="example.portlet.bean.TodoBean" scope="request"/>
<jsp:setProperty name="todoBean" property="*"/>
<%
// Get the user name out of the session
String owner = (String)getSessionValue(com.beasys.portal.tags.PortalTagConstants.PORTAL_USER,
request);
%>
<%-- Use the preparedstatement tag to execute a query --%>
<es:preparedstatement id="ps" sql="<%=todoBean.QUERY%>" pool="jdbcPool">
<%
todoBean.createQuery(ps, owner);
java.sql.ResultSet resultSet = ps.executeQuery();
todoBean.load(resultSet);
%>
</es:preparedstatement>
<%
String target = request.getParameter("target");
//
Use this method to validate that the request that is being
// processed is actually for this jsp page.
if ( target != null && target.equals(getRequestURI(request)))
{
%>
<es:preparedstatement
id="ps" SQL="<%=todoBean.UPDATE%>" pool="jdbcPool">
<%
todoBean.process(request,
owner, ps);
%>
</es:preparedstatement>
<%
}
//
Get the enclosing window out of the session
// Get the current page out of the session.
// For this example, this value will be the
// fullscreenportlet.jsp (with args) or portal.jsp.
String value = getCurrentPage(request);
%>
<%--
set the action on the form to send the post back to the 'traffic cop'
--%>
<form
method="post" action="<%=getTrafficURI(request)%>">
<table
width="100%" border="1">
<tr>
<td>
<table
width="100%" border="0">
<%
String[][]
results = todoBean.asTable();
%>
<%--
Use the foreachinarray tag to iterate over the query results.
--%>
<es:foreachinarray
id="nextRow" array="results" type="String[]">
<TR>
<td
width="10%">
<div
align="center">
<input
type="checkbox" <%=nextRow[0]%> name="<%=todoBean.CHECKBOX+nextRow[2]%>"
value="ON">
</div>
</td>
<td
width="10%" align="center"><%=nextRow[1]%></td>
<td
width="80%"> <%=nextRow[2]%></td>
</TR>
</es:foreachinarray>
</table>
</td>
</tr>
<tr>
<td>
<div
align="center">
<input
type="submit" name="updateButton" value="Update">
</div>
</td>
</tr>
</table>
<input
type="hidden" name="owner" value="<%=owner%>">
<%--
Tell the 'traffic cop' that you want the post to come back to this page.
--%>
<input type="hidden"
name="<%=DESTINATION_TAG%>" value="<%=value%>">
<%--
Use getRequestURI(request)to get this page's name and location.
Also use it if you want to verify that the request is for this page,
by setting a param for the target.
--%>
<input type="hidden"
name="target" value="<getRequestURI(request)%>">
</form>
|