JTS. You no longer need to import weblogic.jts.*, since JavaSoft now supplies JTS as
javax.jts.*, which is shipped
as a zip file with the distribution in the weblogic/lib directory.
(You must add both javax_ejb.zip and javax_jts.zip to your CLASSPATH.)
Since no standard factories have been defined in the EJB
specification, you must specify how to get an initial transaction
UserTransaction object using JNDI.
Here is an example. First import the javax classes for JNDI and JTS:
import javax.naming.*;
import javax.jts.*;
Then use WebLogic JNDI to set up a Context:
Context weblogicCtx = null;
Hashtable env = new Hashtable();
env.put(Context.INITIAL_CONTEXT_FACTORY,
"weblogic.jndi.WLInitialContextFactory");
env.put(Context.PROVIDER_URL,
"t3://localhost:7001");
try {
weblogicCtx = new InitialContext(env);
Now get a UserTransaction object from JNDI:
UserTransaction ut = null;
ut = (UserTransaction)weblogicCtx.lookup("javax.jts.UserTransaction");
ut.begin();
// Do the transaction work
ut.commit();
// Or rollback
}
Finally, close the Context:
catch (NamingException e) {
// a failure occurred
}
finally {
try {weblogicCtx.close();}
catch (Exception e) {
// a failure occurred
}
}
Note that with the 1.0 implementation, you do not have to import anything
WebLogic-specific, nor do you need to call
setServiceProviderURL() because
you have already specified the WebLogic Server for JNDI.
Bean-related changes
There were many changes. Be sure to read the EJBean provider contracts
in the specification.
WebLogic comes with an EJB 1.0 compliance checker, which will help you
upgrade your EJBeans. To run the compliance checker, use this command:
$ java weblogic.ejb.utils.ComplianceChecker jar/ser
where the supplied jar/ser is a jar file or serialized deployment descriptor.
DDCreator runs this compliance checker internally.
In the .8 specification, the word "container" is used to refer to the Container interface. In version 1.0, this has been changed to "Remote" interface.
Deployment Descriptor changes
The structure of the deployment descriptor text file has changed to
mirror the structure specified in the spec. As before, all
WebLogic-specific deployment information is packaged under the
"environmentProperties" property.
All the changes in the DD are structural, not conceptual.
- Persistent store properties have been nested one level, below
"persistentStoreProperties".
- For JDBC, specifying a poolName is enough (and is in fact, the
preferred approach). You do not have to specify the driver URL and
driver class name, even though that option is also available.
WebLogic also provides a GUI deployment wizard.
Please see Using the WebLogic EJB Deployment Wizard.
Property-related changes
The syntax of the value you supply for one EJB-related property has
changed. Note that you now specify the fully qualified path of both
the jar and the ser files, as detailed here:
- weblogic.ejb.deploy
- The syntax for registering serialized deployment descriptors and
jar files in the weblogic.properties file has changed. This property
should be set to a comma-delimited list of full (absolute) paths to
each jar file and serialized deployment descriptor that you want to register. For
example:
weblogic.ejb.deploy=\
c:/weblogic/classes/examples/ejb/basic/beanManaged/MyBeanDD.ser,\
c:/weblogic/classes/containerManaged.jar,\
c:/weblogic.classes.statefulSession.jar
Note that backslashes have a special meaning in the
weblogic.properties file.
For more on properties to deploy EJBeans, see Setting WebLogic
properties
If you are using JDBC for persistence, also read about registering and
using a connection pool in
the EJB Developers Guide.
Utilities changes
- DDCreator now has a "-dir" option to specify the destination
location of the serialized DeploymentDescriptor. The serialized
descriptor is named after the pattern
beannameDD.ser.
You can use the "-outputfile" option to specify an alternate
base name.
- RemoteCreator and HomeCreator are not yet included in the release.
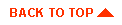