Using jdbcKona/Informix4
- I. Introduction
- What's in this document
- Mapping of types from Informix to jdbcKona/Informix4 to Java
- II. Implementing with jdbcKona/Informix4
- How to connect to an Informix DBMS with jdbcKona/Informix4
- Other properties you can set for jdbcKona/Informix4
- Retrieving the SERIAL column after an insert
- Support for the Informix INTERVAL data type
- Using ResultSetMetaData methods
- Using autocommit mode
- Codeset support
- Using Unicode streams in a prepared statement
- III. What is currently supported in jdbcKona/Informix4
- IV. Change history
- Other documents
- Installing WebLogic (non-Windows)
- Installing
WebLogic (Windows)
- Installing jdbcKona/Informix4
- Developers Guides
- API Reference Manual
- Glossary
- Using jdbcKona
- Using WebLogic JDBC
- Performance tuning your JDBC application
- Troubleshooting JDBC hangs and SEGVs
- Choosing a Java Database Connectivity driver
- Code examples
I. Introduction
What's in this document
This document includes documentation on how to set up and use WebLogic
jdbcKona/Informix4 (formerly known as FastForward from Connect
Software, acquired in April 1998). This database driver is a Type 4,
pure-Java, two-tier
driver. It requires no client-side libraries since it connects to the
database via the proprietary vendor protocol at the wire-format
level. Consequently, unlike Type 2, two-tier drivers, it makes no
"native" calls; it is written exclusively in Java.
A Type 4 driver is similar to a Type 2 driver in other ways, however.
Both Type 2 and Type 4 drivers are two-tier drivers; that means that
each client requires an in-memory copy of the driver to support its
connection to the database.
Within the WebLogic environment, you can use a Type 2 or a Type 4
two-tier driver to connect the WebLogic Server
to a database, and then use WebLogic JDBC, WebLogic's pure-Java Type 3
multitier
JDBC driver, for client connections to the WebLogic
Server. For more information on how WebLogic JDBC works with any
two-tier driver, and how connections are structured in WebLogic, read
the Tech Tip, What
do I do with all these connections, anyway?
jdbcKona/Informix4 supports concurrent ResultSet execution; that is,
you do not have to finish with and close one ResultSet on a Connection
before you can open and work with another. However, the driver
cannot support both concurrent ResultSet execution and
client-side caching, because of issues in dealing with BLOBs.
It is possible that a later version of the driver will allow
row caching if the row contains no BLOB data.
If you are using jdbcKona/Informix4 with WebLogic JDBC, you should also
refer to the Developers Guide Using WebLogic JDBC for more information.
Note that this JDBC driver is only supported with versions of the
JDK
later than 1.1 (including Java 2, JDK 1.2.x). There is no JDK 1.0.2
support. jdbcKona/Informix4 supports Informix OnLine versions 7.x and
9.x, with 7.x data types plus the 9.x INT8 and SERIAL8 data types.
Before you begin working with the jdbcKona/Informix4 driver, make sure
you have installed the driver according to the installation instructions
and, in particular, that you know the port on which the database is
listening for connection requests.
Mapping of types from Informix to jdbcKona/Informix4 to Java
-
Informix data type | jdbcKona/Informix4 | Java type
Byte | Binary | use java.io.InputStream subclasses for INPUT
| Char | Char | java.lang.String
| Date
Y4MD- ('1998-01-31') | Date | java.sql.Date
| Datetime | Timestamp
| java.sql.Timestamp
| Decimal | Decimal | java.math.BigDecimal
| Float | Decimal | java.math.BigDecimal
| Integer | Integer | java.lang.Integer
| Integer8 | Long | java.lang.BigInt
| Interval | InformixInterval | Literal string in Informix INTERVAL format for INPUT
| Money | Decimal | java.math.BigDecimal
| NChar | Char | java.lang.String
| NVarchar | Varchar | java.lang.String
| Serial | Integer | java.lang.Integer
| Serial8 | Long | java.lang.BigInt
| Smallfloat | Decimal | java.math.BigDecimal
| Smallint | Smallint | java.lang.Integer
| Text | Longvarchar | use java.io.InputStream subclasses for INPUT
Varchar | Varchar | java.lang.String
| | |
III. Implementing with jdbcKona/Informix4
- How to connect to an Informix DBMS with jdbcKona/Informix4
- Other properties you can set for jdbcKona/Informix4
- Retrieving the SERIAL column after an insert
- Using the Informix INTERVAL data type
- Using ResultSetMetaData methods
- Using autocommit mode
- Support for Informix-specific features
How to connect to an Informix DBMS with jdbcKona/Informix4
Here is how you set up your application to connect to Informix using
jdbcKona/Informix4. In general, connecting happens in two steps:
- You must load the JDBC driver. The most efficient way to load the
JDBC driver is to call Class.forName().newInstance() with the full class
name of the jdbcKona/Informix4 JDBC driver class, which properly loads and
registers the JDBC driver. Here is an example:
Class.forName("weblogic.jdbc.informix4.Driver").newInstance();
- You get a JDBC Connection object, which becomes an integral piece
in your JDBC operations, by calling the DriverManager.getConnection() method, which takes as
its parameters the URL
of the driver and other information about the connection.
In both steps, you are describing the JDBC driver: in the first
step, you use the full package name of the driver. Note that it
is dot-delimited. In the second step, you identify the driver
with its URL, which is colon-delimited. The URL must include
at least weblogic:jdbc:informix4, and may include
other information, including the server host name and the
database name.
There are several variations on this basic pattern.
Method 1
The simplest way to connect is to pass the URL of the driver that
includes the name of the server, along with a username and password,
as arguments to the
DriverManager.getConnection()
method. The pattern for the URL is:
jdbc:weblogic:informix4:[dbname]@[serverhost]:[port]?[prop1]&[prop2]
as in this example:
Class.forName("weblogic.jdbc.informix4.Driver").newInstance();
Connection conn = DriverManager.getConnection(
"jdbc:weblogic:informix4:mydb@host:8659",
"scott",
"tiger");
where mydb is the alias of an
Informix database and host:8659 is the hostname and port of the server
supporting it.
Method 2
You can also pass a java.util.Properties object with parameters for
connection as an argument to the DriverManager.getConnection() method. This example
shows how to use a Properties object to connect to a database named
"mydb" on a server named "host" (as in the example above).
Properties props = new Properties();
props.put("user", "scott");
props.put("password", "tiger");
props.put("db", "mydb");
props.put("server", "host");
props.put("port", "8659");
Class.forName("weblogic.jdbc.informix4.Driver").newInstance();
Connection conn = DriverManager.getConnection(
"jdbc:weblogic:informix4",
props);
You can combine the "db", "server", and "port" properties into one
"server" property, as in this example:
Properties props = new Properties();
props.put("user", "scott");
props.put("password", "tiger");
props.put("server", "mydb@host:8659");
Class.forName("weblogic.jdbc.informix4.Driver").newInstance();
Connection conn = DriverManager.getConnection(
"jdbc:weblogic:informix4",
props);
There are any number of variations on supplying information in the
URL or in the Properties object. Whatever information you pass in the
URL of the driver, you do not need to include in the Properties object.
Method 3
If you prefer, you can load the JDBC driver from the command line with
the command:
$ java -Djdbc.drivers=weblogic.jdbc.informix4.Driver classname
where classname is the name of the application you want to run;
and then use a Properties object to set parameters necessary for
connecting to the DBMS. In this case, you will not need to call the Class.forName().newInstance()
method, as shown here:
Properties props = new Properties();
props.put("user", "scott");
props.put("password", "tiger");
Connection conn = DriverManager.getConnection(
"jdbc:weblogic:informix4:mydb@host:8659",
props);
Other properties you can set for jdbcKona/Informix4
There are other Informix-specific properties that you can set in
the connection URL or Properties object. These properties give you
more control over an Informix-specific environment. For more information
on these variables, please refer to your Informix documentation.
- weblogic.informix4.login_timeout_secs=seconds_to_wait
-
When an attempt to log into an Informix server times out, the jdbcKona/Informix4
driver returns an SQLException. By default, the driver waits 90 seconds before it times out.
You can modify the timeout period by setting this property to the number of seconds
you want to wait before returning an SQLException.
- weblogic.informix4.delimited_identifiers=y
-
The Informix environment variable DELIMIDENT is used to enable and disable
ANSI SQL Delimited Identifiers. In
older versions before the WebLogic acquisition, this feature was
permanently set on ("y"). The default is now off ("n").
- weblogic.informix4.db_money=currency
-
The Informix environment variable DBMONEY is used to set the display of the currency
symbol. In older versions before the WebLogic acquisition, "$." was
hard-coded as the value for this variable. The default value is
now "$." which can be overridden with this property.
- weblogic.informix4.db_date=dateformat
-
The Informix environment variable DBDATE allows a user to specify the input format of
dates. It sets the Informix DBDATE environment variable at login. The
default value is "Y4MD". Two-digit years (formats containing Y2) are
not supported by the driver. Note that this variable cannot be used to
correctly format a date obtained from a ResultSet.getString() statement. Instead, use ResultSet.getDate() to obtain a
java.util.Date object and then
format the date in your code.
Here is an example of these properties used in a URL. Note that
the URL is always used as a single line but has been broken here for
simplicity.
jdbc:weblogic:informix4:mydb@host:1493
?weblogic.informix4.delimited_identifiers=y
&weblogic.informix4.db_money=DM
&weblogic.informix4.db_date=Y4MD
Note the use of "?" and "&", which are special characters for URLs.
For more on using URLs with WebLogic, read the Developers Guide, Using URLs to set properties for a JDBC Connection.
Here is how these properties might be used with a Properties object:
Properties props = new Properties();
props.put("user", "scott");
props.put("password", "tiger");
props.put("weblogic.informix4.delimited_identifiers", "y");
props.put("weblogic.informix4.db_money", "DM");
Connection conn =
DriverManager.getConnection(
"jdbc:weblogic:informix4:mydb@host:8659",
props);
Retrieving the SERIAL column after an insert
- weblogic.jdbc.informix4.Statement
You can obtain serial values after an insert by using the Statement.getSerialNumber() method,
a WebLogic extension to JDBC in jdbcKona/Informix4. This allows you to
track the index order of rows as you add them to the table. Note
that you must create the table with a SERIAL column.
To use this extension, you will need to explicitly cast your Statement
object to weblogic.jdbc.informix4.Statement.
Here is a simple code example that illustrates how to use the
getSerialNumber()
method.
weblogic.jdbc.informix4.Statement stmt =
(weblogic.jdbc.informix4.Statement)conn.createStatement();
String sql = "CREATE TABLE test ( s SERIAL, count INT )";
stmt.executeUpdate(sql);
for (int i = 100; i < 110 ; i++ ) {
sql = "INSERT INTO test VALUES (0, " + i + ")";
stmt.executeUpdate(sql);
int ser = stmt.getSerialNumber();
System.out.println("serial number is: " + ser);
}
sql = "SELECT * from test";
ResultSet rs = stmt.executeQuery(sql);
while (rs.next()) {
System.out.println("row: " + rs.getString(2) +
" serial: " + rs.getString(1));
}
Support for the Informix INTERVAL data type
Use a literal string in the Informix INTERVAL format to enter an
INTERVAL value in a SQL statement. Use preparedStatement.setString() to set an INTERVAL value
parameter in a prepared statement.
For retrieving INTERVAL data from an Informix server, the dbKona/Informix4
driver supports three standard API methods on a ResultSet:
-
ResultSet.getString() returns
a String representation of the interval in the standard Informix
format. Returns null if the interval is null.
-
ResultSet.getBytes() returns
the actual bytes the server returns to represent the interval.
-
ResultSet.getObject()
returns an object of type weblogic.jdbc.informix4.InformixInterval.
Returns null if the interval is null.
The InformixInterval class provides the following public methods:
-
String getString() throws SQLException
-
Identical to ResultSet.getString()
- int getYear() throws SQLException
- Returns the signed year of the INTERVAL, zero if YEAR is not
defined
- int getMonth() throws SQLException
- Returns the signed year of the interval, zero if MONTH is not defined
- int getDay() throws SQLException
- Returns the signed day of the interval, zero if DAY is not defined
- int getHour() throws SQLException
- Returns the signed hour of the interval, zero if HOUR is not defined
- int getMinute() throws SQLException
- Returns the signed minute of the interval, zero if MINUTE is not defined
- int getSecond() throws SQLException
- Returns the signed second of the interval, zero if SECOND is not defined
- int getFraction() throws SQLException
- Returns the actual value of the FRACTION times 10**5
Using ResultSetMetaData methods
The metadata the Informix server returns with query results
is available via ResultSetMetaData methods. However, the Informix server
does not return information for the following:
- getSchemaName(int)
- getTableName(int)
- getCatalogName(int)
Using autocommit mode
Unlike other database system attributes, the autocommit mode of an
Informix database is not dynamically settable. It is defined when the
database is created. You can't change it with a call to the Connection.setAutoCommit method.
Only non-ANSI, non-logged databases support changing autocommit.
The JDBC spec says that the autocommit mode should be true by default,
but it is not possible to do this with Informix. All that you can do
is determine what the autocommit mode is. To change the autocommit
state, you must rebuild your database (for more information, see the
information on "CREATE DATABASE" in the Informix docs).
This affects how transactions and locking work. A JDBC program could
behave very differently depending on how the Informix database is
created.
Before you depend on autocommit, you should know the setting of
autocommit for the database you will be using. You can check its state
with the Connection.getAutoCommit() method, which returns true
if autocommit is enabled. For Informix, this method returns false by
default for ANSI databases; it may return true or false, depending
on how the database was created, for a non-ANSI database.
Here is what is supported in jdbcKona/Informix4 when you call the
Connection.setAutoCommit()
method:
- For ANSI databases, only autocommit=false is supported.
- For non-ANSI databases, autocommit can be set to either true or
false.
- For non-ANSI databases without logging, only autocommit=true is
supported.
Your program must then operate in accordance with the state of your
Informix database.
If you are using a non-ANSI database and you set autocommit to false,
all transactional SQL must be carried out using the Connection.commit() or Connection.rollback() methods. You
should never execute the explicit transaction controls BEGIN
WORK, COMMIT WORK, or ROLLBACK WORK calls on a Statement, since
jdbcKona/Informix4 uses transaction commands internally to simulate an
autocommit=false status. You should always control a transaction
using commit() and rollback() methods in the Connection class.
For non-ANSI databases without logging, autocommit=false cannot be
supported, since transactions are not supported. Consequently, only
autocommit=true is supported for use with such databases.
Support for Informix-specific features
jdbcKona/Informix4 includes support for other Informix-specific
features that may not be part of the JDBC specification, but that add
power when writing a client application for an Informix database.
Creating TEMP tables
CREATE TEMP TABLE is an INFORMIX SQL command that isn't covered by the
JDBC specification. jdbcKona/Informix4 supports this Informix feature
by making the proper acknowledgment to the Informix server that allows
the SQL command to be executed.
Informix TEMP tables are useful as scratch pads. Users can create them
on the fly to hold data to be used for intermediate processing; for
example, data might be retrieved initially in an intermediate TEMP
TABLE, and then, with further selects or other operations, might be
reduced further to a more manageable size, before retrieval by a
client application. The TEMP table disappears when the session is
over or the client closes the connection. DBMSes often use TEMP tables
internally to correlate or reduce data retrieved from many or very
large tables.
Retrieving VARCHAR/CHAR data as bytes
jdbcKona/Informix4 provides an extension to JDBC for Informix that
allows users to retrieve VARCHAR and CHAR columns using the ResultSet.getBytes(String
columnName) and ResultSet.getBytes(int columnIndex) methods. Although
this is outside the scope of the JDBC specification, this was
implemented in response to customer requests. No cast of the ResultSet
is required to take advantage of this feature.
SET LOCK MODE
You can use the SET LOCK MODE command with an Informix database to
influence how the database deals with a locked record when it receives
another request to update or delete it. The command "SET LOCK MODE TO
WAIT" just means that instead of throwing an error to the client
application, the Driver should be made to wait until the record in
question becomes free (like the Oracle waitOnResource() command that is supported in
jdbcKona/Oracle).
Don't confuse the use of this command with the need to adjust a
transaction isolation level. If you have an ANSI database, then by
default the transaction level is set to serializable. Please read
Informix documentation on "SET TRANSACTION" and "SET ISOLATION" to
find out more about it.
Use of this feature depends on your application, which may not
allow a long wait for a record.
Codeset support
As a Java application, jdbcKona/Informix4 handles character strings as
Unicode
strings. To exchange character strings with a database that may
operate with a different codeset, you must set the the weblogic.codeset connection property
to the proper JDK codeset. If there is no direct mapping between the
codeset of your database and the character sets provided with the JDK,
you can set the weblogic.codeset connection property to the most appropriate
Java character set. You can find the List
of supported encodings at the JavaSoft website.
For example, to use the cp932 codeset, create a Properties object and
set the weblogic.codeset property before
calling DriverManager.getConnection(),
as in this example:
java.util.Properties props = new java.util.Properties();
props.put("weblogic.codeset", "cp932");
props.put("user", "scott");
props.put("password", "tiger");
String connectUrl = "jdbc:weblogic:informix4:mydb@host:1493";
Class.forName("weblogic.jdbc.informix4.Driver").newInstance();
Connection conn =
DriverManager.getConnection(connectUrl, props);
Using Unicode streams in a prepared statement
- weblogic.jdbc.informix4.UnicodeInputStream()
If you are using the PreparedStatement.setUnicodeStream method, you can
either create your own InputStream object, or you can create a weblogic.jdbc.informix4.UnicodeInputStream object,
using a String value in the constructor. Here is an example that shows
how to input a Unicode stream into an Informix TEXT column, Connection c =
DriverManager.getConnection(database,user,password);
PreparedStatement ps =
c.prepareStatement("insert into dbTEST values (99,?)");
String s = new String("\u93e1\u68b0\u897f");
weblogic.jdbc.informix4.UnicodeInputStream uis =
new weblogic.jdbc.informix4.UnicodeInputStream(s);
try {
ps.setUnicodeStream(1,uis,uis.available());
}
catch (java.io.IOException ioe) {
System.out.println("-- IO Exception in setUnicodeStream");
}
ps.executeUpdate();
To retrieve data from a UnicodeInputStream you can use java.io.InputStream. For example,
InputStream uisout = rs.getUnicodeStream(2);
int i=0;
while (true) {
try {
i = uisout.read(); // read 1 byte at a time from UnicodeStream
}
catch (IOException e) {
System.out.println("-- IOException reading UnicodeStream");
}
For more information, check the full example included in the distribution, in the
examples/jdbc/informix4 directory.
III. What is currently supported in jdbcKona/Informix4
jdbcKona/Informix4 is a complete and compliant implementation of the JDBC
specification, except for those features described in JDBC that are
either unsupported or unavailable in Informix. Since there is often
confusion about the implementation the DatabaseMetaData interface, we
list all of its methods here. Most are supported; some are planned for
a future release, and some (because of Informix limitations or
implementations) will not be supported for this JDBC driver.
- The following DatabaseMetaData methods are supported:
- allProceduresAreCallable()
- allTablesAreSelectable()
- dataDefinitionCausesTransactionCommit()
- dataDefinitionIgnoredInTransactions()
- doesMaxRowSizeIncludeBlobs()
- getCatalogSeparator()
- getCatalogTerm()
- getColumns()
- getDatabaseProductName()
- getDatabaseProductVersion()
- getDefaultTransactionIsolation()
- getDriverMajorVersion()
- getDriverMinorVersion()
- getDriverName()
- getDriverVersion()
- getExportedKeys()
- getExtraNameCharacters()
- getIdentifierQuoteString()
- getImportedKeys()
- getMaxBinaryLiteralLength()
- getMaxCatalogNameLength()
- getMaxCharLiteralLength()
- getMaxColumnNameLength()
- getMaxColumnsInGroupBy()
- getMaxColumnsInIndex()
- getMaxColumnsInOrderBy()
- getMaxColumnsInSelect()
- getMaxColumnsInTable()
- getMaxConnections()
- getMaxCursorNameLength()
- getMaxIndexLength()
- getMaxProcedureNameLength()
- getMaxRowSize()
- getMaxSchemaNameLength()
- getMaxStatementLength()
- getMaxStatements()
- getMaxTableNameLength()
- getMaxTablesInSelect()
- getMaxUserNameLength()
- getNumericFunctions()
- getPrimaryKeys()
- getProcedures()
- getProcedureTerm()
- getSchemas()
- getSchemaTerm()
- getSearchStringEscape()
- getSQLKeywords()
- getStringFunctions()
- getSystemFunctions()
- getTables()
- getTableTypes()
- getTimeDateFunctions()
- getTypeInfo()
- getURL()
- getUserName()
- isCatalogAtStart()
- isReadOnly()
- nullPlusNonNullIsNull()
- nullsAreSortedAtEnd()
- nullsAreSortedAtStart()
- nullsAreSortedHigh()
- nullsAreSortedLow()
- storesLowerCaseIdentifiers()
- storesLowerCaseQuotedIdentifiers()
- storesMixedCaseIdentifiers()
- storesMixedCaseQuotedIdentifiers()
- storesUpperCaseIdentifiers()
- storesUpperCaseQuotedIdentifiers()
- supportsAlterTableWithAddColumn()
- supportsAlterTableWithDropColumn()
- supportsANSI92EntryLevelSQL()
- supportsANSI92FullSQL()
- supportsANSI92IntermediateSQL()
- supportsCatalogsInDataManipulation()
- supportsCatalogsInIndexDefinitions()
- supportsCatalogsInPrivilegeDefinitions()
- supportsCatalogsInProcedureCalls()
- supportsCatalogsInTableDefinitions()
- supportsColumnAliasing()
- supportsConvert()
- supportsCoreSQLGrammar()
- supportsCorrelatedSubqueries()
- supportsDataDefinitionAndDataManipulationTransactions()
- supportsDataManipulationTransactionsOnly()
- supportsDifferentTableCorrelationNames()
- supportsExpressionsInOrderBy()
- supportsExtendedSQLGrammar()
- supportsFullOuterJoins()
- supportsGroupBy()
- supportsGroupByBeyondSelect()
- supportsGroupByUnrelated()
- supportsIntegrityEnhancementFacility()
- supportsLikeEscapeClause()
- supportsLimitedOuterJoins()
- supportsMinimumSQLGrammar()
- supportsMixedCaseIdentifiers()
- supportsMixedCaseQuotedIdentifiers()
- supportsMultipleResultSets()
- supportsMultipleTransactions()
- supportsNonNullableColumns()
- supportsOpenCursorsAcrossCommit()
- supportsOpenCursorsAcrossRollback()
- supportsOpenStatementsAcrossCommit()
- supportsOpenStatementsAcrossRollback()
- supportsOrderByUnrelated()
- supportsOuterJoins()
- supportsPositionedDelete()
- supportsPositionedUpdate()
- supportsSchemasInDataManipulation()
- supportsSchemasInIndexDefinitions()
- supportsSchemasInPrivilegeDefinitions()
- supportsSchemasInProcedureCalls()
- supportsSchemasInTableDefinitions()
- supportsSelectForUpdate()
- supportsStoredProcedures()
- supportsSubqueriesInComparisons()
- supportsSubqueriesInExists()
- supportsSubqueriesInIns()
- supportsSubqueriesInQuantifieds()
- supportsTableCorrelationNames()
- supportsTransactionIsolationLevel()
- supportsTransactions()
- supportsUnion()
- supportsUnionAll()
- usesLocalFilePerTable()
- usesLocalFiles()
- Support for the following methods is implemented and under testing:
- getBestRowIdentifier()
- getColumnPrivileges()
- getTablePrivileges()
- Support for the following methods is planned:
- getIndexInfo()
- supportsConvert()
- These methods will not be supported:
- getCatalogs()
- getCrossReference()
- getProcedureColumns()
- getVersionColumns()
IV. Change history
- Release 3.1.7 7/12/1999
-
Fixed a bug that was returning the years 2001-2009 as 201-209 when
using the DATETIME datatype. We recommend that all customers upgrade
to version 3.1.7 of the Informix driver to avoid this problem.
-
When using Prepared Statements in a connection pool the driver was
failing to close PreparedStatements under certain conditions. The
driver now closes the statements correctly, preventing memory leaks.
-
When attempting to read unsupported Informix version 9 datatypes,
the driver will now throw a SQLException instead of an
ArrayOutOfBounds exception.
-
Accessing data from a remote server was causing a "Sqlhosts file not
found or cannot be opened" error. You can now set a property called
INFORMIXSQLHOSTS or weblogic.informix4.informixsqlhosts
that specifies a full path to your sqlhosts file. For example:
weblogic.informix4.informixsqlhosts=\usr\informix\etc\sqlhosts
-
You can now set a property which will log SQL commands. You must first
specify an output PrintStream with DriverManager.setLogStream() and then set the property
weblogic.informix4.SQLTrace=Y.
- Release 3.1.6 -- 2/23/1999
-
Improved support for Unicode and multibyte character sets. Database character sets may be set as a property so that strings received and transmitted by clients are automatically translated using the proper encoding.
- Release 3.1.5 -- 2/4/1999
- Maintenance refresh of the distribution.
- Release 3.1.4 -- 1/25/1999
-
CallableStatements can now have input parameters of BYTE and TEXT data
types. This feature was not previously implemented in
jdbcKona/Informix4.
-
Informix Dynamic Server can throw an exception during ResultSet
processing. The driver now anticipates this and throws a SQLException
when it happens.
-
Added class weblogic.jdbc.common4.sql.Values, which was
omitted from the distribution .zip file.
- Release 3.1.3 -- 12/1/1998
-
PrepareStatement.setString()
no longer produces a syntax error (-210) if you set a parameter to the
empty string ("").
- Release 3.1.2 -- 11/4/98
-
PreparedStatement.setString() now accepts strings
longer than 256 characters, if the property weblogic.informix4.escape_quotes_in_setString is not set
or is set to "Y". If this property is set to "N", then backwards
compatibility forces old behavior, including the 256-character length
restriction.
-
ResultSet.getBigDecimal() now
returns a Java null value if the result is a SQL NULL value. Previously, it returned
0.
-
SQLExceptions encountered during TEXT/BYTE retrieval are now returned as regular
SQLExceptions.
-
DatabaseMetaData.supportsTransactions() now accurately
reports the transaction isolation levels supported by the database
the driver has logged into.
-
PreparedStatement.setObject()
now does type conversion if a compatible target JDBC type is specified.
-
- Release 3.1.1 -- 10/5/98
-
Added a sequence to positively close cursors on the Informix server after
a SELECT, reducing memory use on the server.
-
If there is no response from a login attempt, the driver returns an
SQLException. The default wait time is 90 seconds. The value can be
modified by setting the connection property weblogic.informix4.login_timeout_secs=seconds_to_wait.
-
Fixed a bug where,
after a failed login attempt due to a bad password,
subsequent login attempts on some Informix server versions failed.
- Release 3.1.0 -- 9/15/98
-
This release supports the INT8 and SERIAL8 data types available with
Informix Universal Data Option. The INT8 data type maps to java.sql.types.BigInt
and is retrieved with ResultSet.getLong().
the SERIAL8 has the same data format as the INT8 and can be inserted and queried
like other data types. Unlike the (4-byte) SERIAL type, the driver does not immediately have
access to the value of a SERIAL8 data type after an insert; you must SELECT the column after
an INSERT to find its value.
-
PreparedStatement.setString()
now automatically escapes single quotes sent to the server.
Although it is not recommended, you can revert to the old method (no automatic escapes for
single quotes) by setting the following property in the connection URL:
weblogic.informix4.escape_quotes_in_setString=N
-
Formatting of FLOAT and DECIMAL column data is fixed.
-
Processing aggregates of FLOAT and DECIMAL columns is cleaned up.
- Implementedweblogic.informix4.db_date property for Y4 style date formats. Like the
Informix DBDATE environment variable, the weblogic.informix4.db_date property controls formatting
of input dates in Strings. It does not affect the
storage format. The driver allows only "Y4" formats. Attempting to
use a format containing "Y2" causes an exception: "Driver only
supports Y4 style date dates".
-
Fixed an error when PreparedStatement.setObject()
or PreparedStatement.setString() were used with
null objects.
-
PreparedStatement.setTimestamp() now works for all
definable DATETIME columns.
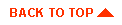